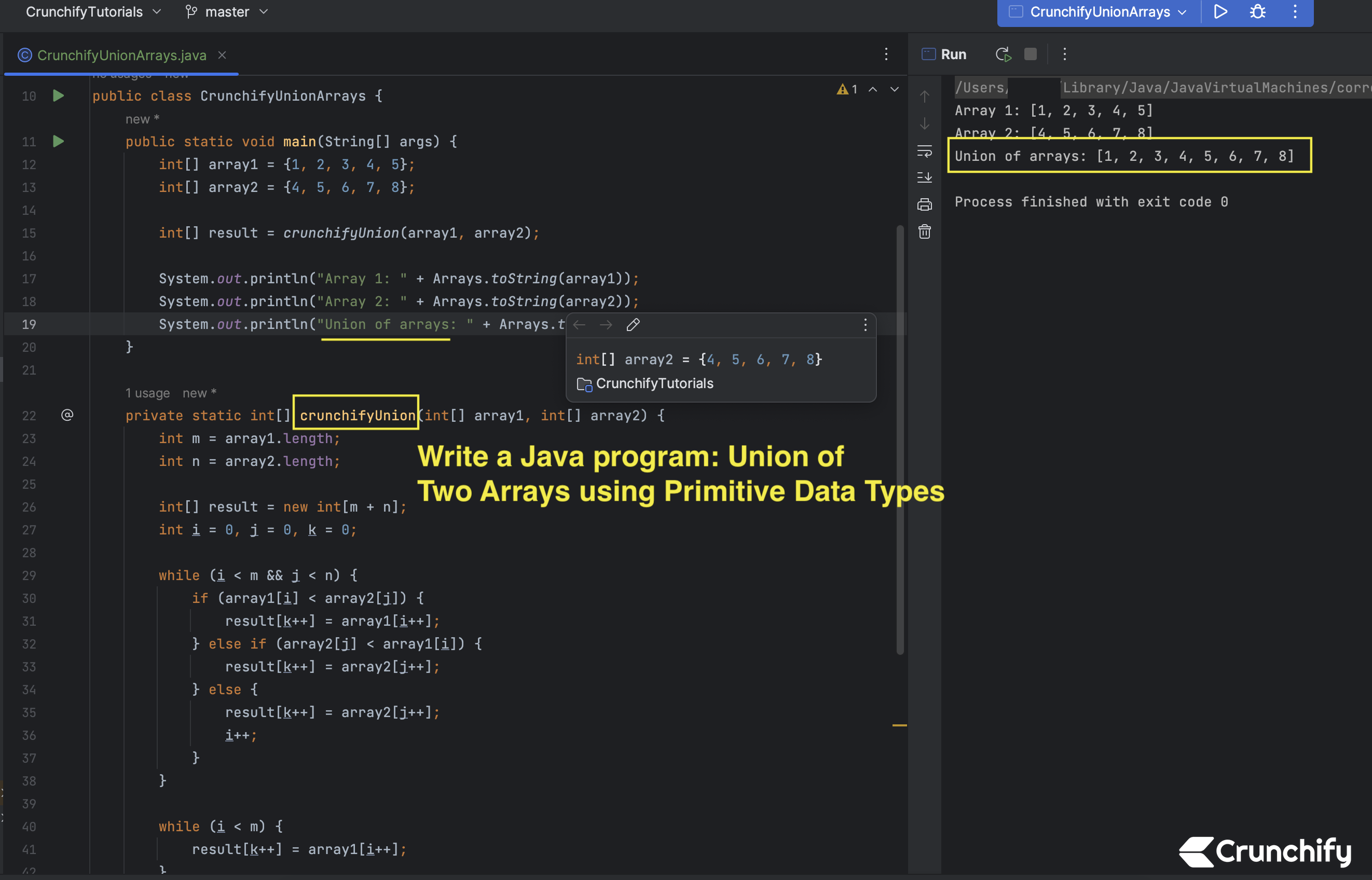
A primitive type is predefined by the Java language and is named by a reserved keyword. Primitive values do not share state with other primitive values.
In Java, primitive data types are basic data types that are built-in to the language.
byte
: A 8-bit signed integer.short
: A 16-bit signed integer.int
: A 32-bit signed integer.long
: A 64-bit signed integer.float
: A 32-bit floating-point number.double
: A 64-bit floating-point number.char
: A 16-bit Unicode character.boolean
: A value that represents eithertrue
orfalse
.
