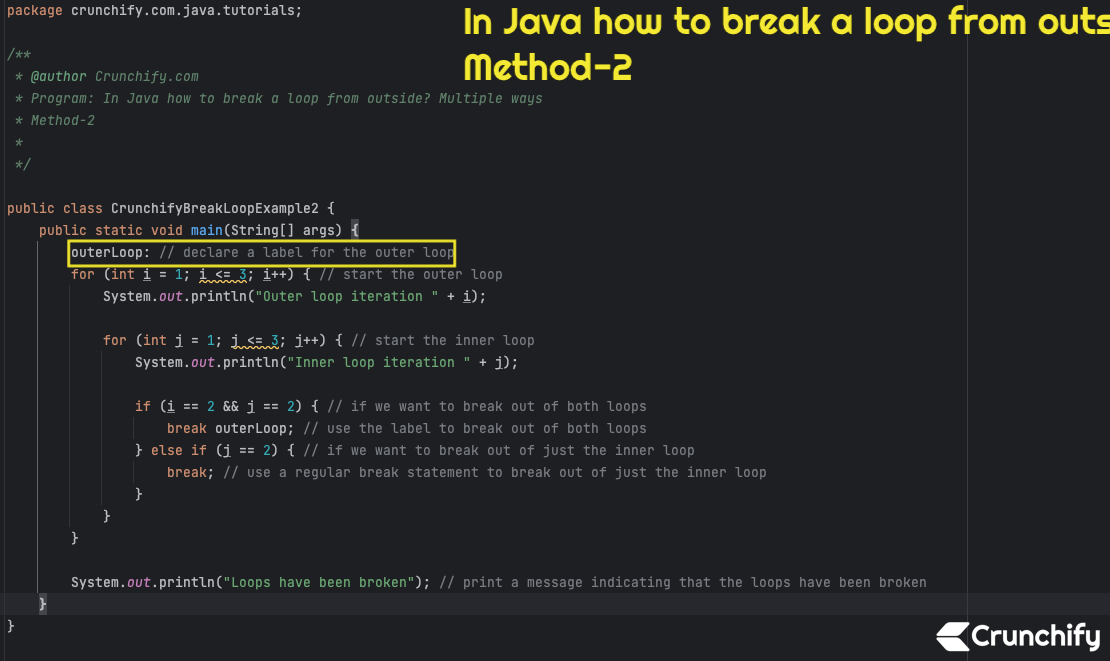
In Java, it is possible to break out of a loop from outside the loop’s function by using a labeled break statement. This can be useful when you need to stop the loop based on a condition outside of the loop, such as a user input or a system event. In this blog post, we will explore how to use labeled break statements in Java and provide code examples.
Labeled Break Statement.
A labeled break statement in Java allows you to specify a label for a loop, which can then be used to break out of the loop from outside of the loop’s function. The syntax for a labeled break statement is as follows:
labelName: for (initialization; condition; increment/decrement) { // loop body if (condition) { break labelName; } }
Example-1
In this syntax, labelName
is any valid Java identifier, and it is followed by a colon. The label is then placed before the loop keyword (for
, while
, or do-while
). Inside the loop body, you can use the break
keyword followed by the label name to break out of the loop.
package crunchify.com.java.tutorials; /** * @author Crunchify.com * Program: In Java how to break a loop from outside? Multiple ways * Method-2 * */ public class CrunchifyBreakLoopExample2 { public static void main(String[] args) { outerLoop: // declare a label for the outer loop for (int i = 1; i <= 3; i++) { // start the outer loop System.out.println("Outer loop iteration " + i); for (int j = 1; j <= 3; j++) { // start the inner loop System.out.println("Inner loop iteration " + j); if (i == 2 && j == 2) { // if we want to break out of both loops break outerLoop; // use the label to break out of both loops } else if (j == 2) { // if we want to break out of just the inner loop break; // use a regular break statement to break out of just the inner loop } } } System.out.println("Loops have been broken"); // print a message indicating that the loops have been broken } }
In this program, we use a label called outerLoop
to mark the outer loop. We start the outer loop with a for
loop that counts from 1 to 3. Inside the outer loop, we start an inner loop with another for
loop that counts from 1 to 3.
Inside the inner loop, we include two if
statements that check if we should break out of the loops. The first if statement checks if we want to break out of both loops (when i
is 2 and j
is 2), and if so, we use the break
statement with the outerLoop
label to break out of both loops. The second if
statement checks if we want to break out of just the inner loop (when j
is 2), and if so, we use a regular break statement to break out of just the inner loop.
Once the loops are broken, we print a message indicating that they have been broken.
This program demonstrates how you can use a label to break out of a loop, which can be useful in situations where you have nested loops and want to break out of multiple loops at once.
Example-2
Here’s an example Java program with comments that demonstrates how to break a loop from outside of a function:
package crunchify.com.java.tutorials; /** * @author Crunchify.com * Program: In Java how to break a loop from outside? Multiple ways * Method-1 * */ public class CrunchifyBreakLoopExample { private static boolean shouldBreak = false; // declare a static boolean variable to track if the loop should be broken public static void main(String[] args) { int count = 0; // declare a variable to keep track of the number of iterations while (!shouldBreak) { // start a loop that continues until shouldBreak is true System.out.println("Iteration " + count); // print out the current iteration number count++; // increment the iteration count if (count == 5) { // if the iteration count reaches 5 breakLoop(); // call the function that sets shouldBreak to true and breaks the loop } } System.out.println("Loop has been broken"); // print a message indicating that the loop has been broken } private static void breakLoop() { System.out.println("Breaking loop from outside of function"); // print a message indicating that the loop is being broken from outside of the function shouldBreak = true; // set shouldBreak to true, which will cause the loop to terminate } }
We also include an if statement inside the loop that checks if count
is equal to 5. If it is, we call a function called breakLoop()
, which sets shouldBreak
to true and breaks the loop.
The breakLoop()
function simply prints a message indicating that the loop is being broken from outside of the function and sets shouldBreak
to true.
Once the loop is broken, we print a message indicating that the loop has been broken.
This program demonstrates how you can break a loop from outside of a function by using a static variable to keep track of whether the loop should be terminated.
The post In Java how to break a loop from outside? Multiple ways appeared first on Crunchify.
0 Commentaires