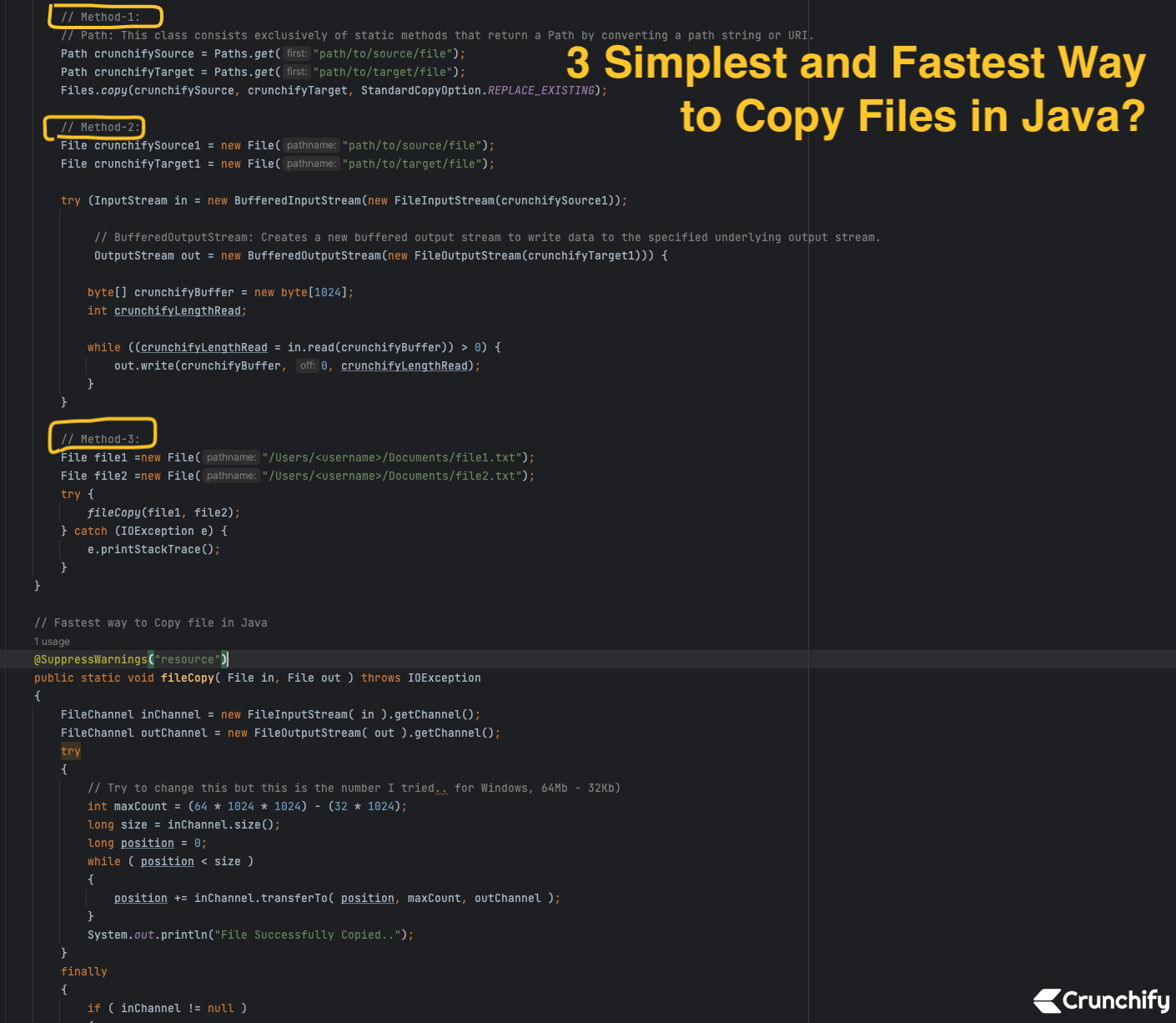
There are multiple ways to copy a file in Java, but the fastest way would depend on several factors such as the size of the file, the available system resources, and the desired level of concurrency. Here are some options to consider:
Method-1. Using NIO (Java NIO):
Java NIO provides a set of classes that offer faster and more efficient file operations than the traditional IO classes. You can use the Files.copy()
method to copy a file using NIO. This method uses the native operating system’s file copying mechanism, which can be much faster than copying the file byte by byte.
