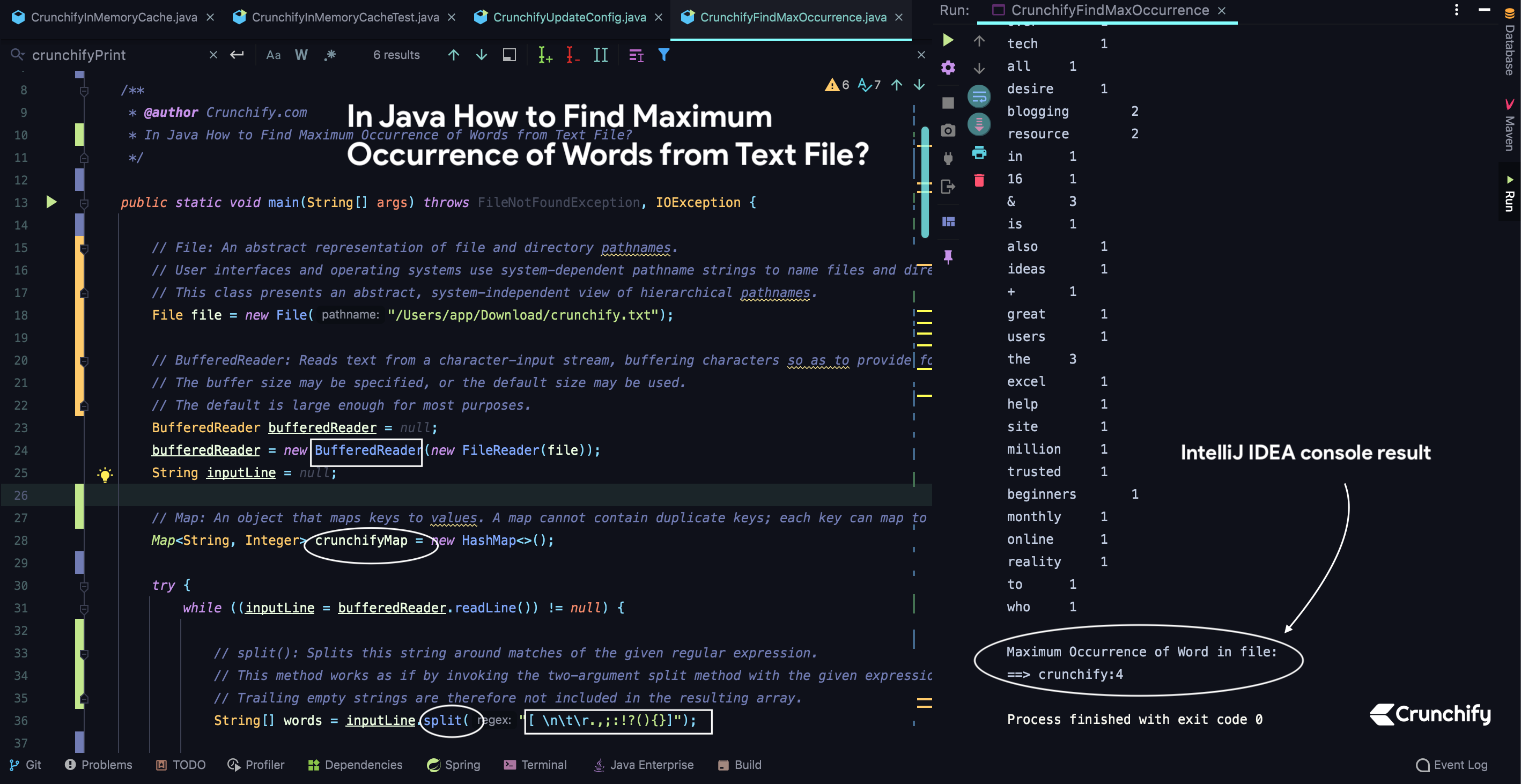
In this Java tutorial, you will learn How to Find Maximum Occurrence of Words from given Text File?
