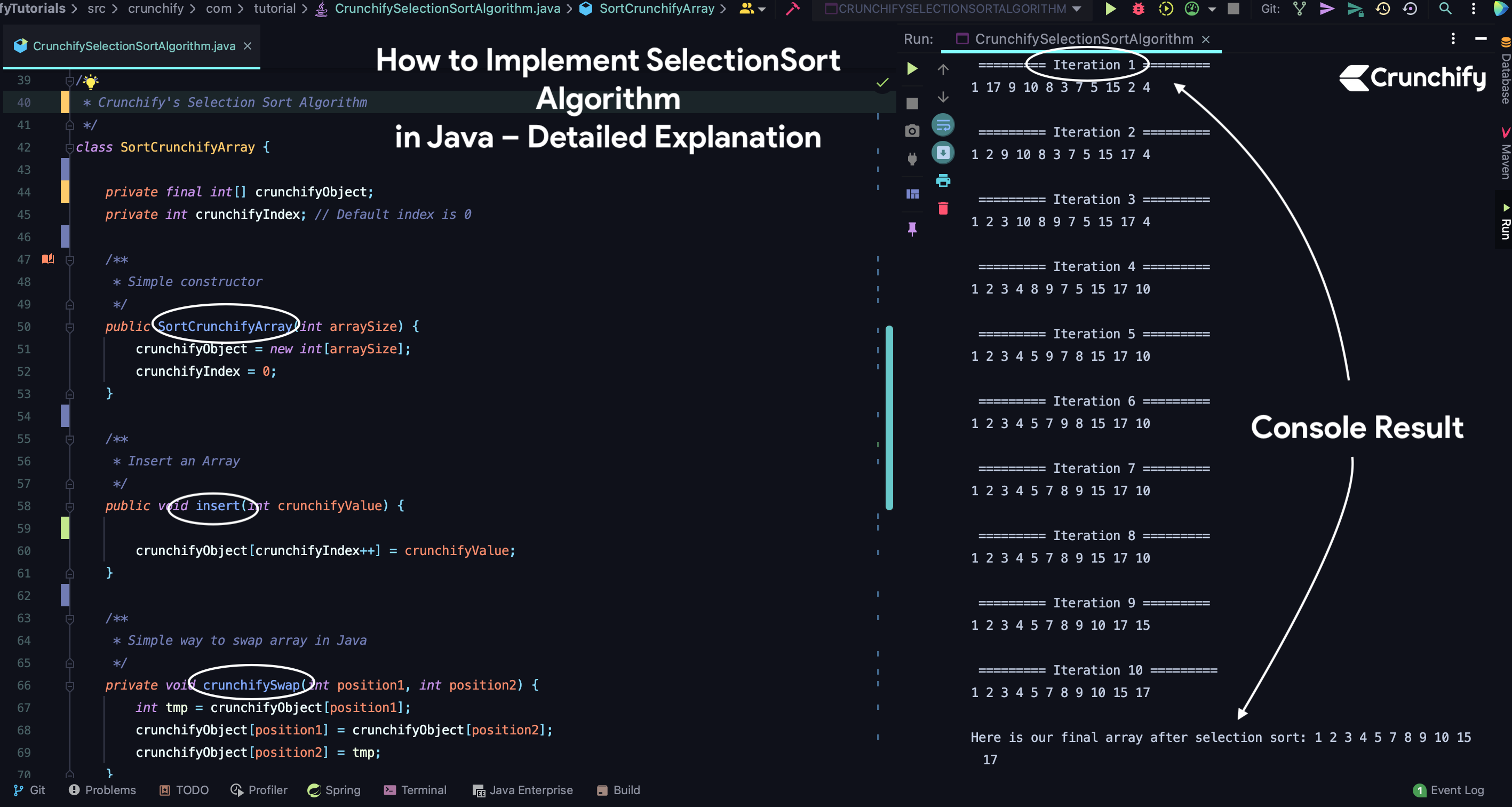
Are you a Computer Science Graduate? Are you preparing for an Interview? Want to become a master in Data Structure?
Well, Sorting algorithms are the most frequently asked questions in Java interview questions. Sometime back we have written an article on Bubble sort ascending and descending order. I remember learning Data Structures and Algorithms Selection Sort in my BE Computer Science degree course. Let’s implement the same today here.
If you have any of below questions then you are at right place:
- Implement selection sort in java
- What is a selection sort in Java? – Simple Search and Sort
- Java Program to Implement Selection Sort
- selection sort program in java with output
In this tutorial we will go over the best way to implement Selection Sort Algorithm in Java
. After each iteration we will print result for you to get a better idea.