package crunchify.com.tutorials; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.nio.file.StandardCopyOption; /** * @author Crunchify.com * Program: 3 Simplest and Fastest Way to Copy Files in Java? */ public class CrunchifyFileCopy { public class FileCopyExample { public static void main(String[] args) throws Exception { // Path: This class consists exclusively of static methods that return a Path by converting a path string or URI. Path crunchifySource = Paths.get("path/to/source/file"); Path crunchifyTarget = Paths.get("path/to/target/file"); // Files: This class consists exclusively of static methods that operate on files, directories, or other types of files. // copy(): Copy a file to a target file. //This method copies a file to the target file with the options parameter specifying how the copy is performed. // By default, the copy fails if the target file already exists or is a symbolic link, except if the source and target are the same file, // in which case the method completes without copying the file. File attributes are not required to be copied to the target file. // If symbolic links are supported, and the file is a symbolic link, then the final target of the link is copied. // If the file is a directory then it creates an empty directory in the target location (entries in the directory are not copied). // This method can be used with the walkFileTree method to copy a directory and all entries in the directory, or an entire file-tree where required. Files.copy(crunchifySource, crunchifyTarget, StandardCopyOption.REPLACE_EXISTING); } } }
Method-2. Using Buffered Streams:
Another way to copy a file in Java is to use Buffered Streams. Buffered Streams use an internal buffer to read and write data, which can reduce the number of I/O operations needed and improve performance.
package crunchify.com.tutorials; import java.io.*; /** * @author Crunchify.com * Program: 3 Simplest and Fastest Way to Copy Files in Java? */ public class CrunchifyFileCopy { public class FileCopyExample { public static void main(String[] args) throws Exception { File crunchifySource = new File("path/to/source/file"); File crunchifyTarget = new File("path/to/target/file"); // BufferedInputStream: Creates a BufferedInputStream and saves its argument, the input stream in, for later use. // An internal buffer array is created and stored in buf. try (InputStream in = new BufferedInputStream(new FileInputStream(crunchifySource)); // BufferedOutputStream: Creates a new buffered output stream to write data to the specified underlying output stream. OutputStream out = new BufferedOutputStream(new FileOutputStream(crunchifyTarget))) { byte[] crunchifyBuffer = new byte[1024]; int crunchifyLengthRead; while ((crunchifyLengthRead = in.read(crunchifyBuffer)) > 0) { out.write(crunchifyBuffer, 0, crunchifyLengthRead); } } } } }
Method-3. Using FileChannel:
How to copy file in Java from one directory to another is common requirement. Java didn’t come with any ready made code to copy file. Below method is the Fastest Way
to Copy File in Java?
package com.crunchify.tutorials; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.nio.channels.FileChannel; /** * @author Crunchify.com */ public class CrunchifyFileCopy { public static void main(String[] args) { File file1 =new File("/Users/<username>/Documents/file1.txt"); File file2 =new File("/Users/<username>/Documents/file2.txt"); try { fileCopy(file1, file2); } catch (IOException e) { e.printStackTrace(); } } // Fastest way to Copy file in Java @SuppressWarnings("resource") public static void fileCopy( File in, File out ) throws IOException { FileChannel inChannel = new FileInputStream( in ).getChannel(); FileChannel outChannel = new FileOutputStream( out ).getChannel(); try { // Try to change this but this is the number I tried.. for Windows, 64Mb - 32Kb) int maxCount = (64 * 1024 * 1024) - (32 * 1024); long size = inChannel.size(); long position = 0; while ( position < size ) { position += inChannel.transferTo( position, maxCount, outChannel ); } System.out.println("File Successfully Copied.."); } finally { if ( inChannel != null ) { inChannel.close(); } if ( outChannel != null ) { outChannel.close(); } } } }
Let me know if you face any issue running this code in your Production environment.
The post 3 Simplest and Fastest Way to Copy Files in Java? appeared first on Crunchify.
0 Commentaires