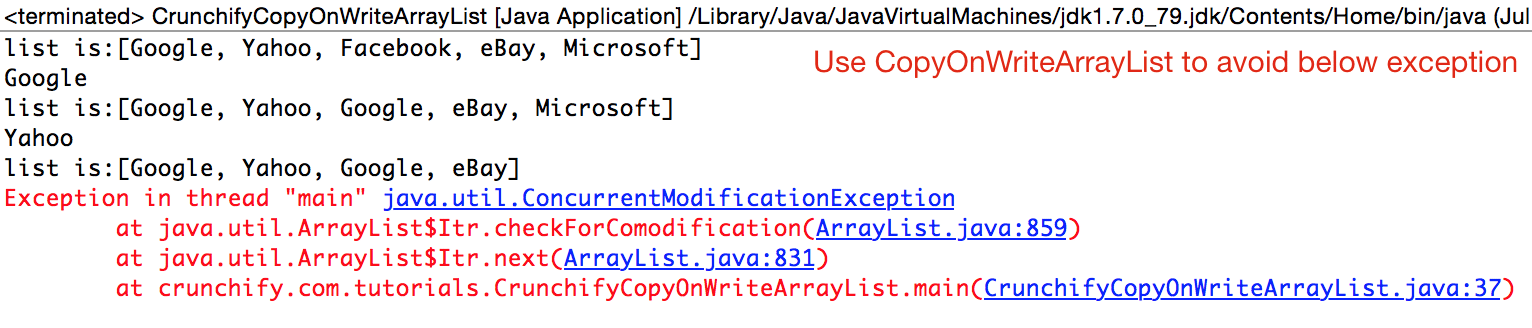
java.util.List
is an ordered collection also known as a sequence
. ArrayList is a very basic implementation of List
. There are number of articles I’ve posted on Crunchify before on ArrayList list convert HashMap to ArrayList, Find unique values from ArrayList, etc.
In this tutorial we will go over why we could use CopyOnWriteArrayList
to avoid java.util.ConcurrentModificationException
.
Let’s get started:
- Create class CrunchifyCopyOnWriteArrayList.java
- Create a List
companies
- Add 5 values to
companies
and iterate through List - While iterating modify (add/remove) elements from List
- You will see ConcurrentModificationException because its internal
modCount
was what tipped off the iterator - Now Just comment
line 18
and uncommentline 21
and re-run program.
package crunchify.com.tutorials; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import java.util.concurrent.CopyOnWriteArrayList; /** * @author Crunchify.com * */ public class CrunchifyCopyOnWriteArrayList { public static void main(String[] args) { // Disable this to avoid ConcurrentModificationException List<String> companies = new ArrayList<>(); // Enable this to avoid ConcurrentModificationException // List<String> companies = new CopyOnWriteArrayList<>(); companies.add("Google"); companies.add("Yahoo"); companies.add("Facebook"); companies.add("eBay"); companies.add("Microsoft"); // Get an iterator over a collection. Iterator takes the place of // Enumeration in the Java Collections Framework. Iterator<String> crunchifyIterator = companies.iterator(); // Make changes to companies List while performing hasNext() while (crunchifyIterator.hasNext()) { System.out.println("companies list: " + companies); String crunchifyString = crunchifyIterator.next(); // Test1: Below statement causes ConcurrentModificationException System.out.println(crunchifyString); if (crunchifyString.equals("Yahoo")) // modCount = 6 companies.remove("Microsoft"); if (crunchifyString.equals("eBay")) companies.add("My Message Goes here... eBay present"); // Test2: Below change wont throw ConcurrentModificationException // Reason: It doesn't change modCount variable of "companies" if (crunchifyString.equals("Google")) companies.set(2, "Google"); } } }
Why java.util.ConcurrentModificationException:
Point-1)
After adding all 5 companies to list you will see modCount = 5
, size = 5
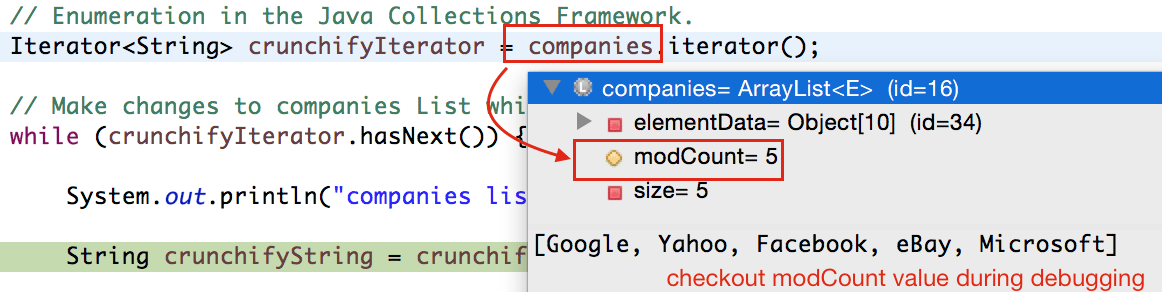
Point-2)
After removing element Microsoft
you will see modCount = 6
, size = 4
which causes an exception.
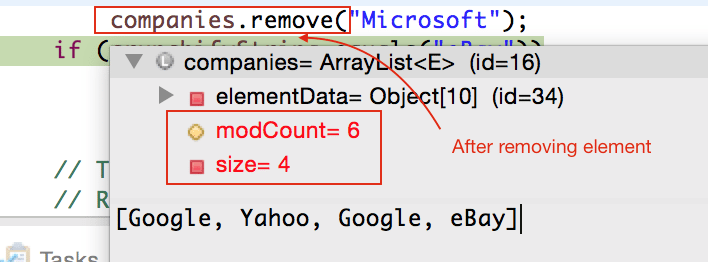
How to solve java.util.ConcurrentModificationException
Just use CopyOnWriteArrayList
instead of ArrayList
in above program. During debug you will see it will add ReentrantLock
on object.
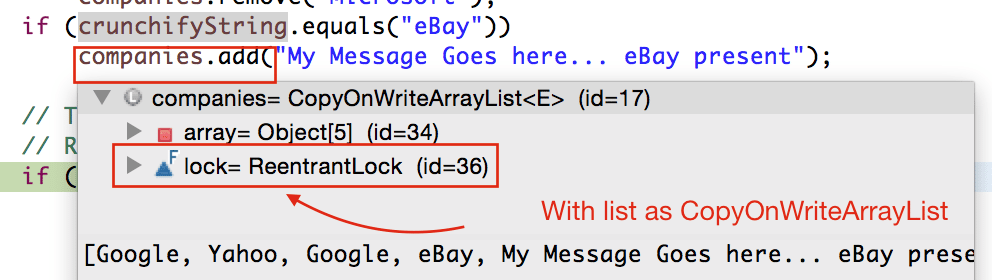
Let me know if you face any issue running this program.
The post When Should I use CopyOnWriteArrayList Vs. ArrayList in Java? Avoid java.util.ConcurrentModification Exception appeared first on Crunchify.
0 Commentaires