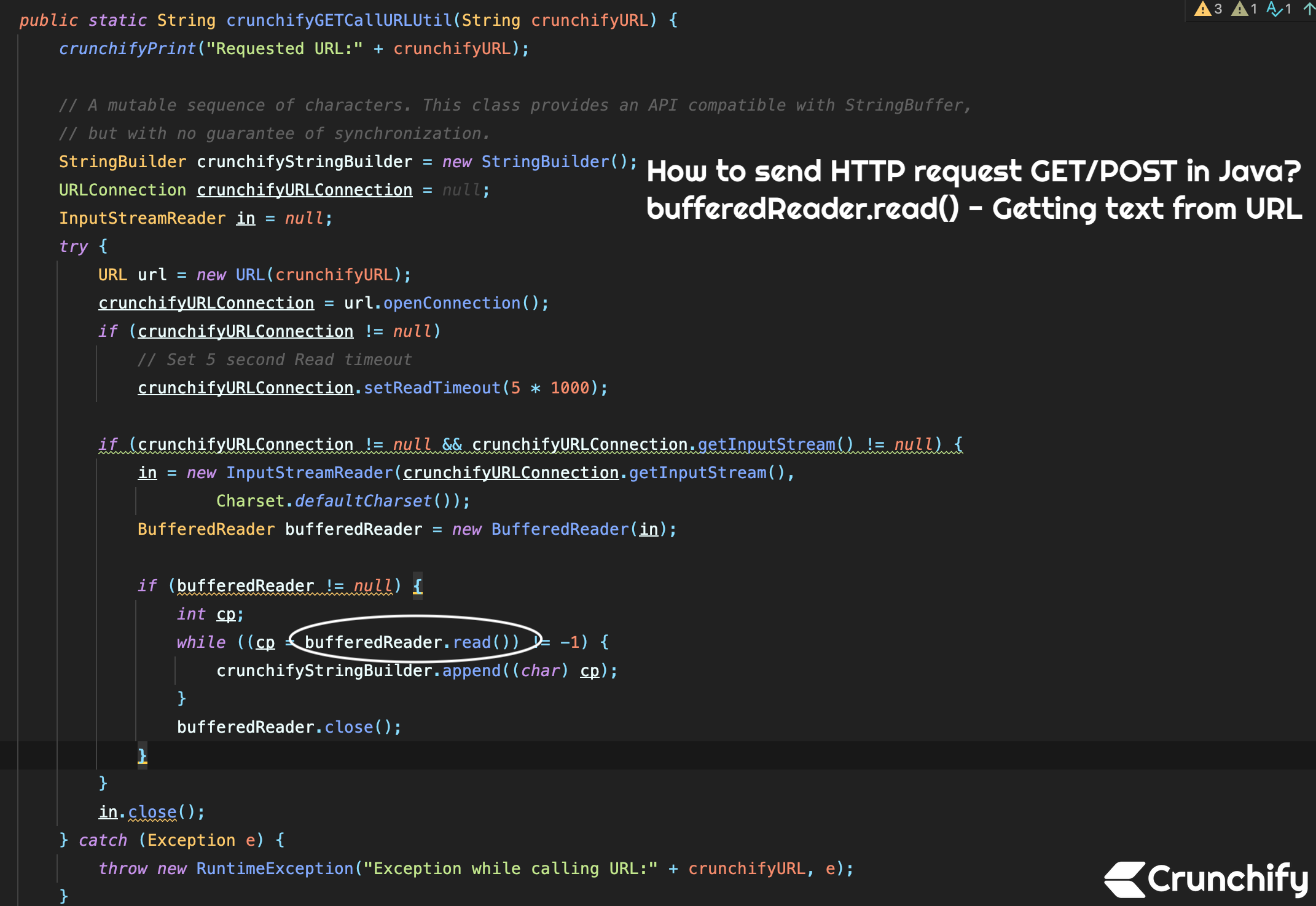
How to send HTTP request GET/POST in Java?
How to use java.net.URLConnection to fire and handle HTTP? Below is a simple example to get Response from URL in Java Program.
The URLConnection
class contains many methods that let you communicate with the URL over the network. URLConnection
is an HTTP-centric class; that is, many of its methods are useful only when you are working with HTTP URLs. However, most URL protocols allow you to read from and write to the connection. urlconnection getinputstream
usage.
This section describes both functions.
package crunchify.com.tutorials; import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.URL; import java.net.URLConnection; import java.nio.charset.Charset; /** * @author Crunchify.com * Getting text from URL: Send HTTP request GET/POST in Java - bufferedReader.read() */ public class CrunchifycallUrlAndGetResponse { public static void main(String[] args) { crunchifyPrint("\nOutput: \n" + crunchifyGETCallURLUtil("https://crunchify.com/wp-content/uploads/code/json.sample.txt")); } public static String crunchifyGETCallURLUtil(String crunchifyURL) { crunchifyPrint("Requested URL:" + crunchifyURL); // A mutable sequence of characters. This class provides an API compatible with StringBuffer, // but with no guarantee of synchronization. StringBuilder crunchifyStringBuilder = new StringBuilder(); URLConnection crunchifyURLConnection = null; InputStreamReader in = null; try { URL url = new URL(crunchifyURL); crunchifyURLConnection = url.openConnection(); if (crunchifyURLConnection != null) // Set 5 second Read timeout crunchifyURLConnection.setReadTimeout(5 * 1000); if (crunchifyURLConnection != null && crunchifyURLConnection.getInputStream() != null) { in = new InputStreamReader(crunchifyURLConnection.getInputStream(), Charset.defaultCharset()); BufferedReader bufferedReader = new BufferedReader(in); if (bufferedReader != null) { int cp; while ((cp = bufferedReader.read()) != -1) { crunchifyStringBuilder.append((char) cp); } bufferedReader.close(); } } in.close(); } catch (Exception e) { throw new RuntimeException("Exception while calling URL:" + crunchifyURL, e); } return crunchifyStringBuilder.toString(); } private static void crunchifyPrint(String print) { System.out.println(print); } }
Just run above program in Eclipse Console or IntelliJ IDEA and you should see same result as below.
Requested URL:https://crunchify.com/wp-content/uploads/code/json.sample.txt Output: {"menu": { "id": "file", "value": "File", "popup": { "menuitem": [ {"value": "New", "onclick": "CreateNewDoc()"}, {"value": "Open", "onclick": "OpenDoc()"}, {"value": "Close", "onclick": "CloseDoc()"} ] } }}
The post Java URL example: How to send HTTP request GET/POST in Java? bufferedReader.read() – Getting text from URL appeared first on Crunchify.
0 Commentaires