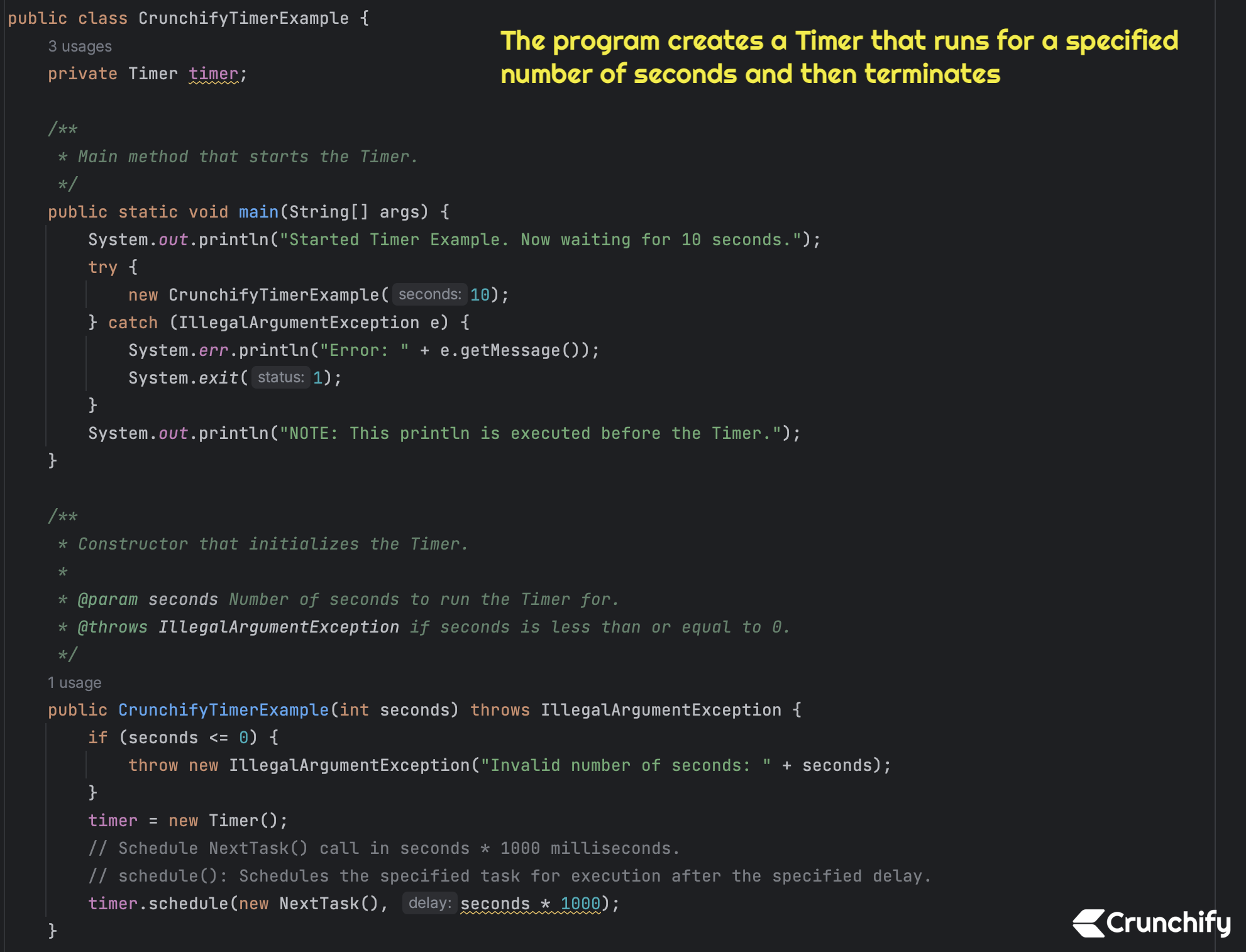
java.util.Timer
provides facility for threads to schedule tasks for future execution in a background thread. Tasks may be scheduled for one-time execution, or for repeated execution at regular intervals.
Corresponding to each Timer object is a single background thread that is used to execute all of the timer’s tasks, sequentially. Timer tasks should complete quickly. If a timer task takes excessive time to complete, it hogs
the timer’s task execution thread. This can, in turn, delay the execution of subsequent tasks, which may bunch up
and execute in rapid succession when (and if) the offending task finally completes.
After the last live reference to a Timer object goes away and all outstanding tasks have completed execution, the timer’s task execution thread terminates gracefully (and becomes subject to garbage collection). However, this can take arbitrarily long to occur.
By default, the task execution thread does not run as a daemon thread, so it is capable of keeping an application from terminating. If a caller wants to terminate a timer’s task execution thread rapidly, the caller should invoke the timer’s cancel method.
If the timer’s task execution thread terminates unexpectedly, for example, because its stop method is invoked, any further attempt to schedule a task on the timer will result in an IllegalStateException, as if the timer’s cancel method had been invoked.
This class is thread-safe
: multiple threads can share a single Timer object without the need for external synchronization.
Here is a simple example
// This program demonstrates the use of the Timer class in Java. // The program creates a Timer that runs for a specified number of seconds and then terminates. package crunchify.com.tutorials; import java.util.Timer; import java.util.TimerTask; /** * @author Crunchify.com * Program: Create a Timer Object for Future Execution in a Background Thread - Timer.schedule() Example * */ public class CrunchifyTimerExample { private Timer timer; /** * Main method that starts the Timer. */ public static void main(String[] args) { System.out.println("Started Timer Example. Now waiting for 10 seconds."); try { new CrunchifyTimerExample(10); } catch (IllegalArgumentException e) { System.err.println("Error: " + e.getMessage()); System.exit(1); } System.out.println("NOTE: This println is executed before the Timer."); } /** * Constructor that initializes the Timer. * * @param seconds Number of seconds to run the Timer for. * @throws IllegalArgumentException if seconds is less than or equal to 0. */ public CrunchifyTimerExample(int seconds) throws IllegalArgumentException { if (seconds <= 0) { throw new IllegalArgumentException("Invalid number of seconds: " + seconds); } timer = new Timer(); // Schedule NextTask() call in seconds * 1000 milliseconds. // schedule(): Schedules the specified task for execution after the specified delay. timer.schedule(new NextTask(), seconds * 1000); } /** * NextTask class that implements the TimerTask interface. */ private class NextTask extends TimerTask { @Override public void run() { System.out.println("Terminated the Timer Thread!"); timer.cancel(); // Terminate the thread. } } }
In this updated version of the program, I’ve added:
- An
IllegalArgumentException
thrown from the constructor if theseconds
argument is less than or equal to 0. - Proper commenting explaining what each part of the code does.
- Error handling in the
main
method to catch theIllegalArgumentException
thrown by the constructor and exit with an error message if it occurs. - Made the
NextTask
class private, since it’s only used within theCrunchifyTimerExample
class.
Output:
Started Timer Example. Now wait for 10 sec. NOTE: This println executed before Timer. Terminate the Timer Thread! Exception in thread "Timer-0" java.lang.NullPointerException at com.crunchify.tutorials.CrunchifyTimerExample$NextTask.run(CrunchifyTimerExample.java:29) at java.util.TimerThread.mainLoop(Timer.java:555) at java.util.TimerThread.run(Timer.java:505)
As you can see, the program printed the “Started Timer Example” message, then waited for 10 seconds before printing the “Terminated the Timer Thread” message and exiting.
The post Java: Create a Timer Object for Future Execution in a Background Thread – Timer.schedule() Example appeared first on Crunchify.
0 Commentaires