Here is a logic for getting top element:
- Create a class
CrunchifyComparable
that can store the String value of the word and the number of occurrences it appears. - Implement the Comparable interface for this class to
sort by occurrences first and then alphabetically
if the number of occurrences is the same crunchifyFindMaxOccurrence
method, you create a new List ofCrunchifyComparable
from your original map. You add the entries of this to your List- Sort this list
- Take the n-first items of this list using subList
- Add Strings to the
List<String>
and you return it
Another most read
: Find more information on equals() and hashcode()
Java Code:
Create class CrunchifyFindMaxOccurrence.java. Put below code into file.
package crunchify.com.tutorials; import java.io.*; import java.util.*; public class CrunchifyFindMaxOccurrence { /** * @author Crunchify.com * In Java How to Find Maximum Occurrence of Words from Text File? */ public static void main(String[] args) throws FileNotFoundException, IOException { // File: An abstract representation of file and directory pathnames. // User interfaces and operating systems use system-dependent pathname strings to name files and directories. // This class presents an abstract, system-independent view of hierarchical pathnames. File file = new File("/Users/app/Download/crunchify.txt"); // BufferedReader: Reads text from a character-input stream, buffering characters so as to provide for the efficient reading of characters, arrays, and lines. // The buffer size may be specified, or the default size may be used. // The default is large enough for most purposes. BufferedReader bufferedReader = null; bufferedReader = new BufferedReader(new FileReader(file)); String inputLine = null; // Map: An object that maps keys to values. A map cannot contain duplicate keys; each key can map to at most one value. Map<String, Integer> crunchifyMap = new HashMap<>(); try { while ((inputLine = bufferedReader.readLine()) != null) { // split(): Splits this string around matches of the given regular expression. // This method works as if by invoking the two-argument split method with the given expression and a limit argument of zero. // Trailing empty strings are therefore not included in the resulting array. String[] words = inputLine.split("[ \n\t\r.,;:!?(){}]"); for (String word : words) { String key = word.toLowerCase(); // remove .toLowerCase for Case Sensitive result. if (key.length() > 0) { if (crunchifyMap.get(key) == null) { crunchifyMap.put(key, 1); } else { int value = crunchifyMap.get(key).intValue(); value++; crunchifyMap.put(key, value); } } } } // Set: A collection that contains no duplicate elements. // More formally, sets contain no pair of elements e1 and e2 such that e1.equals(e2), // and at most one null element. As implied by its name, this interface models the mathematical set abstraction. Set<Map.Entry<String, Integer>> entrySet = crunchifyMap.entrySet(); crunchifyPrint("Words" + "\t\t" + "# of Occurances"); for (Map.Entry<String, Integer> entry : entrySet) { crunchifyPrint(entry.getKey() + "\t\t" + entry.getValue()); } List<String> myTopOccurrence = crunchifyFindMaxOccurrence(crunchifyMap, 1); crunchifyPrint("\nMaximum Occurrence of Word in file: "); for (String result : myTopOccurrence) { crunchifyPrint("==> " + result); } // IOException: Signals that an I/O exception of some sort has occurred. // This class is the general class of exceptions produced by failed or interrupted I/O operations. } catch (IOException error) { crunchifyPrint("Invalid File"); } finally { bufferedReader.close(); } } private static void crunchifyPrint(String s) { System.out.println(s); } /** * @param map = All Words in map * @param n = How many top elements you want to print? If n=1 it will print the highest occurrence word. If n=2 it * will print top 2 highest occurrence words. * @returns list of String */ public static List<String> crunchifyFindMaxOccurrence(Map<String, Integer> map, int n) { List<CrunchifyComparable> l = new ArrayList<>(); for (Map.Entry<String, Integer> entry : map.entrySet()) l.add(new