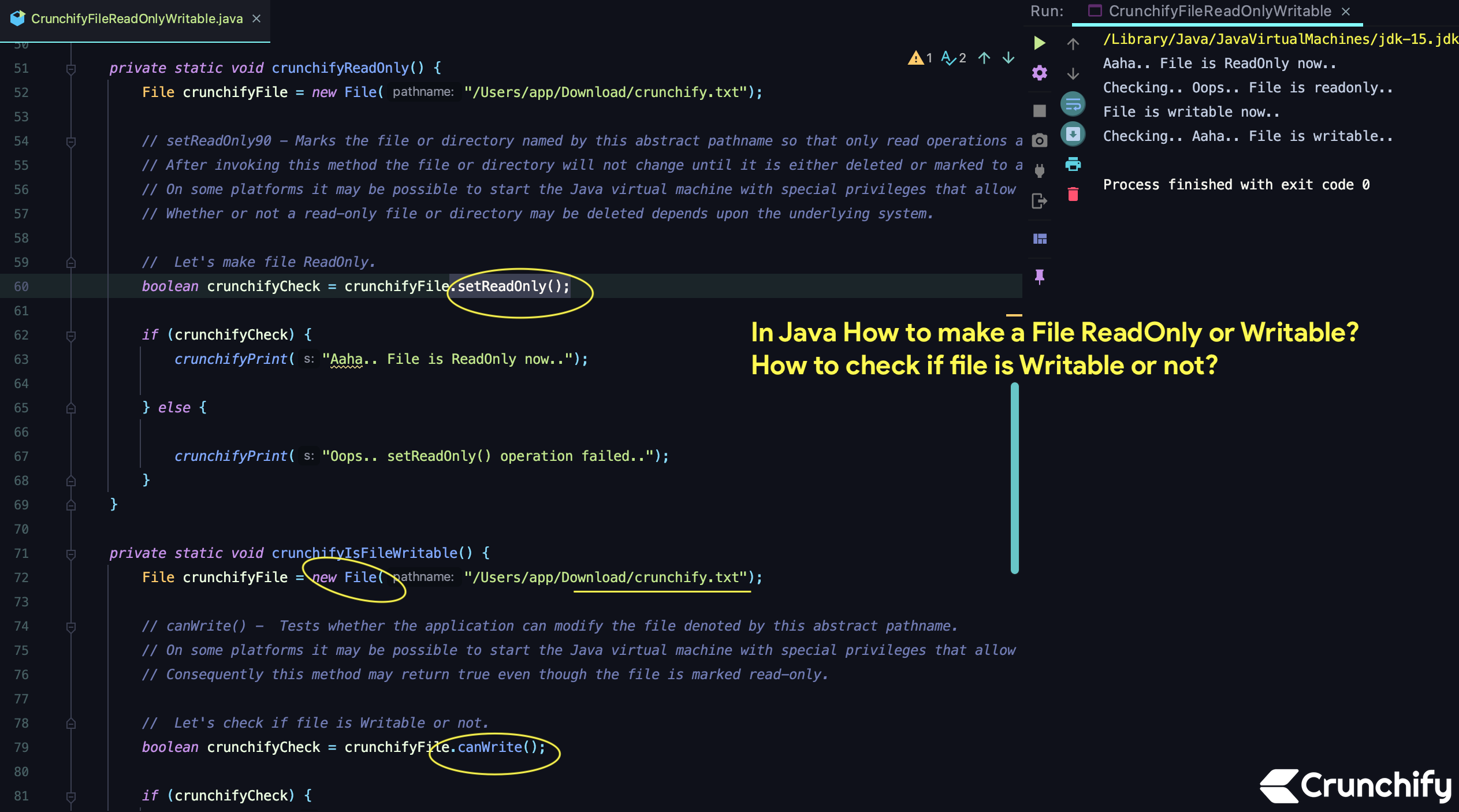
In Java how to make file Read only or Writable? Also, how to check if file is Writable or not?
In this tutorial we will go over below different File Operations.
- file.createNewFile()
- file.canWrite()
- file.setWritable(true)
- file.setReadOnly()
If you have any of below questions then you are at right place.
- Why can we change the file attributes of a read-only file in Java?
- How to change file permission to Read-Only in Linux or MacOS?
- Can I set a file to read-only in Java?
- How to open a file as read-only with java?
