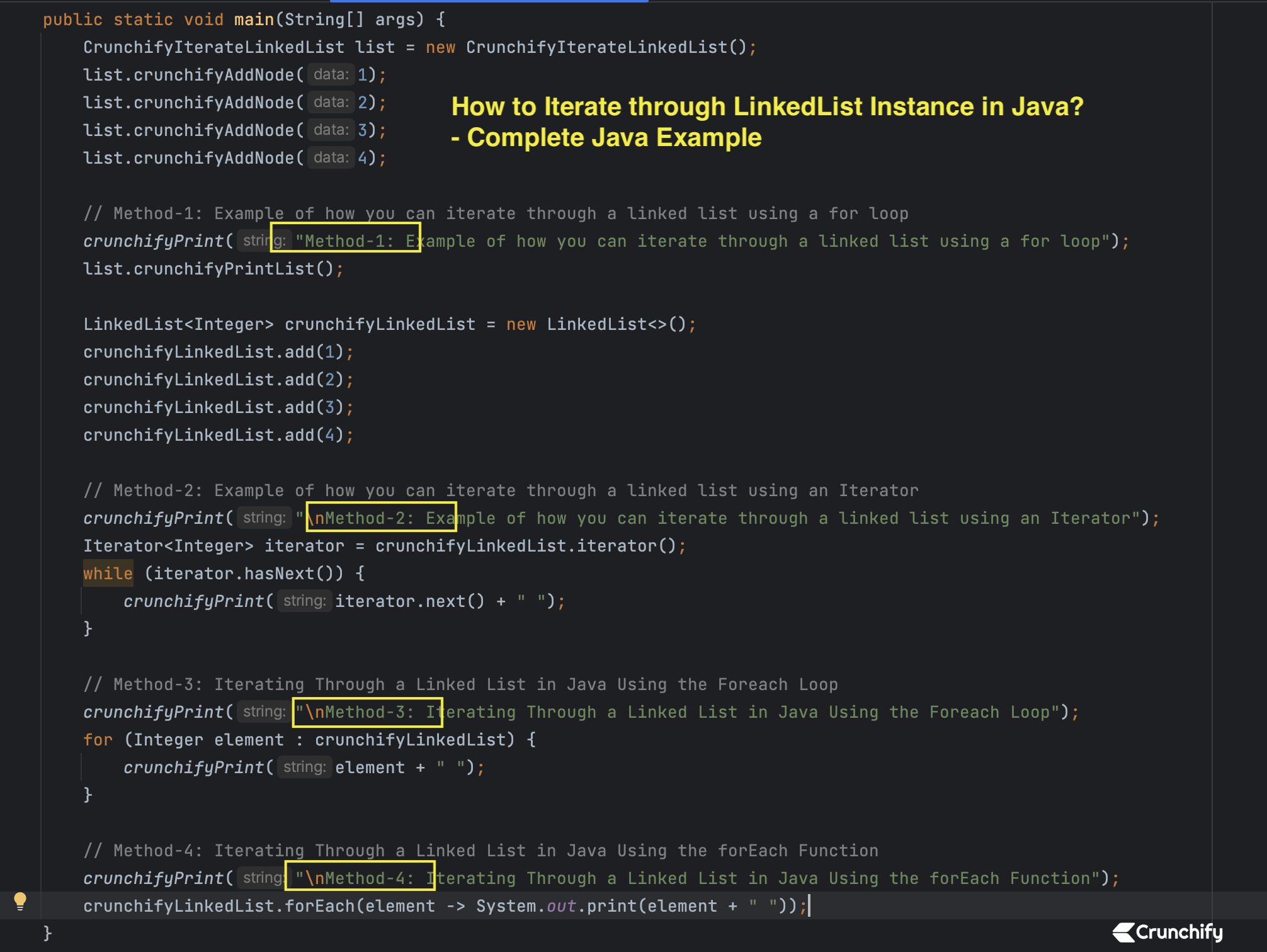
A linked list is a data structure that consists of a sequence of nodes, where each node stores an element and a reference to the next node. In Java, the LinkedList
class implements the Iterable
interface, which provides several ways to iterate through its elements. In this article, we will discuss three common methods to iterate through a linked list: using a for loop, foreach loop, and forEach function.
