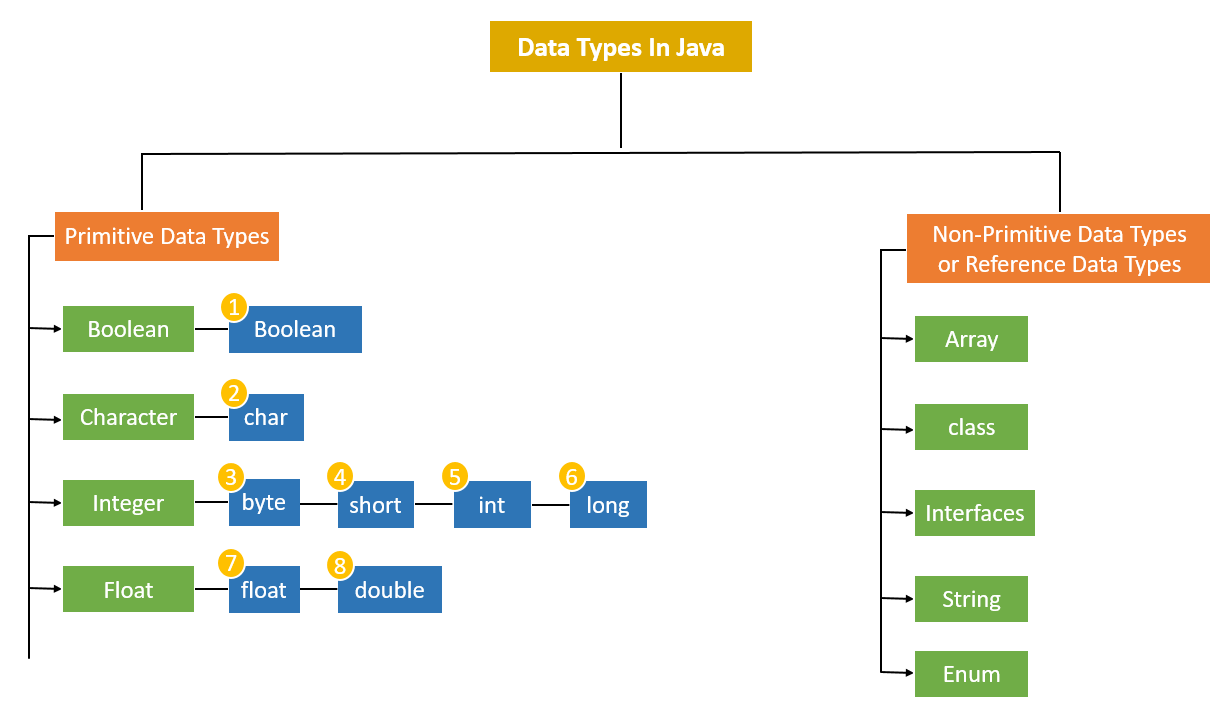
Java is a widely used programming language that is known for its simplicity and versatility. One of the fundamental concepts in Java is the data type, which defines the type of data that can be stored in a variable or used in an expression. Java supports two categories of data types: primitive data types and reference data types. In this blog post, we will focus on Java primitive data types.
Eight primitive data types in Java
- byte
- short
- int
- long
- float
- double
- char
- boolean
Primitive data types in Java are the most basic types of data that are directly supported by the language. There are eight primitive data types in Java, which are classified into four categories: integer, floating-point, character, and boolean.
The eight primitive data types supported by the Java programming language are:
byte
: Thebyte
data type is an 8-bit signed two’s complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive).- The
byte
data type can be useful for saving memory in large arrays, where the memory savings actually matters. - They can also be used in place of
int
where their limits help to clarify your code; the fact that a variable’s range is limited can serve as a form of documentation.
- The
short
: Theshort
data type is a 16-bit signed two’s complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive).- As with
byte
, the same guidelines apply: you can use ashort
to save memory in large arrays, in situations where the memory savings actually matters.
- As with
int
: Theint
data type is a 32-bit signed two’s complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive).- For integral values, this data type is generally the default choice unless there is a reason (like the above) to choose something else.
- This data type will most likely be large enough for the numbers your program will use, but if you need a wider range of values, use
long
instead.
long
: Thelong
data type is a 64-bit signed two’s complement integer.- It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive).
- Use this data type when you need a range of values wider than those provided by
int
.
float
: Thefloat
data type is a single-precision 32-bit IEEE 754 floating point.- Its range of values is beyond the scope of this discussion, but is specified in the Floating-Point Types, Formats, and Values section of the Java Language Specification.
- As with the recommendations for
byte
andshort
, use afloat
(instead ofdouble
) if you need to save memory in large arrays of floating point numbers. This data type should never be used for precise values, such as currency. - For that, you will need to use the java.math.BigDecimal class instead. Numbers and Strings covers
BigDecimal
and other useful classes provided by the Java platform.
double
: Thedouble
data type is a double-precision 64-bit IEEE 754 floating point.- Its range of values is beyond the scope of this discussion, but is specified in the Floating-Point Types, Formats, and Values section of the Java Language Specification.
- For decimal values, this data type is generally the default choice. As mentioned above, this data type should never be used for precise values, such as currency.
boolean
: Theboolean
data type has only two possible values:true
andfalse
.- Use this data type for simple flags that track true/false conditions.
- This data type represents one bit of information, but its “size” isn’t something that’s precisely defined.
char
: Thechar
data type is a single 16-bit Unicode character.- It has a minimum value of
'\u0000'
(or 0) and a maximum value of'\uffff'
(or 65,535 inclusive).
- It has a minimum value of
Here is a table that lists the eight primitive data types in Java,
- Along with their size in bits and their range of values:
Data Type | Size (bits) | Range of Values |
byte | 8 | -128 to 127 |
short | 16 | -32,768 to 32,767 |
int | 32 | -2,147,483,648 to 2,147,483,647 |
long | 64 | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 32 | Approximately 1.4E-45 to 3.4E+38, with a precision of 6-7 decimal digits |
double | 64 | Approximately 4.9E-324 to 1.8E+308, with a precision of 15-16 decimal digits |
char | 16 | Unicode characters from ‘\u0000’ to ‘\uffff’ |
boolean | not specified | true or false |
It is important to note that Java is a strongly-typed language, which means that each variable must have a specific data type. When declaring a variable in Java, you must specify its data type, as well as its name. For example, to declare a variable of type int with the name “x”, you would write:
int x;
You can also initialize the variable at the time of declaration, like this:
int x = 676;
Java also supports type casting, which allows you to convert a value from one data type to another. There are two types of casting in Java: widening and narrowing. Widening casting is done automatically by the compiler when converting a smaller data type to a larger data type.
For example, if you assign an int value to a long variable, the int value will be automatically widened to a long. Narrowing casting, on the other hand, must be done explicitly using a cast operator. For example, if you want to convert a long value to an int, you would write:
long l = 1234567890L; int i = (int) l;
Understanding the different primitive data types in Java is an essential part of learning the language. By mastering these data types, you will be able to write more complex and powerful programs that can handle a wide range of data.
The post Java Primitive Data Types details appeared first on Crunchify.
0 Commentaires