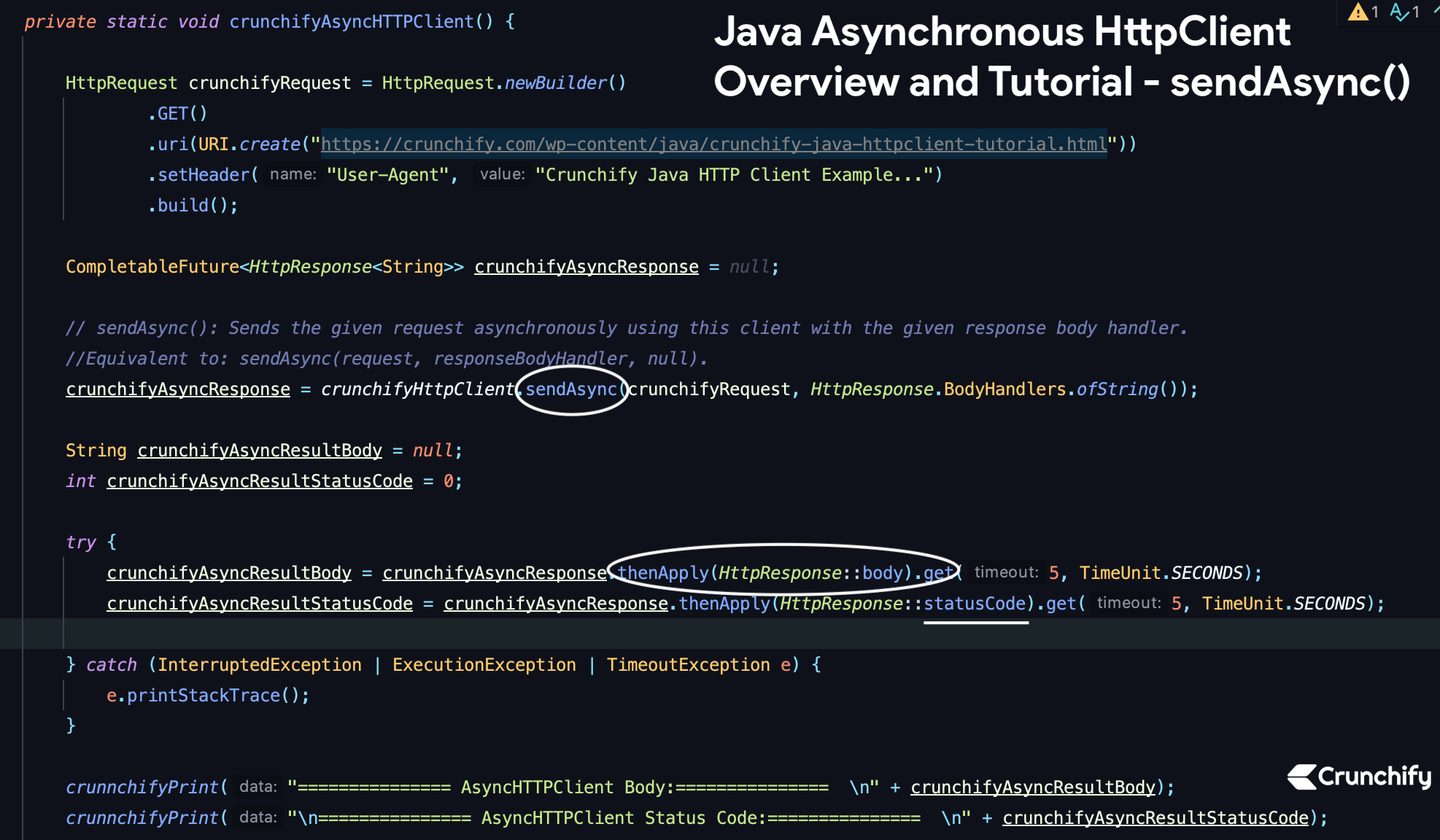
Java is very powerful. Every release brings so many new APIs and functionalities to core Java SDK. In this tutorial we will go over Java Asynchronous HttpClient Example and details.
