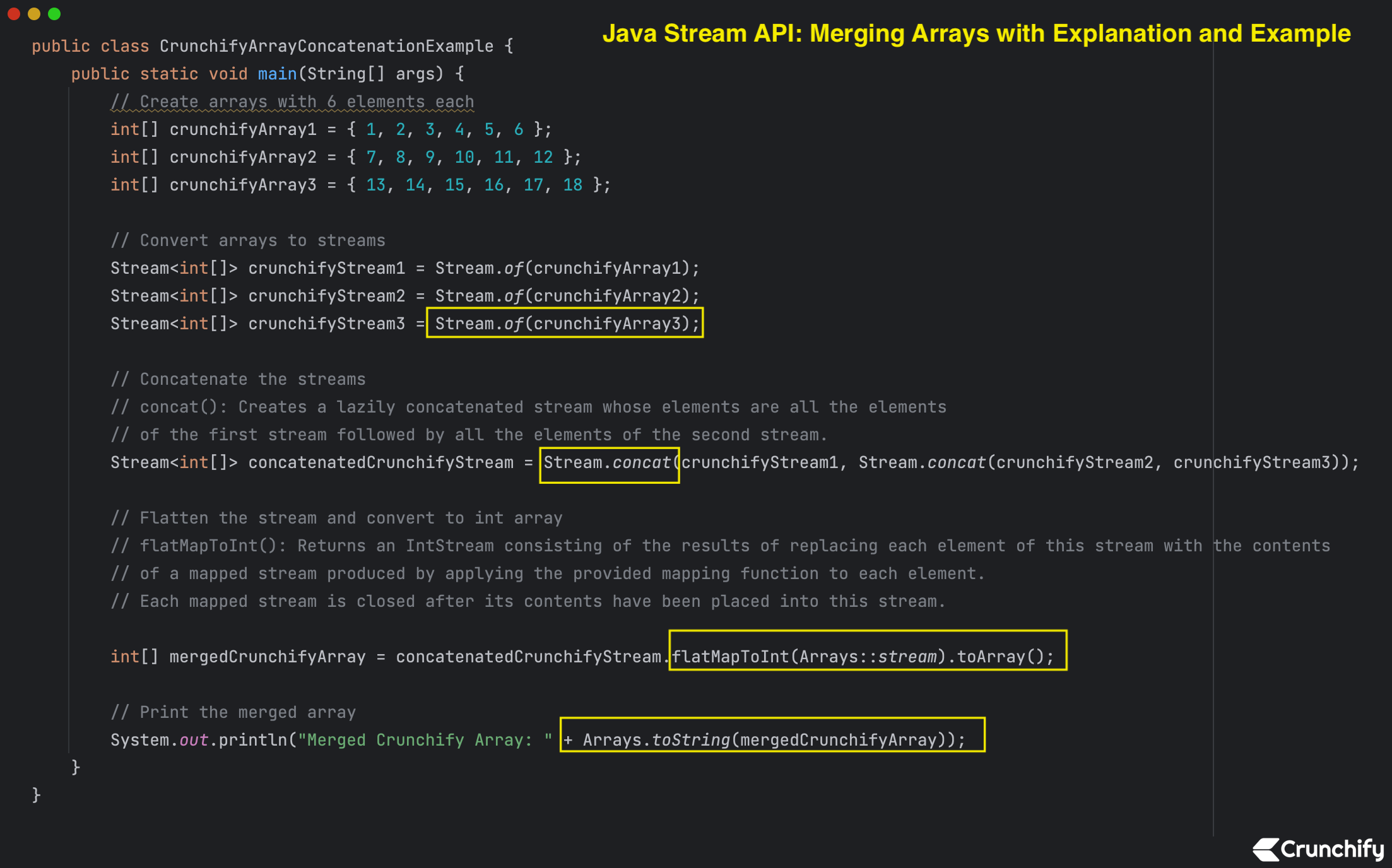
In Java programming, working with arrays is a common task.
However, there are situations where you need to merge
or concatenate
arrays to create a single, larger array. The Java Stream API
provides a concise and elegant way to achieve this while also enhancing code readability. In this blog post, we will explore how to merge arrays using the Java Stream API and provide a detailed example for better understanding.
Merging Arrays Using Java Stream API:
Merging arrays can be a cumbersome task, especially when dealing with multiple arrays. The Java Stream API simplifies this process by providing methods that enable seamless array concatenation.
Complete program:
CrunchifyArrayConcatenationExample.java
package crunchify.com.tutorial; import java.util.Arrays; import java.util.stream.Stream; /** * @author Crunchify.com * Java Stream API: Merging Arrays with Explanation and Example * */ public class CrunchifyArrayConcatenationExample { public static void main(String[] args) { // Create arrays with 6 elements each int[] crunchifyArray1 = { 1, 2, 3, 4, 5, 6 }; int[] crunchifyArray2 = { 7, 8, 9, 10, 11, 12 }; int[] crunchifyArray3 = { 13, 14, 15, 16, 17, 18 }; // Convert arrays to streams Stream<int[]> crunchifyStream1 = Stream.of(crunchifyArray1); Stream<int[]> crunchifyStream2 = Stream.of(crunchifyArray2); Stream<int[]> crunchifyStream3 = Stream.of(crunchifyArray3); // Concatenate the streams // concat(): Creates a lazily concatenated stream whose elements are all the elements // of the first stream followed by all the elements of the second stream. Stream<int[]> concatenatedCrunchifyStream = Stream.concat(crunchifyStream1, Stream.concat(crunchifyStream2, crunchifyStream3)); // Flatten the stream and convert to int array // flatMapToInt(): Returns an IntStream consisting of the results of replacing each element of this stream with the contents // of a mapped stream produced by applying the provided mapping function to each element. // Each mapped stream is closed after its contents have been placed into this stream. int[] mergedCrunchifyArray = concatenatedCrunchifyStream.flatMapToInt(Arrays::stream).toArray(); // Print the merged array System.out.println("Merged Crunchify Array: " + Arrays.toString(mergedCrunchifyArray)); } }
Eclipse Console Result:
Just run above program as a Java Application in Eclipse console or IntelliJ IDEA and you will see result as below.
Merged Crunchify Array: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18] Process finished with exit code 0
In this example, we start by creating three arrays, each containing 6 elements. We then use the Java Stream API to convert these arrays into streams using the Stream.of()
method.
Next, we employ the Stream.concat()
method to concatenate the streams sequentially, effectively merging the arrays.
To convert the merged stream back into an array, we use the flatMapToInt()
method followed by toArray()
. Finally, we print the merged array to the console.
The post Java Stream API: Merging Arrays with Explanation and Example appeared first on Crunchify.
0 Commentaires