Create Java class: CrunchifyFileReadOnlyWritable.java
package crunchify.com.java.tutorials; import java.io.File; import java.io.IOException; /** * @author Crunchify.com * In Java How to make a File ReadOnly or Writable? How to check if file is Writable or not? */ public class CrunchifyFileReadOnlyWritable { public static void main(String[] args) throws IOException { try { // Let's make file Read Only crunchifyReadOnly(); Thread.sleep(10000); // Let's double check if file is Writable or not? crunchifyIsFileWritable(); Thread.sleep(10000); // Let's make file Writable crunchifyMakeWritable(); Thread.sleep(10000); // Let's double check if file is Writable or not? crunchifyIsFileWritable(); // Extra code to create new file in Java. By default it will be writable. File file = new File("/Users/app/Download/newCrunchify.txt"); try { // createNewFile() - Atomically creates a new, empty file named by this abstract pathname if and only if a file with this name does not yet exist. // The check for the existence of the file and the creation of the file if it does not exist are a single // operation that is atomic with respect to all other filesystem activities that might affect the file. // Note: this method should not be used for file-locking, as the resulting protocol cannot be made to work reliably. The FileLock facility should be used instead. boolean newCrunchifyFile = file.createNewFile(); } catch (IOException e) { e.printStackTrace(); } } catch (InterruptedException e) { e.printStackTrace(); } } private static void crunchifyReadOnly() { File crunchifyFile = new File("/Users/app/Download/crunchify.txt"); // setReadOnly90 - Marks the file or directory named by this abstract pathname so that only read operations are allowed. // After invoking this method the file or directory will not change until it is either deleted or marked to allow write access. // On some platforms it may be possible to start the Java virtual machine with special privileges that allow it to modify files that are marked read-only. // Whether or not a read-only file or directory may be deleted depends upon the underlying system. // Let's make file ReadOnly. boolean crunchifyCheck = crunchifyFile.setReadOnly(); if (crunchifyCheck) { crunchifyPrint("Aaha.. File is ReadOnly now.."); } else { crunchifyPrint("Oops.. setReadOnly() operation failed.."); } } private static void crunchifyIsFileWritable() { File crunchifyFile = new File("/Users/app/Download/crunchify.txt"); // canWrite() - Tests whether the application can modify the file denoted by this abstract pathname. // On some platforms it may be possible to start the Java virtual machine with special privileges that allow it to modify files that are marked read-only. // Consequently this method may return true even though the file is marked read-only. // Let's