Have you ever wondered how to efficiently find the third smallest number in an array, list, or stream of integers? In this blog post, we’ll dive into the world of Java programming and explore different techniques for identifying the third smallest number in various data sources.
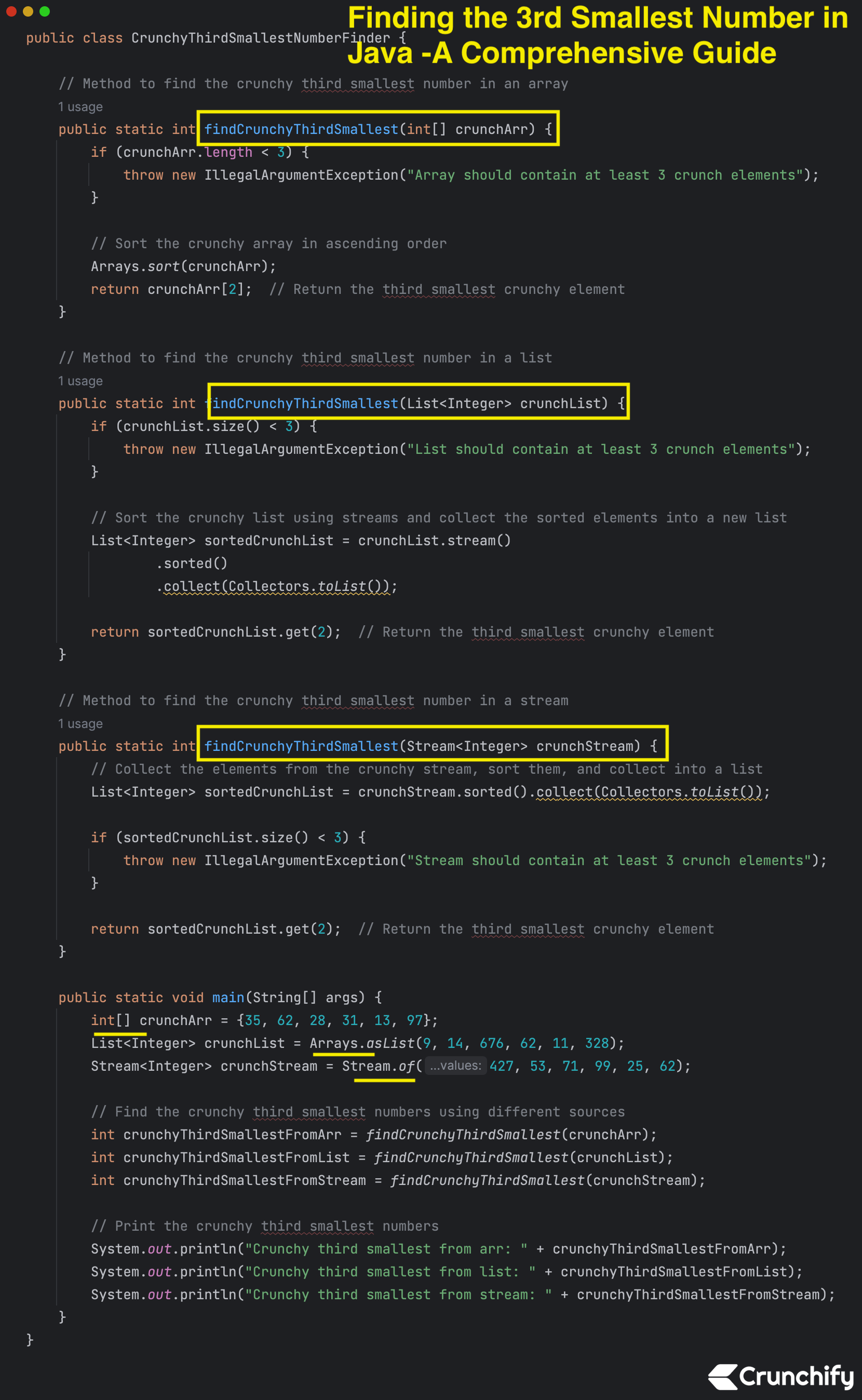
When working with data manipulation and analysis, the need often arises to identify specific elements within a collection of numbers. Finding the smallest or largest number is relatively straightforward, but determining the third smallest number requires a bit more finesse. In this blog post, we’ll cover three methods for tackling this problem using Java: sorting an array, sorting a list, and utilizing streams.