Here is a tutorial on Java Synchronous HttpClient example. Java Asynchronous HttpClient Overview: sendAsync()
The Java Asynchronous HttpClient is a powerful tool for making HTTP requests asynchronously, allowing your application to handle multiple requests concurrently without blocking the main thread. In this blog post, we will explore the basics of using the sendAsync() method to make asynchronous HTTP requests using the Java Asynchronous HttpClient.
What is Asynchronous HttpClient?
Asynchronous HttpClient is a Java library that allows you to send HTTP requests asynchronously. It uses the CompletableFuture class to manage the asynchronous processing of HTTP requests, which allows your application to send multiple requests concurrently without blocking the main thread.
Using the sendAsync() Method
The sendAsync() method is the primary method for sending HTTP requests asynchronously with the Java Asynchronous HttpClient. It takes a HttpRequest object as its parameter and returns a CompletableFuture<HttpResponse<T>> object.
sendAsync()
sends the given request asynchronously using this client with the given response body handler.
Equivalent to: sendAsync(request, responseBodyHandler, null).
Let’s get started:
Create file CrunchifyJavaAsynchronousHTTPClient.java
package crunchify.com.tutorial; import java.net.URI; import java.net.http.HttpClient; import java.net.http.HttpRequest; import java.net.http.HttpResponse; import java.time.Duration; import java.util.concurrent.CompletableFuture; import java.util.concurrent.ExecutionException; import java.util.concurrent.TimeUnit; import java.util.concurrent.TimeoutException; /** * @author Crunchify.com * Overview and Simple Java Asynchronous HttpClient Client Tutorial */ public class CrunchifyJavaAsynchronousHTTPClient { private static final HttpClient crunchifyHttpClient = HttpClient.newBuilder() .version(HttpClient.Version.HTTP_1_1) .connectTimeout(Duration.ofSeconds(5)) .build(); // HttpClient: An HttpClient can be used to send requests and retrieve their responses. An HttpClient is created through a builder. // Duration: A time-based amount of time, such as '5 seconds'. public static void main(String[] args) { // Async HTTPClient Example crunchifyAsyncHTTPClient(); } private static void crunchifyAsyncHTTPClient() { HttpRequest crunchifyRequest = HttpRequest.newBuilder() .GET() .uri(URI.create("https://crunchify.com/wp-content/java/crunchify-java-httpclient-tutorial.html")) .setHeader("User-Agent", "Crunchify Java Aysnc HTTPClient Example...") .build(); CompletableFuture<HttpResponse<String>> crunchifyAsyncResponse = null; // sendAsync(): Sends the given request asynchronously using this client with the given response body handler. //Equivalent to: sendAsync(request, responseBodyHandler, null). crunchifyAsyncResponse = crunchifyHttpClient.sendAsync(crunchifyRequest, HttpResponse.BodyHandlers.ofString()); String crunchifyAsyncResultBody = null; int crunchifyAsyncResultStatusCode = 0; try { crunchifyAsyncResultBody = crunchifyAsyncResponse.thenApply(HttpResponse::body).get(5, TimeUnit.SECONDS); // OR: // join(): Returns the result value when complete, or throws an (unchecked) exception if completed exceptionally. // To better conform with the use of common functional forms, // if a computation involved in the completion of this CompletableFuture threw an exception, // this method throws an (unchecked) CompletionException with the underlying exception as its cause. HttpResponse<String> response = crunchifyAsyncResponse.join(); crunchifyAsyncResultStatusCode = crunchifyAsyncResponse.thenApply(HttpResponse::statusCode).get(5, TimeUnit.SECONDS); } catch (InterruptedException | ExecutionException | TimeoutException e) { e.printStackTrace(); } crunnchifyPrint("=============== AsyncHTTPClient Body:=============== \n" + crunchifyAsyncResultBody); crunnchifyPrint("\n=============== AsyncHTTPClient Status Code:=============== \n" + crunchifyAsyncResultStatusCode); } private static void crunnchifyPrint(Object data) { System.out.println(data); } }
In this example, we first create a new HttpClient object and then create an HttpRequest object using the HttpRequest.newBuilder()
method. We set the URI of the request to “https://ift.tt/N6EDoOP; using the uri() method.
