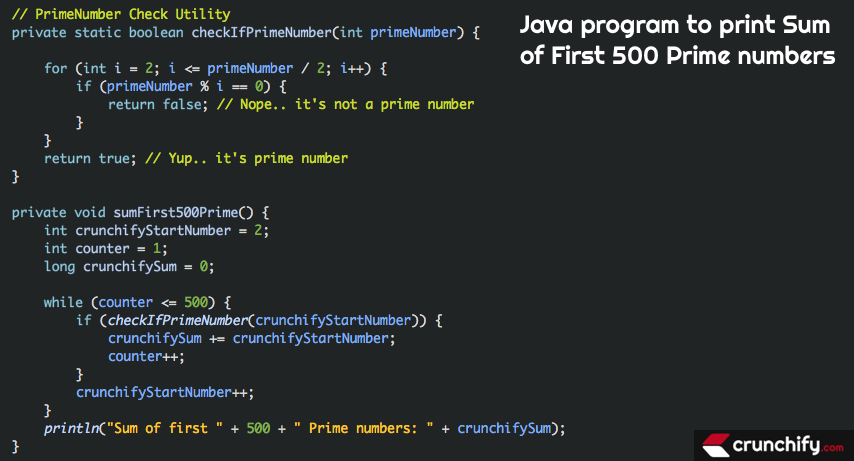
What is a Prime Number?
A number which is greater than 1 and which has no positive divisors other than 1 and itself. Sometime back I’ve written a tutorial which shows clearly if provided number is prime or not.
In this tutorial we will go over details on how to print Sum of First 500 Prime numbers
. We will also provide an option for users to enter a number and Java Program will sums up provided total first Prime numbers
Scanner class constructs a new Scanner that produces values scanned from the specified input stream.
Let’s get started:
- Create Java class
CrunchifySumOfNPrimeNumbers
.java sumFirst500Prime()
method will print sum of first 500 Prime numbers- Next we will ask user to enter number and program will sums up total that many numbers
checkIfPrimeNumber()
is a method to find out if number is prime or not?
Here is a complete Java program to print sum of first 500 prime numbers.
package crunchify.com.tutorial; import java.util.Scanner; /** * @author Crunchify.com * Program: Java program to print Sum of First 500 Prime numbers (or First N Prime numbers) * Version: 1.0.1 * */ public class CrunchifySumOfNPrimeNumbers { public static void main(String args[]) { CrunchifySumOfNPrimeNumbers object = new CrunchifySumOfNPrimeNumbers(); object.sumFirst500Prime(); @SuppressWarnings("resource") Scanner reader = new Scanner(System.in); // Reading from System.in System.out.println("\nEnter a number: "); int myNumber = reader.nextInt(); int crunchifyStartNumber = 2; int counter = 1; int crunchifySum = 0; while (counter <= myNumber) { if (checkIfPrimeNumber(crunchifyStartNumber)) { crunchifySum += crunchifyStartNumber; counter++; } crunchifyStartNumber++; } println("Sum of first " + myNumber + " Prime numbers: " + crunchifySum); } private void sumFirst500Prime() { int crunchifyStartNumber = 2; int counter = 1; long crunchifySum = 0; while (counter <= 500) { if (checkIfPrimeNumber(crunchifyStartNumber)) { crunchifySum += crunchifyStartNumber; counter++; } crunchifyStartNumber++; } println("Sum of first " + 500 + " Prime numbers: " + crunchifySum); } // Simple Println Utility private static void println(String crunchifySum) { System.out.println(crunchifySum); } // PrimeNumber Check Utility private static boolean checkIfPrimeNumber(int primeNumber) { for (int i = 2; i <= primeNumber / 2; i++) { if (primeNumber % i == 0) { return false; // Nope.. it's not a prime number } } return true; // Yup.. it's prime number } }
Run the program in Eclipse IDE and you will be able find similar result:
Sum of first 500 Prime numbers: 824693 Enter a number: 800 Sum of first 800 Prime numbers: 2277959
Why is the number 1 not a prime number?
As per a Prime number definition, Prime number is a number which is always greater than 1
. Number 3 and 5 are prime numbers as both numbers divided by itself and 1.
What is Composite Number?
A whole number that can be divided evenly by numbers other than 1 or itself is called Composite Number.
The post In Java How to print Sum of First 500 Prime numbers (or First N Prime numbers) appeared first on Crunchify.
0 Commentaires