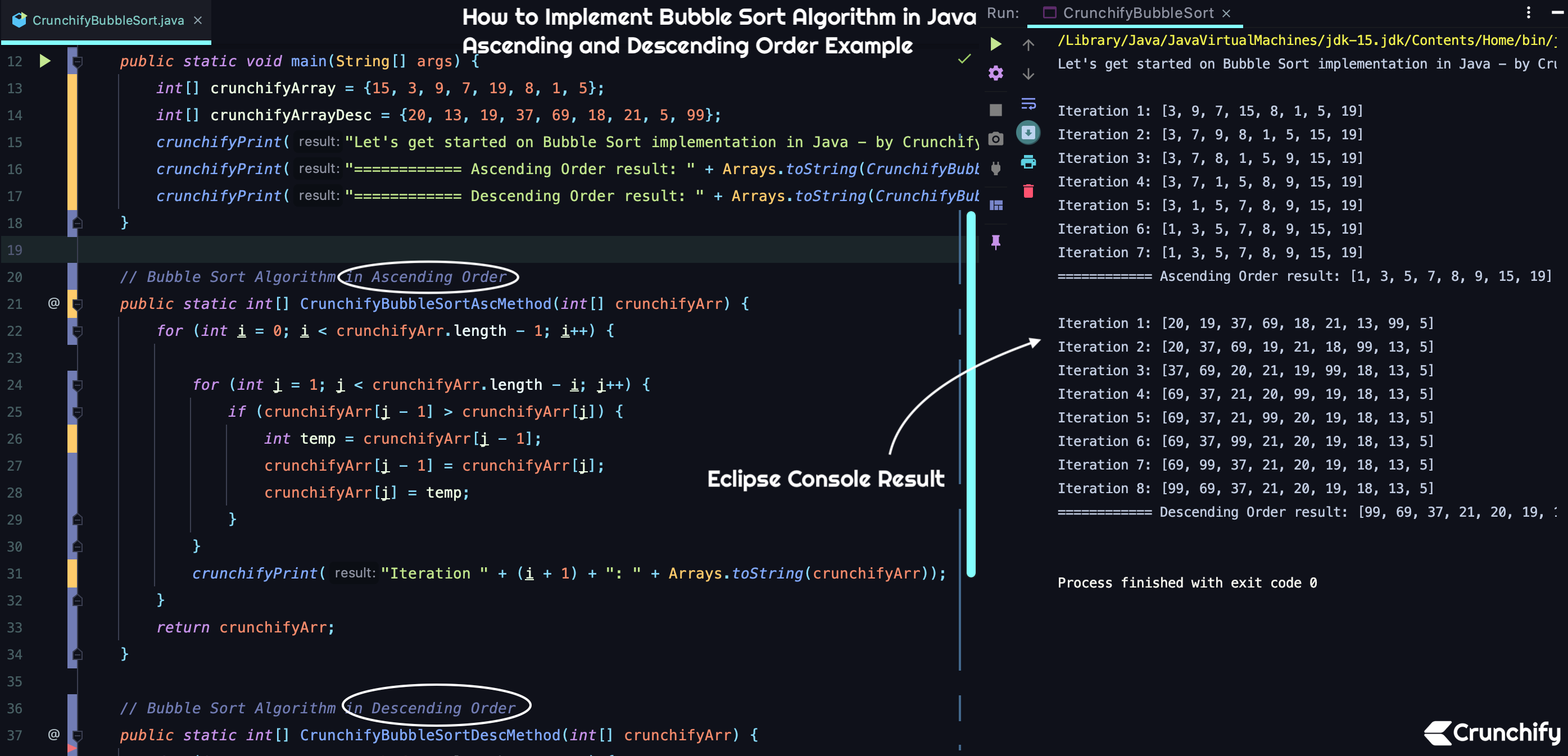
Bubble sort
, sometimes incorrectly referred to as sinking sort
, is a simple sorting algorithm that works by repeatedly stepping through the list to be sorted, comparing each pair of adjacent items and swapping them if they are in the wrong order.
The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted. The algorithm gets its name from the way smaller elements bubble
to the top of the list.
Because it only uses comparisons to operate on elements, it is a comparison sort. Although the algorithm is simple, most of the other sorting algorithms are more efficient for large lists.
Logic is Simple:
In bubble sort, we basically traverse the arraylist from first to (size – 1) position and compare the element with the next one. Swap Element with the next element only if the next element is greater.