CrunchifyComparable(entry.getKey(), entry.getValue())); // sort(): Sorts the specified list into ascending order, according to the natural ordering of its elements. // All elements in the list must implement the Comparable interface. Furthermore, all elements in the list must be mutually comparable // (that is, e1.compareTo(e2) must not throw a ClassCastException for any elements e1 and e2 in the list). Collections.sort(l); List<String> list = new ArrayList<>(); // subList(): Returns a view of the portion of this list between the specified fromIndex, // inclusive, and toIndex, exclusive. (If fromIndex and toIndex are equal, the returned list is empty.) // The returned list is backed by this list, so non-structural changes in the returned list are reflected in this list, and vice-versa. // The returned list supports all of the optional list operations supported by this list. for (CrunchifyComparable w : l.subList(0, n)) list.add(w.wordFromFile + ":" + w.numberOfOccurrence); return list; } } class CrunchifyComparable implements Comparable<CrunchifyComparable> { public String wordFromFile; public int numberOfOccurrence; public CrunchifyComparable(String wordFromFile, int numberOfOccurrence) { super(); this.wordFromFile = wordFromFile; this.numberOfOccurrence = numberOfOccurrence; } @Override public int compareTo(CrunchifyComparable arg0) { int crunchifyCompare = Integer.compare(arg0.numberOfOccurrence, this.numberOfOccurrence); return crunchifyCompare != 0 ? crunchifyCompare : wordFromFile.compareTo(arg0.wordFromFile); } @Override public int hashCode() { final int uniqueNumber = 19; int crunchifyResult = 9; crunchifyResult = uniqueNumber * crunchifyResult + numberOfOccurrence; // hasCode(): Returns a hash code for this string. The hash code for a String object is computed as // s[0]*31^(n-1) + s[1]*31^(n-2) + ... + s[n-1] // // using int arithmetic, where s[i] is the ith character of the string, n is the length of the string, // and ^ indicates exponentiation. (The hash value of the empty string is zero.) crunchifyResult = uniqueNumber * crunchifyResult + ((wordFromFile == null) ? 0 : wordFromFile.hashCode()); return crunchifyResult; } // Override: Indicates that a method declaration is intended to override a method declaration in a supertype. // If a method is annotated with this annotation type compilers are required // to generate an error message unless at least one of the following conditions hold: // The method does override or implement a method declared in a supertype. // The method has a signature that is override-equivalent to that of any public method declared in Object. @Override public boolean equals(Object crunchifyObj) { if (this == crunchifyObj) return true; if (crunchifyObj == null) return false; if (getClass() != crunchifyObj.getClass()) return false; CrunchifyComparable other = (CrunchifyComparable) crunchifyObj; if (numberOfOccurrence != other.numberOfOccurrence) return false; if (wordFromFile == null) { if (other.wordFromFile != null) return false; } else if (!wordFromFile.equals(other.wordFromFile)) return false; return true; } }
Example-1 file:
Crunchify is the largest free, premium, technical & blogging resource site for beginners, who are passionate & have the desire to excel in the online world. Attracting over 16 million monthly users, we are leading & trusted tech + blogging resource for all. We also help clients transform their great ideas into reality! Crunchify, Crunchify, Crunchify.com
Output:
Here is a IntelliJ IDEA console result. Run above program as a Java Application and you are will see result as below.
