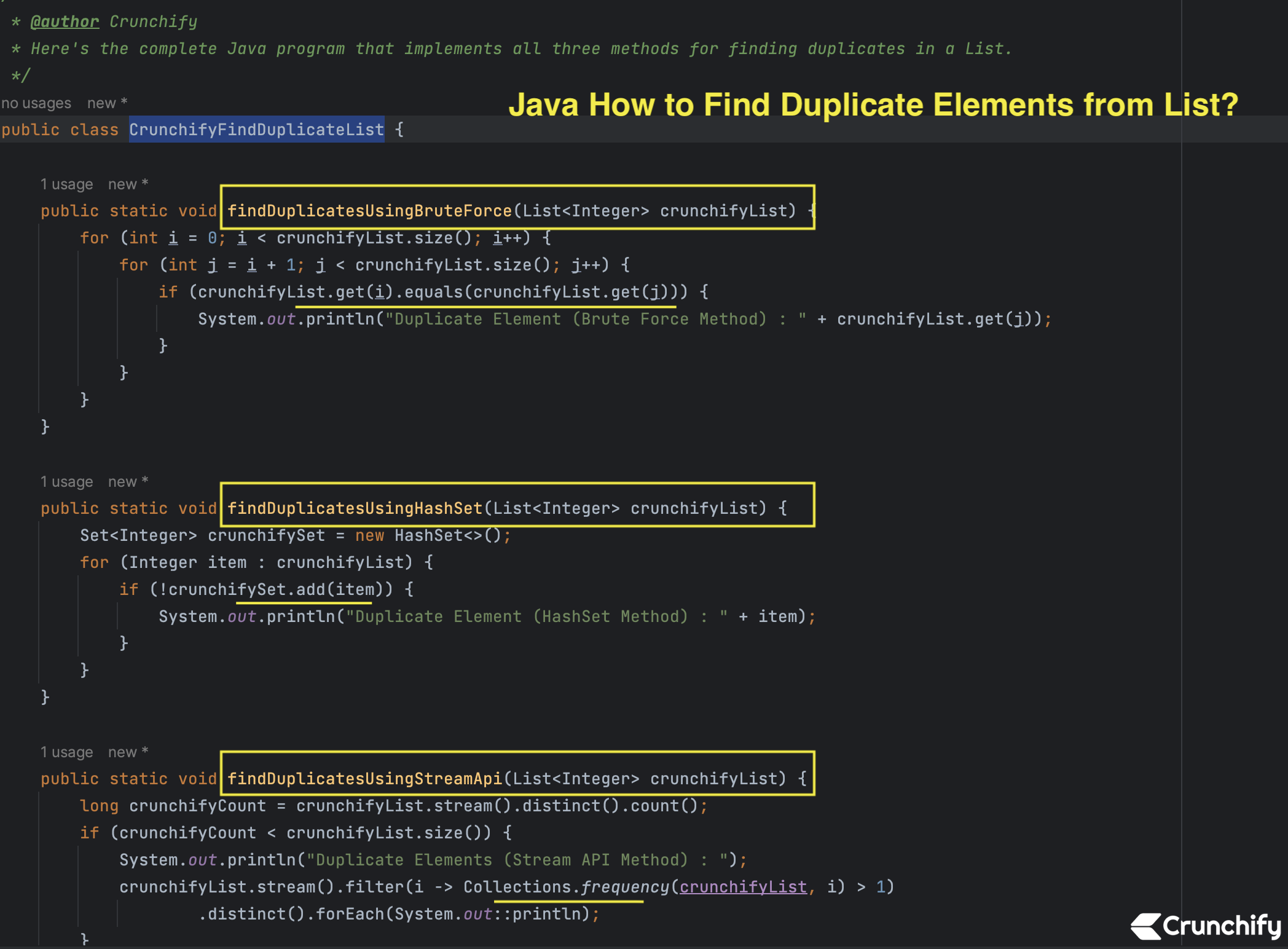
Do you want to identify duplicates elements from Java List?
Finding Duplicate Elements in a Java List. A List is a collection of elements that can contain duplicates. In some cases, it’s necessary to identify duplicates in a List, and there are various methods to achieve this. In this blog post, we’ll discuss three methods for finding duplicates in a Java List: Brute Force, HashSet, and Stream API.
