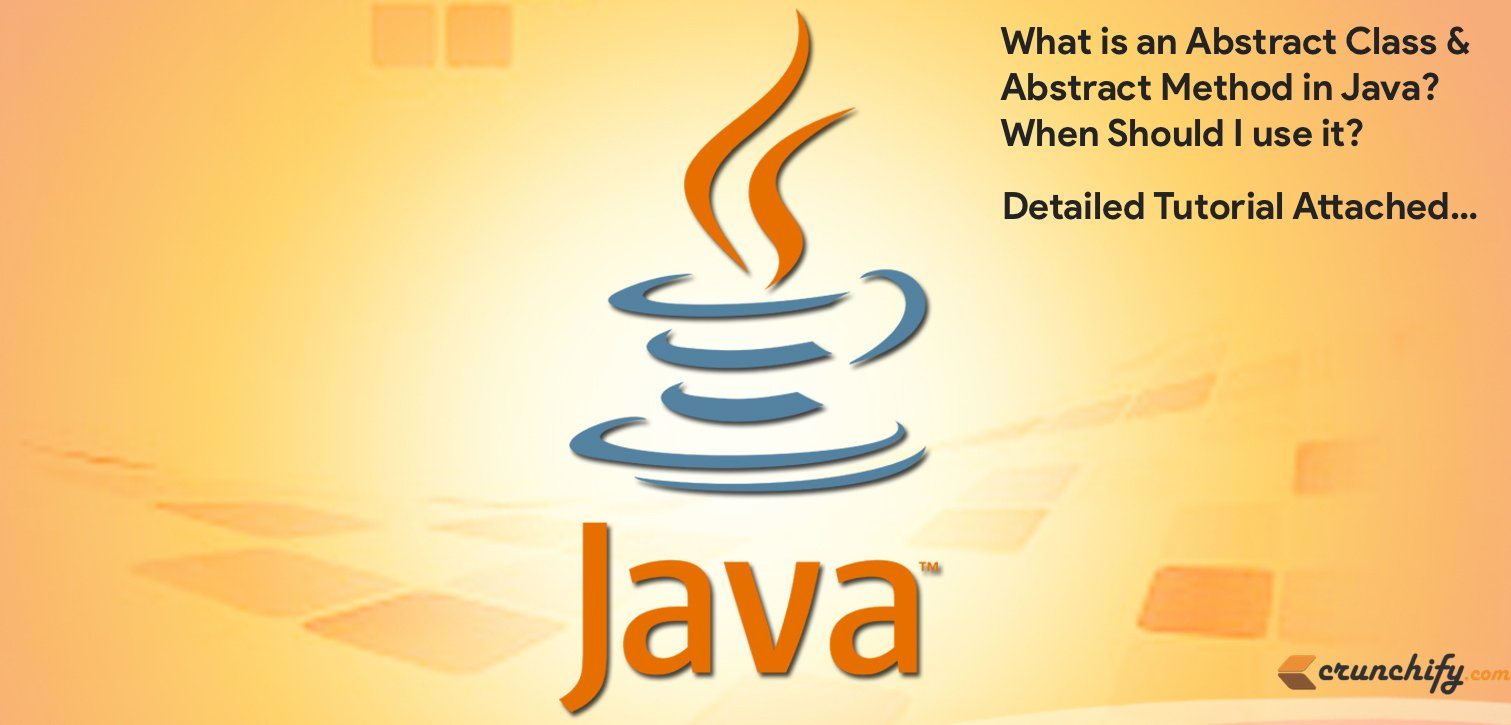
Couple of days back I wrote an article on basic Java Fundamental on What is an Interface in Java and How it’s used? This tutorial is also related to basic Java fundamental “Abstract Class and Abstract Method
“.
What is an Abstract Class?
Let’s start understanding Abstract class first and then we will go over Example.
- An abstract class is a class that is declared
abstract
- Abstract classes cannot be instantiated
- Abstract classes can be subclassed
- It may or may not include abstract methods
- When an abstract class is
subclassed
, the subclass usually provides implementations for all of the abstract methods in its parent class - If subclass doesn’t provide implementations then the subclass must also be declared
abstract
.
This is a very basic Java Interview Question
. Probably the 1st Java Interview Question
you get during interview.
Can I define an abstract class without adding an abstract method?
Of course yes. Declaring a class abstract only means that you don’t allow it to be instantiated on its own. You can’t have an abstract method in a non-abstract class.
What is an Abstract Method?
- An
abstract method
is a method that is declared without an implementation. - It just has a method signature.