check if file is Writable or not. boolean crunchifyCheck = crunchifyFile.canWrite(); if (crunchifyCheck) { crunchifyPrint("Checking.. Aaha.. File is writable now.."); } else { crunchifyPrint("Checking.. Oops.. File is readonly :("); } } private static void crunchifyMakeWritable() { File crunchifyFile = new File("/Users/app/Download/crunchify.txt"); // setWritable() - A convenience method to set the owner's write permission for this abstract pathname. // On some platforms it may be possible to start the Java virtual machine with special privileges that allow it to modify files that disallow write operations. // An invocation of this method of the form file.setWritable(arg) behaves in exactly the same way as the invocation. // Let's make file Writable. boolean crunchifyCheck = crunchifyFile.setWritable(true); if (crunchifyCheck) { crunchifyPrint("File is writable now.."); } else { crunchifyPrint("Oops.. File is readonly.."); } } // Crunchify's simple Print utility. private static void crunchifyPrint(String s) { System.out.println(s); } }
Here is a list of all Java File() Operations:
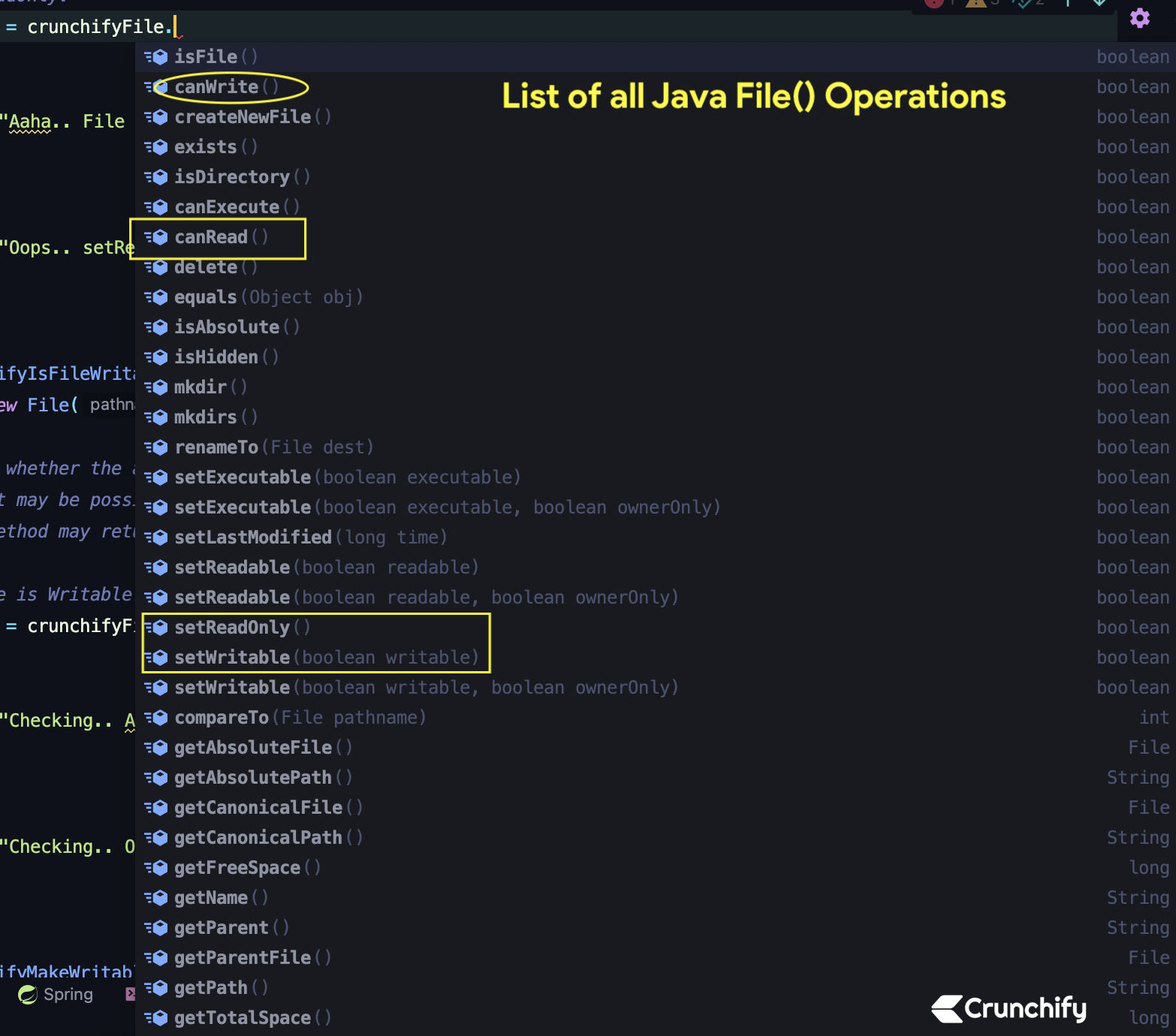
Just run above program as a Java Application and you will see IntelliJ console result as below.
Console result:
Aaha.. File is ReadOnly now.. Checking.. Oops.. File is readonly.. File is writable now.. Checking.. Aaha.. File is writable.. Process finished with exit code 0
Let me know if you face any issue running above Java Program.
Example-2.
Another simple Java program to make file readonly. This program creates a File object representing a file called “crunchify.json” in the current directory.
It first checks if the file exists, and if it does, it makes the file read-only using the setReadOnly()
method.
If the operation is successful, it prints “File is now read-only.” Otherwise, it prints “Failed to make file read-only.” Please note that depending on the file system, the readonly property may not be supported or may be ignored.
package crunchify.com.java.tutorials; import java.io.File; public class CrunchifyFileReadOnly { public static void main(String[] args) { File crunchifyFile = new File("/Users/app/Downloads/crunchify.json"); // Check if the crunchifyFile exists if (!crunchifyFile.exists()) { System.out.println("File does not exist."); return; } // Make the crunchifyFile read-only if (crunchifyFile.setReadOnly()) { System.out.println("File is now read-only."); } else { System.out.println("Failed to make crunchifyFile read-only."); } } }
IntelliJ IDEA console result
File is now read-only. Process finished with exit code 0
Let me know if you face any issue running this code.
The post In Java How to make a File ReadOnly or Writable? How to create new file? How to check if file is Writable or not? appeared first on Crunchify.
0 Commentaires