Below Java Code shows you how to do Union in Java without using Java Collection Class & with Primitive Data Types.
Method-1
CrunchifyUnionArrays.java
package crunchify.com.java.tutorials; import java.util.Arrays; /** * @author Crunchify * Write a Java program: Union of Two Arrays using Primitive Data Types */ public class CrunchifyUnionArrays { public static void main(String[] args) { int[] array1 = {1, 2, 3, 4, 5}; int[] array2 = {4, 5, 6, 7, 8}; int[] result = crunchifyUnion(array1, array2); System.out.println("Array 1: " + Arrays.toString(array1)); System.out.println("Array 2: " + Arrays.toString(array2)); System.out.println("Union of arrays: " + Arrays.toString(result)); } private static int[] crunchifyUnion(int[] array1, int[] array2) { int m = array1.length; int n = array2.length; int[] result = new int[m + n]; int i = 0, j = 0, k = 0; while (i < m && j < n) { if (array1[i] < array2[j]) { result[k++] = array1[i++]; } else if (array2[j] < array1[i]) { result[k++] = array2[j++]; } else { result[k++] = array2[j++]; i++; } } while (i < m) { result[k++] = array1[i++]; } while (j < n) { result[k++] = array2[j++]; } int[] finalResult = Arrays.copyOf(result, k); return finalResult; } }
Run above program as Java Application.
Array 1: [1, 2, 3, 4, 5] Array 2: [4, 5, 6, 7, 8] Union of arrays: [1, 2, 3, 4, 5, 6, 7, 8] Process finished with exit code 0
The program implements the union of two arrays using primitive data types in Java. The program starts by declaring a UnionArray
class and a main
method within it, which serves as the starting point of the program.
Within the main
method, two arrays array1
and array2
are created and initialized with some integer values. The program then calls the union
method and passes the two arrays as arguments, which returns a new array that contains the union of the two input arrays.
The result array is then printed to the console using the Arrays.toString
method, which converts the array to a string representation.
The union
method implements the algorithm for the union of two arrays. The method takes two arrays as input and uses two pointers, one for each array, to merge the elements of the arrays into a single output array.
The result array contains the union of the two input arrays, with no duplicates. The method uses a while loop to iterate through both arrays, comparing the elements of each array and adding the smaller element to the result array.
If the elements are equal, the element from the second array is added to the result array. The method uses additional while loops to handle the remaining elements in the arrays if one array has been fully processed before the other. The result array is then returned as the output of the method.
Method-2
Java Code: CrunchifyUnionTwoArrays.java
package com.crunchify.tutorials; import java.util.Arrays; /** * @author Crunchify.com */ public class CrunchifyUnionTwoArrays { public static void main(String[] args) { int[] A = { 4, 11, 2, 1, 3, 3, 5, 7}; int[] B = { 5, 2, 3, 15, 1, 0, 9 }; System.out.println("Integer Array A: " + Arrays.toString(A)); System.out.println("Integer Array B: " + Arrays.toString(B)); System.out.println("\nUnion: " + Arrays.toString(findUnion(A, B))); } /* Union of multiple arrays */ public static int[] findUnion(int[]... arrays) { int maxSize = 0; int counter = 0; for (int[] array : arrays) maxSize += array.length; int[] temp = new int[maxSize]; for (int[] array : arrays) for (int i : array) if (!findDuplicated(temp, counter, i)) temp[counter++] = i; int[] result = new int[counter]; for (int i = 0; i < counter; i++) result[i] = temp[i]; return result; } public static boolean findDuplicated(int[] array, int counter, int value) { for (int i = 0; i < counter; i++) if (array[i] == value) return true; return false; } }
Result:
Integer Array A: [4, 11, 2, 1, 3, 3, 5, 7] Integer Array B: [5, 2, 3, 15, 1, 0, 9] Union: [4, 11, 2, 1, 3, 5, 7, 15, 0, 9]
Hope you find this useful. In next post will post Union of Two Arrays with the use of Java Collection Class.
The post How to Union Two Arrays in Java using Primitive Data Types? Without Java Collection appeared first on Crunchify.
0 Commentaires