/Library/Java/JavaVirtualMachines/jdk-15.jdk/Contents/Home/bin/java -javaagent:/Applications/IntelliJ IDEA.app/Contents/lib/idea_rt.jar=50339:/Applications/IntelliJ IDEA.app/Contents/bin -Dfile.encoding=UTF-8 -classpath /Users/app/crunchify/github/CrunchifyTutorials/target/classes:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/zxing-2.1.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/commons-logging-1.1.2.jar :/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/commons-collections-3.2.1.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/javax.mail.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/commons-io-2.4.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/commons-lang-2.6.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/commons-configuration-1.9.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/log4j-1.2.17.jar:/Users/app/crunchify/github/CrunchifyTutorials/WebContent/WEB-INF/lib/commons-beanutils-1.8.3.jar :/Users/app/Library/Application Support/JetBrains/IntelliJIdea2021.2/javascript/extLibs/http_code.jquery.com_jquery-3.6.0.js:/Users/app/.m2/repository/org/glassfish/javax.json/1.1.4/javax.json-1.1.4.jar:/Users/app/.m2/repository/com/github/wnameless/json-flattener/0.2.2/json-flattener-0.2.2.jar:/Users/app/.m2/repository/com/eclipsesource/minimal-json/minimal-json/0.9.4/minimal-json-0.9.4.jar:/Users/app/.m2/repository/com/github/wnameless/json/json-flattener/0.12.0/json-flattener-0.12.0.jar :/Users/app/.m2/repository/com/fasterxml/jackson/core/jackson-databind/2.12.0/jackson-databind-2.12.0.jar:/Users/app/.m2/repository/com/fasterxml/jackson/core/jackson-annotations/2.12.0/jackson-annotations-2.12.0.jar:/Users/app/.m2/repository/com/fasterxml/jackson/core/jackson-core/2.12.0/jackson-core-2.12.0.jar :/Users/app/.m2/repository/org/apache/commons/commons-text/1.9/commons-text-1.9.jar:/Users/app/.m2/repository/com/github/wnameless/json/json-base/2.0.0/json-base-2.0.0.jar:/Users/app/.m2/repository/commons-io/commons-io/2.10.0/commons-io-2.10.0.jar :/Users/app/.m2/repository/com/google/code/gson/gson/2.8.7/gson-2.8.7.jar:/Users/app/.m2/repository/net/jodah/expiringmap/0.5.9/expiringmap-0.5.9.jar:/Users/app/.m2/repository/org/apache/httpcomponents/httpclient/4.5.13/httpclient-4.5.13.jar:/Users/app/.m2/repository/org/apache/httpcomponents/httpcore/4.4.13/httpcore-4.4.13.jar :/Users/app/.m2/repository/commons-codec/commons-codec/1.11/commons-codec-1.11.jar:/Users/app/.m2/repository/org/json/json/20210307/json-20210307.jar:/Users/app/.m2/repository/net/spy/spymemcached/2.12.3/spymemcached-2.12.3.jar:/Users/app/.m2/repository/com/whalin/Memcached-Java-Client/3.0.2/Memcached-Java-Client-3.0.2.jar :/Users/app/.m2/repository/commons-pool/commons-pool/1.5.6/commons-pool-1.5.6.jar:/Users/app/.m2/repository/com/googlecode/xmemcached/xmemcached/2.4.7/xmemcached-2.4.7.jar:/Users/app/.m2/repository/com/paypal/sdk/rest-api-sdk/1.14.0/rest-api-sdk-1.14.0.jar :/Users/app/.m2/repository/org/apache/commons/commons-dbcp2/2.8.0/commons-dbcp2-2.8.0.jar:/Users/app/.m2/repository/org/apache/commons/commons-pool2/2.8.1/commons-pool2-2.8.1.jar:/Users/app/.m2/repository/commons-dbcp/commons-dbcp/20030825.184428/commons-dbcp-20030825.184428.jar:/Users/app/.m2/repository/javax/ws/rs/javax.ws.rs-api/2.1.1/javax.ws.rs-api-2.1.1.jar:/Users/app/.m2/repository/org/hamcrest/hamcrest-all/1.3/hamcrest-all-1.3.jar:/Users/app/.m2/repository/org/apache/logging/log4j/log4j-core/2.14.1/log4j-core-2.14.1.jar:/Users/app/.m2/repository/org/apache/logging/log4j/log4j-api/2.14.1/log4j-api-2.14.1.jar :/Users/app/.m2/repository/com/google/guava/guava/30.1.1-jre/guava-30.1.1-jre.jar:/Users/app/.m2/repository/com/google/guava/failureaccess/1.0.1/failureaccess-1.0.1.jar:/Users/app/.m2/repository/com/google/guava/listenablefuture/9999.0-empty-to-avoid-conflict-with-guava/listenablefuture-9999.0-empty-to-avoid-conflict-with-guava.jar:/Users/app/.m2/repository/com/google/code/findbugs/jsr305/3.0.2/jsr305-3.0.2.jar :/Users/app/.m2/repository/org/checkerframework/checker-qual/3.8.0/checker-qual-3.8.0.jar:/Users/app/.m2/repository/com/google/errorprone/error_prone_annotations/2.5.1/error_prone_annotations-2.5.1.jar:/Users/app/.m2/repository/com/google/j2objc/j2objc-annotations/1.3/j2objc-annotations-1.3.jar:/Users/app/.m2/repository/com/googlecode/json-simple/json-simple/1.1.1/json-simple-1.1.1.jar:/Users/app/.m2/repository/junit/junit/4.10/junit-4.10.jar:/Users/app/.m2/repository/org/hamcrest/hamcrest-core/1.1/hamcrest-core-1.1.jar:/Users/app/.m2/repository/commons-net/commons-net/3.8.0/commons-net-3.8.0.jar:/Users/app/.m2/repository/org/ow2/asm/asm/9.1/asm-9.1.jar:/Users/app/.m2/repository/axis/axis/1.4/axis-1.4.jar:/Users/app/.m2/repository/org/apache/axis/axis-jaxrpc/1.4/axis-jaxrpc-1.4.jar:/Users/app/.m2/repository/axis/axis-wsdl4j/1.5.1/axis-wsdl4j-1.5.1.jar:/Users/app/.m2/repository/commons-beanutils/commons-beanutils/1.9.4/commons-beanutils-1.9.4.jar:/Users/app/.m2/repository/commons-collections/commons-collections/3.2.2/commons-collections-3.2.2.jar:/Users/app/.m2/repository/org/apache/commons/commons-collections4/4.4/commons-collections4-4.4.jar:/Users/app/.m2/repository/org/apache/commons/commons-configuration2/2.7/commons-configuration2-2.7.jar :/Users/app/.m2/repository/commons-discovery/commons-discovery/0.5/commons-discovery-0.5.jar:/Users/app/.m2/repository/org/apache/commons/commons-lang3/3.12.0/commons-lang3-3.12.0.jar:/Users/app/.m2/repository/commons-logging/commons-logging/1.2/commons-logging-1.2.jar:/Users/app/.m2/repository/commons-logging/commons-logging-api/1.1/commons-logging-api-1.1.jar:/Users/app/.m2/repository/javax/mail/javax.mail-api/1.6.2/javax.mail-api-1.6.2.jar:/Users/app/.m2/repository/javax/mail/mail/1.4.7/mail-1.4.7.jar:/Users/app/.m2/repository/javax/activation/activation/1.1/activation-1.1.jar:/Users/app/.m2/repository/javax/xml/jaxrpc-api/1.1/jaxrpc-api-1.1.jar:/Users/app/.m2/repository/org/apache/axis/axis-saaj/1.4/axis-saaj- 1.4.jar:/Users/app/.m2/repository/wsdl4j/wsdl4j/1.6.3/wsdl4j-1.6.3.jar:/Users/app/.m2/repository/com/google/zxing/core/3.4.1/core-3.4.1.jar:/Users/app/.m2/repository/org/apache/commons/commons-compress/1.20/commons-compress-1.20.jar:/Users/app/.m2/repository/mysql/mysql-connector-java/8.0.25/mysql-connector-java-8.0.25.jar:/Users/app/.m2/repository/com/google/protobuf/protobuf-java/3.11.4/protobuf-java-3.11.4.jar :/Users/app/.m2/repository/ch/qos/logback/logback-classic/1.3.0-alpha5/logback-classic-1.3.0-alpha5.jar:/Users/app/.m2/repository/ch/qos/logback/logback-core/1.3.0-alpha5/logback-core-1.3.0-alpha5.jar:/Users/app/.m2/repository/com/sun/mail/javax.mail/1.6.2/javax.mail-1.6.2.jar:/Users/app/.m2/repository/edu/washington/cs/types/checker/checker-framework/1.7.0/checker-framework-1.7.0.jar:/Users/app/.m2/repository/commons-validator/commons-validator/1.7/commons-validator-1.7.jar:/Users/app/.m2/repository/commons-digester/commons-digester/2.1/commons-digester-2.1.jar:/Users/app/.m2/repository/org/springframework/spring-context/5.3.8/spring-context-5.3.8.jar:/Users/app/.m2/repository/org/springframework/spring-aop/5.3.8/spring-aop-5.3.8.jar:/Users/app/.m2/repository/org/springframework/spring-beans/5.3.8/spring-beans-5.3.8.jar :/Users/app/.m2/repository/org/springframework/spring-core/5.3.8/spring-core-5.3.8.jar:/Users/app/.m2/repository/org/springframework/spring-jcl/5.3.8/spring-jcl-5.3.8.jar:/Users/app/.m2/repository/org/springframework/spring-expression/5.3.8/spring-expression-5.3.8.jar:/Users/app/.m2/repository/org/springframework/spring-context-support/5.3.8/spring-context-support-5.3.8.jar:/Users/app/.m2/repository/org/slf4j/slf4j-api/1.7.31/slf4j-api-1.7.31.jar:/Users/app/.m2/repository/org/slf4j/jul-to-slf4j/1.7.31/jul-to-slf4j-1.7.31.jar:/Users/app/.m2/repository/org/slf4j/jcl-over-slf4j/1.7.31/jcl-over-slf4j-1.7.31.jar:/Users/app/.m2/repository/org/slf4j/log4j-over-slf4j/1.7.31/log4j-over-slf4j-1.7.31.jar crunchify.com.tutorials.CrunchifyFindMaxOccurrence Words # of Occurances com 1 largest 1 clients 1 technical 1 passionate 1 leading 1 for 2 their 1 we 2 into 1 transform 1 premium 1 world 1 are 2 attracting 1 have 1 free 1 crunchify 4 over 1 tech 1 all 1 desire 1 blogging 2 resource 2 in 1 16 1 & 3 is 1 also 1 ideas 1 + 1 great 1 users 1 the 3 excel 1 help 1 site 1 million 1 trusted 1 beginners 1 monthly 1 online 1 reality 1 to 1 who 1 Maximum Occurrence of Word in file: ==> crunchify:4 Process finished with exit code 0
Example-2 File:
this and and and then and this
Output:
Words # of Occurances and 4 this 2 then 1 Maixmum Occurance of Word in file: ==> and:4
Are you looking for more java tutorials? Look at this collection.
The post In Java How to Find Maximum Occurrence of Words from Text File? appeared first on Crunchify.
0 Commentaires