Example-1. Integer LinkedList
package crunchify.com.tutorials; import java.util.Iterator; import java.util.LinkedList; /** * @author Crunchify.com * Iterating Through a Linked List in Java: Using for Loop, Foreach Loop, and forEach Function */ public class CrunchifyIterateLinkedList { private crunchifyNode head; static class crunchifyNode { int data; crunchifyNode next; crunchifyNode(int d) { data = d; next = null; } } public void crunchifyAddNode(int data) { crunchifyNode newNode = new crunchifyNode(data); newNode.next = head; head = newNode; } public void crunchifyPrintList() { crunchifyNode current = head; while (current != null) { crunchifyPrint(current.data + " "); current = current.next; } } private static void crunchifyPrint(Object string) { System.out.println(string); } public static void main(String[] args) { CrunchifyIterateLinkedList list = new CrunchifyIterateLinkedList(); list.crunchifyAddNode(1); list.crunchifyAddNode(2); list.crunchifyAddNode(3); list.crunchifyAddNode(4); // Method-1: Example of how you can iterate through a linked list using a for loop crunchifyPrint("Method-1: Example of how you can iterate through a linked list using a for loop"); list.crunchifyPrintList(); LinkedList<Integer> crunchifyLinkedList = new LinkedList<>(); crunchifyLinkedList.add(1); crunchifyLinkedList.add(2); crunchifyLinkedList.add(3); crunchifyLinkedList.add(4); // Method-2: Example of how you can iterate through a linked list using an Iterator crunchifyPrint("\nMethod-2: Example of how you can iterate through a linked list using an Iterator"); Iterator<Integer> iterator = crunchifyLinkedList.iterator(); while (iterator.hasNext()) { crunchifyPrint(iterator.next() + " "); } // Method-3: Iterating Through a Linked List in Java Using the Foreach Loop crunchifyPrint("\nMethod-3: Iterating Through a Linked List in Java Using the Foreach Loop"); for (Integer element : crunchifyLinkedList) { crunchifyPrint(element + " "); } // Method-4: Iterating Through a Linked List in Java Using the forEach Function crunchifyPrint("\nMethod-4: Iterating Through a Linked List in Java Using the forEach Function"); crunchifyLinkedList.forEach(element -> System.out.print(element + " ")); } }
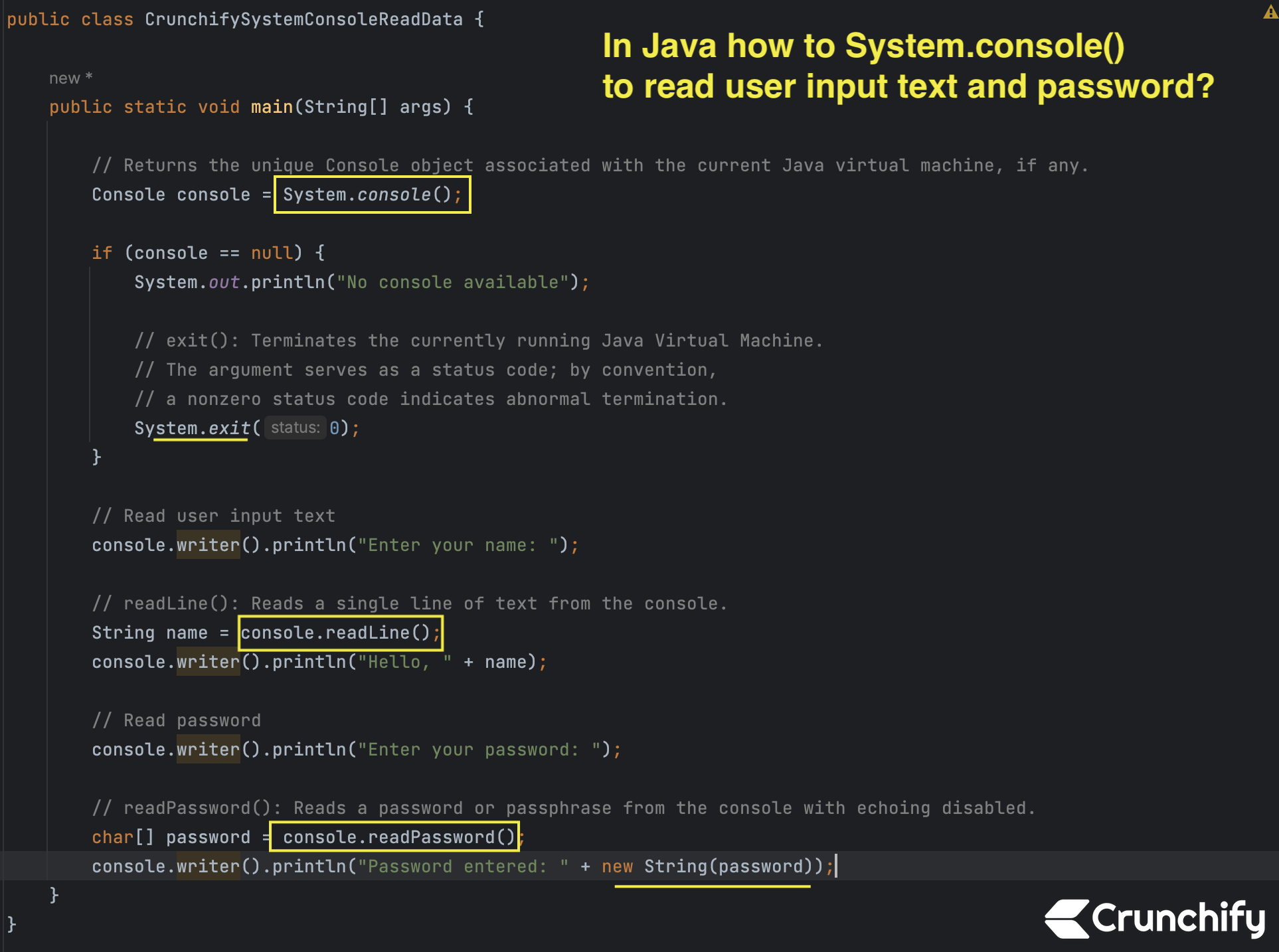
Using a for loop
In this example, we’ve created a LinkedList
of integers and added several elements to it. We then used a for loop to iterate through its elements. The for loop uses the size
method of the linked list to determine the number of iterations, and the get
method to access each element by index.