Brute Force Method
The brute force method is the simplest method to find duplicates in a List. It involves looping through each element of the List and comparing it with other elements to check if there are any duplicates. Here’s an example implementation:
import java.util.List; public class FindDuplicates { public static void findDuplicatesUsingBruteForce(List<Integer> list) { for (int i = 0; i < list.size(); i++) { for (int j = i + 1; j < list.size(); j++) { if (list.get(i).equals(list.get(j))) { System.out.println("Duplicate Element : " + list.get(j)); } } } } }
HashSet Method
Another method to find duplicates in a List is to use a HashSet. A HashSet doesn’t allow duplicate elements, so you can loop through the List and add each element to the HashSet. If an element is already present in the HashSet, it means it’s a duplicate. Here’s an example implementation:
import java.util.HashSet; import java.util.List; import java.util.Set; public class FindDuplicates { public static void findDuplicatesUsingHashSet(List<Integer> list) { Set<Integer> set = new HashSet<>(); for (Integer item : list) { if (!set.add(item)) { System.out.println("Duplicate Element : " + item); } } } }
Stream API Method
Java 8 introduced the Stream API, which provides a more concise way of finding duplicates in a List. You can use the distinct()
method to remove duplicates and compare the size of the original List with the size of the List after removing duplicates. If the sizes are different, it means there were duplicates. Here’s an example implementation:
import java.util.Collections; import java.util.List; public class FindDuplicates { public static void findDuplicatesUsingStreamApi(List<Integer> list) { long count = list.stream().distinct().count(); if (count < list.size()) { System.out.println("Duplicate Elements : "); list.stream().filter(i -> Collections.frequency(list, i) > 1) .distinct().forEach(System.out::println); } } }
Here’s the complete Java program that implements all three methods for finding duplicates in a List.
CrunchifyFindDuplicateList.java
package crunchify.com.java.tutorials; import java.util.Collections; import java.util.HashSet; import java.util.List; import java.util.Set; /** * @author Crunchify * Here's the complete Java program that implements all three methods for finding duplicates in a List. */ public class CrunchifyFindDuplicateList { public static void findDuplicatesUsingBruteForce(List<Integer> crunchifyList) { for (int i = 0; i < crunchifyList.size(); i++) { for (int j = i + 1; j < crunchifyList.size(); j++) { if (crunchifyList.get(i).equals(crunchifyList.get(j))) { System.out.println("Duplicate Element (Brute Force Method) : " + crunchifyList.get(j)); } } } } public static void findDuplicatesUsingHashSet(List<Integer> crunchifyList) { Set<Integer> crunchifySet = new HashSet<>(); for (Integer item : crunchifyList) { if (!crunchifySet.add(item)) { System.out.println("Duplicate Element (HashSet Method) : " + item); } } } public static void findDuplicatesUsingStreamApi(List<Integer> crunchifyList) { long crunchifyCount = crunchifyList.stream().distinct().count(); if (crunchifyCount < crunchifyList.size()) { System.out.println("Duplicate Elements (Stream API Method) : "); crunchifyList.stream().filter(i -> Collections.frequency(crunchifyList, i) > 1) .distinct().forEach(System.out::println); } } public static void main(String[] args) { // An ordered collection (also known as a sequence). // The user of this interface has precise control over where in the list each element is inserted. // The user can access elements by their integer index (position in the list), and search for elements in the list. List<Integer> crunchifyList = List.of(1, 2, 3, 4, 5, 1, 3, 9, 33, 12, 9, 4); findDuplicatesUsingBruteForce(crunchifyList); findDuplicatesUsingHashSet(crunchifyList); findDuplicatesUsingStreamApi(crunchifyList); } }
The method add
of set
returns a boolean whether a value already exists (true if it does not exist, false if it already exists, see Set documentation).
Output:
Just run above program as Java Application and you will see result as below.
Duplicate Element (Brute Force Method) : 1 Duplicate Element (Brute Force Method) : 3 Duplicate Element (Brute Force Method) : 4 Duplicate Element (Brute Force Method) : 9 Duplicate Element (HashSet Method) : 1 Duplicate Element (HashSet Method) : 3 Duplicate Element (HashSet Method) : 9 Duplicate Element (HashSet Method) : 4 Duplicate Elements (Stream API Method) : 1 3 4 9 Process finished with exit code 0
Visit More Java Tutorials. More Spring MVC Tutorials. Let us know if you have any issue running this program.
The post In Java How to Find Duplicate Elements from List? (Brute Force, HashSet and Stream API) appeared first on Crunchify.
0 Commentaires