package crunchify.com.tutorial; /** * @author Crunchify.com * Simple Abstract Class and Method Example with live result * */ public abstract class CrunchifyExam { public enum ExamStatus { PASSED, FAILED } private String examTime; private ExamStatus status; public CrunchifyExam(String examTime, ExamStatus status) { this.examTime = examTime; this.status = status; } public String getExamTime() { return examTime; } public void setExamTime(String examTime) { this.examTime = examTime; } public void setExamStatus(ExamStatus status) { this.status = status; } public ExamStatus getExamStatus() { return status; } abstract public void checkResult(); }
Let’s start with an Example. Problem Description:
- Create class
CrunchifyExam.java
, which has one abstract method calledcheckResult()
- Create class
Crunchify1stSchoolExamResult.java
, which extends Abstract classCrunchifyExam.java
- Create class
Crunchify2ndSchoolExamResult.java
, which extends Abstract classCrunchifyExam.java
- Now both above classes have to provide implementation for checkResult() method
- Both Schools may have their own different
procedure
ornumber of checks
to find out if user isPASSED or FAILED
, they are free to have their own implementation ofcheckResult()
Once you extend CrunchifyExam
class in Crunchify1stSchoolExamResult.java
– Eclipse will prompt you to implement Abstract methods mentioned in CrunchifyExam.java
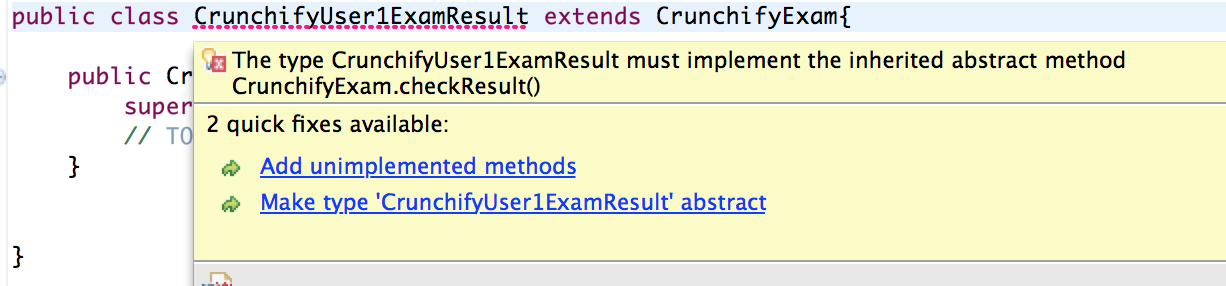
Crunchify1stSchoolExamResult.java
package crunchify.com.tutorial; /** * @author Crunchify.com */ public class Crunchify1stSchoolExamResult extends CrunchifyExam { public Crunchify1stSchoolExamResult(String examTime, ExamStatus status) { super(examTime, status); // TODO Auto-generated constructor stub } @Override public void checkResult() { String studentName = "Crunchify1"; String studentResult = "85%"; // School NO-1 will provide all their formula to find if user is passed or failed. // After detailed calculation let's say student's grade is "PASSED". System.out.println("Hey.. this is user " + studentName + " with grade " + studentResult + " - " + getExamStatus() + ", ExamTime: " + getExamTime()); } }
Crunchify2ndSchoolExamResult.java
package crunchify.com.tutorial; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; /** * @author Crunchify.com */ public class Crunchify2ndSchoolExamResult extends CrunchifyExam { public Crunchify2ndSchoolExamResult(String examTime, ExamStatus status) { super(examTime, status); } @Override public void checkResult() { String studentName = "Crunchify2"; String studentResult = "45%"; // School NO-2 will provide all their formula to find if user is passed or failed. // After detailed calculation let's say student's grade is "FAILED". log("Hey.. this is user " + studentName + " with grade " + studentResult + " - " + getExamStatus() + ", ExamTime: " + getExamTime()); } public static void main(String args[]) { DateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss"); // 1st School's checkResult() Date date = new Date(); String examTime = dateFormat.format(date); log("Initializing 1st School object at time " + examTime); // We are setting up time and Result for 1st School Crunchify1stSchoolExamResult object = new Crunchify1stSchoolExamResult(examTime, ExamStatus.PASSED); object.checkResult(); // Let's wait 5 seconds wait to see time difference in console log try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } // 2nd School's checkResult() date = new Date(); examTime = dateFormat.format(date); log("\nInitializing 2nd School object at time " + examTime); // We are setting up time and Result for 2nd School Crunchify2ndSchoolExamResult object2 = new Crunchify2ndSchoolExamResult(examTime, ExamStatus.FAILED); object2.checkResult(); } // Simple log method private static void log(String value) { System.out.println(value); } }
Eclipse Console Result:
Just right click on Crunchify2ndSchoolExamResult.java
and run as Java Application
to see below result.
Initializing 1st School object at time 2016/11/30 14:24:37 Hey.. this is user Crunchify1 with grade 85% - PASSED ExamTime: 2016/11/30 14:24:37 Initializing 2nd School object at time 2016/11/30 14:24:42 Hey.. this is user Crunchify2 with grade 45% - FAILED ExamTime: 2016/11/30 14:24:42
Now you may have a question
Why can’t I use Interface here rather than having Abstract Method
and Class
and have CrunchifyExam as an Interface?
Well – Sure you could
– but you’d also need to implement the getExamTime
(), setExamTime
(), getExamTime
(), setExamTime
() methods.
By using abstract classes, you can inherit the implementation of other (non-abstract) methods. You can’t do that with interfaces – an interface cannot provide
any method implementations.
Is it possible to create abstract and final class in Java?
- The abstract and final are the mutually exclusive concept.
- So answer is NO, we can’t make an abstract class or method final in Java.
- Final class is complete class and can’t be extended further.
- Abstract class is called incomplete class and can be only extended by other concrete class and you have to implement all abstract methods.
- Also, there is no way and it’s not possible to have an abstract method in a final class.
Is it possible to inherit from multiple abstract classes in Java?
- Java does not support multiple inheritance.
- In java we can only Extend a single class.
- In Java we can implement Interfaces from many sources.
The post What is an Abstract Class and Abstract Method in Java? When Should I use it? Tutorial Attached appeared first on Crunchify.
0 Commentaires