Using the foreach loop
In this example, we’ve created a LinkedList
of integers and added several elements to it. We then used the foreach loop to iterate through its elements. The foreach loop automatically calls the iterator
method on the linkedList
object to obtain an Iterator, and then repeatedly calls the hasNext
and next
methods on the Iterator until there are no more elements.
Using the forEach function
Performs the given action for each element of the Iterable until all elements have been processed or the action throws an exception. Actions are performed in the order of iteration, if that order is specified. Exceptions thrown by the action are relayed to the caller.
The behavior of this method is unspecified if the action performs side-effects that modify the underlying source of elements, unless an overriding class has specified a concurrent modification policy.
IntelliJ IDEA Console Result:
Method-1: Example of how you can iterate through a linked list using a for loop 4 3 2 1 Method-2: Example of how you can iterate through a linked list using an Iterator 1 2 3 4 Method-3: Iterating Through a Linked List in Java Using the Foreach Loop 1 2 3 4 Method-4: Iterating Through a Linked List in Java Using the forEach Function 1 2 3 4 Process finished with exit code 0
Example-2. String LinkedList
LinkedList implementation of the List interface. Implements all optional list operations, and permits all elements (including null). In addition to implementing the List interface, the LinkedList class provides uniformly named methods to get, remove and insert an element at the beginning and end of the list. These operations allow linked lists to be used as a stack, queue, or double-ended queue.
The class implements the Deque interface, providing first-in-first-out queue operations for add, poll, along with other stack and deque operations.
All of the operations perform as could be expected for a doubly-linked list. Operations that index into the list will traverse the list from the beginning or the end, whichever is closer to the specified index.
Note that this implementation is not synchronized
. If multiple threads access a linked list concurrently, and at least one of the threads modifies the list structurally, it must be synchronized externally.
Lets first instantiate and populate a LinkedList implementation which contains the names of top Bay Area’s Companies.
Java Code:
package crunchify.com.tutorials; import java.util.LinkedList; import java.util.ListIterator; /** * @author Crunchify.com * How to iterate through LinkedList in Java? */ public class CrunchifyLinkedListIterator { public static void main(String[] args) { LinkedList<String> linkedList = new LinkedList<String>(); linkedList.add("Paypal"); linkedList.add("Google"); linkedList.add("Yahoo"); linkedList.add("IBM"); linkedList.add("Facebook"); // ListIterator approach System.out.println("ListIterator Approach: =========="); ListIterator<String> listIterator = linkedList.listIterator(); while (listIterator.hasNext()) { System.out.println(listIterator.next()); } System.out.println("\nLoop Approach: =========="); // Traditional for loop approach for (int i = 0; i < linkedList.size(); i++) { System.out.println(linkedList.get(i)); } // Java8 Loop System.out.println("\nJava8 Approach: =========="); // forEach Performs the given action for each element of the Iterable until all elements have been processed or // the action throws an exception. linkedList.forEach(System.out::println); } }
Output:
ListIterator Approach: Paypal Google Yahoo IBM Facebook Loop Approach: Paypal Google Yahoo IBM Facebook Java8 Approach: Paypal Google Yahoo IBM Facebook
Let me know if you face any issue running this code.
The post How to Iterate through LinkedList Instance in Java? appeared first on Crunchify.
0 Commentaires