We then call the sendAsync() method on the HttpClient object, passing in the HttpRequest object and an instance of the HttpResponse.BodyHandlers class. The HttpResponse.BodyHandlers.ofString() method returns a BodyHandler that converts the response body to a String.
The sendAsync() method returns a CompletableFuture<HttpResponse<T>> object, which we store in the futureResponse variable. We can then do other things while the request is being processed asynchronously.
Finally, we use the join() method to wait for the response to complete and store it in the response variable. We can then access the response body using the body() method and print it to the console.
Simply run above program and you will see below result.
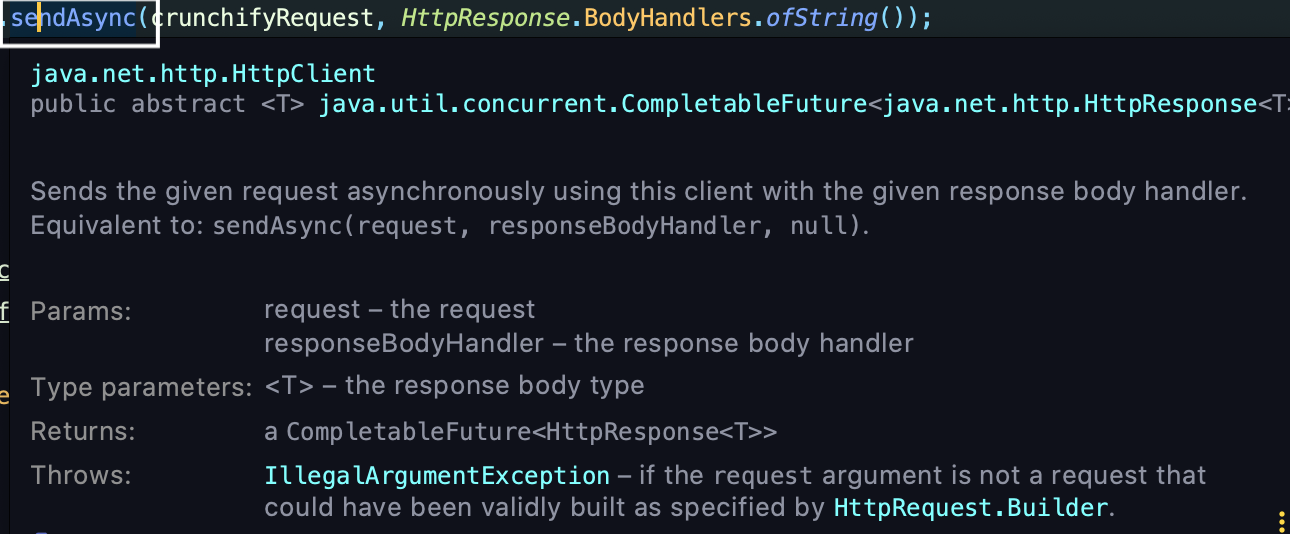
IntelliJ IDEA Result:
=============== AsyncHTTPClient Body:=============== <!DOCTYPE html> <html> <body> <h1>Hey.. This is Crunchify's Java HTTPClient Tutorial. </h1> <br>Tutorial link: <a href="https://crunchify.com/overview-and-simple-java-14-synchronous-httpclient-client-tutorial/">https://crunchify.com/overview-and-simple-java-14-synchronous-httpclient-client-tutorial/</a> </body> </html> =============== AsyncHTTPClient Status Code:=============== 200 Process finished with exit code 0
Let me know if you face any issue running this program.
What’s next?
The post Java Asynchronous HttpClient Overview and Tutorial – sendAsync() appeared first on Crunchify.
0 Commentaires