Here is a Java Code:
- Create file
CrunchifyBubbleSort.java
.
package crunchify.com.tutorials; import java.util.Arrays; /** * @author Crunchify.com * How to Implement Bubble sort algorithm in Java? Ascending and Descending Order Tutorial */ public class CrunchifyBubbleSort { public static void main(String[] args) { int[] crunchifyArray = {15, 3, 9, 7, 19, 8, 1, 5}; int[] crunchifyArrayDesc = {20, 13, 19, 37, 69, 18, 21, 5, 99}; crunchifyPrint("Let's get started on Bubble Sort implementation in Java - by Crunchify \n"); crunchifyPrint("============ Ascending Order result: " + Arrays.toString(CrunchifyBubbleSortAscMethod(crunchifyArray)) + "\n"); crunchifyPrint("============ Descending Order result: " + Arrays.toString(CrunchifyBubbleSortDescMethod(crunchifyArrayDesc)) + "\n"); } // Bubble Sort Algorithm in Ascending Order public static int[] CrunchifyBubbleSortAscMethod(int[] crunchifyArr) { for (int i = 0; i < crunchifyArr.length - 1; i++) { for (int j = 1; j < crunchifyArr.length - i; j++) { if (crunchifyArr[j - 1] > crunchifyArr[j]) { int temp = crunchifyArr[j - 1]; crunchifyArr[j - 1] = crunchifyArr[j]; crunchifyArr[j] = temp; } } crunchifyPrint("Iteration " + (i + 1) + ": " + Arrays.toString(crunchifyArr)); } return crunchifyArr; } // Bubble Sort Algorithm in Descending Order public static int[] CrunchifyBubbleSortDescMethod(int[] crunchifyArr) { for (int i = 0; i < crunchifyArr.length - 1; i++) { for (int j = 1; j < crunchifyArr.length - i; j++) { if (crunchifyArr[j - 1] < crunchifyArr[j]) { int temp = crunchifyArr[j - 1]; crunchifyArr[j - 1] = crunchifyArr[j]; crunchifyArr[j] = temp; } } crunchifyPrint("Iteration " + (i + 1) + ": " + Arrays.toString(crunchifyArr)); } return crunchifyArr; } // Simple log util private static void crunchifyPrint(String result) { System.out.println(result); } }
Eclipse Console Result:
Just run above Bubble Sort java program in Eclipse console or IntelliJ IDE and you should see result as below.
Let's get started on Bubble Sort implementation in Java - by Crunchify Iteration 1: [3, 9, 7, 15, 8, 1, 5, 19] Iteration 2: [3, 7, 9, 8, 1, 5, 15, 19] Iteration 3: [3, 7, 8, 1, 5, 9, 15, 19] Iteration 4: [3, 7, 1, 5, 8, 9, 15, 19] Iteration 5: [3, 1, 5, 7, 8, 9, 15, 19] Iteration 6: [1, 3, 5, 7, 8, 9, 15, 19] Iteration 7: [1, 3, 5, 7, 8, 9, 15, 19] ============ Ascending Order result: [1, 3, 5, 7, 8, 9, 15, 19] Iteration 1: [20, 19, 37, 69, 18, 21, 13, 99, 5] Iteration 2: [20, 37, 69, 19, 21, 18, 99, 13, 5] Iteration 3: [37, 69, 20, 21, 19, 99, 18, 13, 5] Iteration 4: [69, 37, 21, 20, 99, 19, 18, 13, 5] Iteration 5: [69, 37, 21, 99, 20, 19, 18, 13, 5] Iteration 6: [69, 37, 99, 21, 20, 19, 18, 13, 5] Iteration 7: [69, 99, 37, 21, 20, 19, 18, 13, 5] Iteration 8: [99, 69, 37, 21, 20, 19, 18, 13, 5] ============ Descending Order result: [99, 69, 37, 21, 20, 19, 18, 13, 5] Process finished with exit code 0
What is a Time Complexity of Bubble Sort Algorithm?
- If you consider
Best case
scenario then it would beO(n)
- If you consider
Worst case
scenario then it would beO(n2)
Let me know if you have any issue or exception running above program.
The post How to Implement Bubble Sort Algorithm in Java – Ascending and Descending Order Example appeared first on Crunchify.
0 Commentaires