Let’s get started
Here are the steps which we are going to perform:
- Create an array
crunchifyArray
withsize 11
- Add total 11 elements to array in random order
- Print initial array using for loop
- Sort an array using
crunchifySelectionSort()
- After each iteration print half sorted array
- Once all elements in an array will be sorted, quit loop
- Print final array using for loop
Logic:
Selection sort logic is very simple.
Sort the shortest element with the element with the 0th position
. Keep doing the same until you reach at the end.
Here is a simple Java Program to Implement Selection Sort – Algorithm Implementation/Sorting/Selection sort.
package crunchify.com.tutorial; /** * @author Crunchify.com * Selection Sort Algorithm in Java */ public class CrunchifySelectionSortAlgorithm { public static void main(String[] args) { // Let's start with arraySize 11 int arraySize = 11; SortCrunchifyArray crunchifyArray; crunchifyArray = new SortCrunchifyArray(arraySize); crunchifyArray.insert(10); crunchifyArray.insert(17); crunchifyArray.insert(9); crunchifyArray.insert(1); crunchifyArray.insert(8); crunchifyArray.insert(3); crunchifyArray.insert(7); crunchifyArray.insert(5); crunchifyArray.insert(15); crunchifyArray.insert(2); crunchifyArray.insert(4); System.out.print("Here is our initial array: "); crunchifyArray.crunchifyLog(); crunchifyArray.crunchifySelectionSort(); System.out.print("Here is our final array after selection sort: "); crunchifyArray.crunchifyLog(); } } /** * Crunchify's Selection Sort Algorithm */ class SortCrunchifyArray { private final int[] crunchifyObject; private int crunchifyIndex; // Default index is 0 /** * Simple constructor */ public SortCrunchifyArray(int arraySize) { crunchifyObject = new int[arraySize]; crunchifyIndex = 0; } /** * Insert an Array */ public void insert(int crunchifyValue) { crunchifyObject[crunchifyIndex++] = crunchifyValue; } /** * Simple way to swap array in Java */ private void crunchifySwap(int position1, int position2) { int tmp = crunchifyObject[position1]; crunchifyObject[position1] = crunchifyObject[position2]; crunchifyObject[position2] = tmp; } /** * Actual Selection Sort Logic goes here... */ public void crunchifySelectionSort() { int outerObj, innerObj, minObj; // Counter to print iteration int counter = 1; for (outerObj = 0; outerObj < crunchifyIndex - 1; outerObj++) { minObj = outerObj; for (innerObj = outerObj + 1; innerObj < crunchifyIndex; innerObj++) if (crunchifyObject[innerObj] < crunchifyObject[minObj]) { minObj = innerObj; } crunchifySwap(outerObj, minObj); // swap an object // Let's print array after each iteration log("Iteration " + counter); counter++; } } private void log(String string) { System.out.println(" ========= " + string + " ========= "); crunchifyLog(); } /** * Let's display Array */ public void crunchifyLog() { for (int i = 0; i < crunchifyIndex; i++) System.out.print(crunchifyObject[i] + " "); System.out.println("\n"); } }
Let me know if you have any question on implementation of selection sort in java.
Eclipse Console Output:
Here is our initial array: 10 17 9 1 8 3 7 5 15 2 4 ========= Iteration 1 ========= 1 17 9 10 8 3 7 5 15 2 4 ========= Iteration 2 ========= 1 2 9 10 8 3 7 5 15 17 4 ========= Iteration 3 ========= 1 2 3 10 8 9 7 5 15 17 4 ========= Iteration 4 ========= 1 2 3 4 8 9 7 5 15 17 10 ========= Iteration 5 ========= 1 2 3 4 5 9 7 8 15 17 10 ========= Iteration 6 ========= 1 2 3 4 5 7 9 8 15 17 10 ========= Iteration 7 ========= 1 2 3 4 5 7 8 9 15 17 10 ========= Iteration 8 ========= 1 2 3 4 5 7 8 9 15 17 10 ========= Iteration 9 ========= 1 2 3 4 5 7 8 9 10 17 15 ========= Iteration 10 ========= 1 2 3 4 5 7 8 9 10 15 17 Here is our final array after selection sort: 1 2 3 4 5 7 8 9 10 15 17 Process finished with exit code 0
Now the next questions would be what is a time complexity for above program?
Well, the time complexity is O(n2)
.
The post How to Implement Selection Sort Algorithm in Java – Detailed Explanation appeared first on Crunchify.
0 Commentaires