Method 1: Sorting an Array
Arrays are one of the fundamental data structures in Java, making them a natural starting point for our exploration. The idea here is to sort the array in ascending order and then retrieve the element at index 2.
Method 2: Sorting a List
Lists provide a more flexible alternative to arrays, allowing for dynamic resizing and easier manipulation. To find the third smallest number in a list, we can follow a similar approach to array sorting.
Method 3: Utilizing Streams
Java streams are a powerful way to process sequences of elements. We can use streams to sort the elements and then retrieve the third smallest number.
Choosing the Right Method
Each method has its own advantages and use cases. If you have an array at hand, the array sorting method might be the most efficient. Lists are versatile and offer more convenience, while streams provide a functional approach and can handle larger datasets with ease.
Here is a complete example:
CrunchyThirdSmallestNumberFinder.java
package crunchify.com.tutorial; import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; import java.util.stream.Stream; /** * @author Crunchify.com * Finding the 3rd Smallest Number in Java: A Comprehensive Guide * */ public class CrunchyThirdSmallestNumberFinder { // Method to find the crunchy third smallest number in an array public static int findCrunchyThirdSmallest(int[] crunchArr) { if (crunchArr.length < 3) { throw new IllegalArgumentException("Array should contain at least 3 crunch elements"); } // Sort the crunchy array in ascending order Arrays.sort(crunchArr); return crunchArr[2]; // Return the third smallest crunchy element } // Method to find the crunchy third smallest number in a list public static int findCrunchyThirdSmallest(List<Integer> crunchList) { if (crunchList.size() < 3) { throw new IllegalArgumentException("List should contain at least 3 crunch elements"); } // Sort the crunchy list using streams and collect the sorted elements into a new list List<Integer> sortedCrunchList = crunchList.stream() .sorted() .collect(Collectors.toList()); return sortedCrunchList.get(2); // Return the third smallest crunchy element } // Method to find the crunchy third smallest number in a stream public static int findCrunchyThirdSmallest(Stream<Integer> crunchStream) { // Collect the elements from the crunchy stream, sort them, and collect into a list List<Integer> sortedCrunchList = crunchStream.sorted().collect(Collectors.toList()); if (sortedCrunchList.size() < 3) { throw new IllegalArgumentException("Stream should contain at least 3 crunch elements"); } return sortedCrunchList.get(2); // Return the third smallest crunchy element } public static void main(String[] args) { int[] crunchArr = {35, 62, 28, 31, 13, 97}; List<Integer> crunchList = Arrays.asList(9, 14, 676, 62, 11, 328); Stream<Integer> crunchStream = Stream.of(427, 53, 71, 99, 25, 62); // Find the crunchy third smallest numbers using different sources int crunchyThirdSmallestFromArr = findCrunchyThirdSmallest(crunchArr); int crunchyThirdSmallestFromList = findCrunchyThirdSmallest(crunchList); int crunchyThirdSmallestFromStream = findCrunchyThirdSmallest(crunchStream); // Print the crunchy third smallest numbers System.out.println("Crunchy third smallest from arr: " + crunchyThirdSmallestFromArr); System.out.println("Crunchy third smallest from list: " + crunchyThirdSmallestFromList); System.out.println("Crunchy third smallest from stream: " + crunchyThirdSmallestFromStream); } }
Eclipse Console Result:
Just run above program in Eclipse IDE or IntelliJ IDEA and you will result as below.
Crunchy third smallest from arr: 31 Crunchy third smallest from list: 14 Crunchy third smallest from stream: 62 Process finished with exit code 0
There are some performance differences to consider:
In terms of time complexity, all three methods have similar sorting steps, which generally have a time complexity of O(n log n)
, where ‘n’ is the number of elements in the collection (array, list, or stream).
The time complexity of accessing the third smallest element is constant, O(1)
, since it’s a simple array or list lookup.
- Array Method: This method sorts the array in place, so it doesn’t require additional memory for a new sorted collection. This can lead to slightly better memory usage compared to the list and stream methods.
- List Method: This method converts the list into a sorted list using streams. While convenient, it involves creating a new list, which consumes additional memory. This can be a concern if memory usage is crucial.
- Stream Method: The stream method is quite flexible and functional, but it involves collecting the stream into a list. This adds a small overhead in terms of memory and processing compared to directly sorting the array.
Let me know if you face any issue running this code.
The post Finding the 3rd Smallest Number in Java: A Complete Guide appeared first on Crunchify.
0 Commentaires