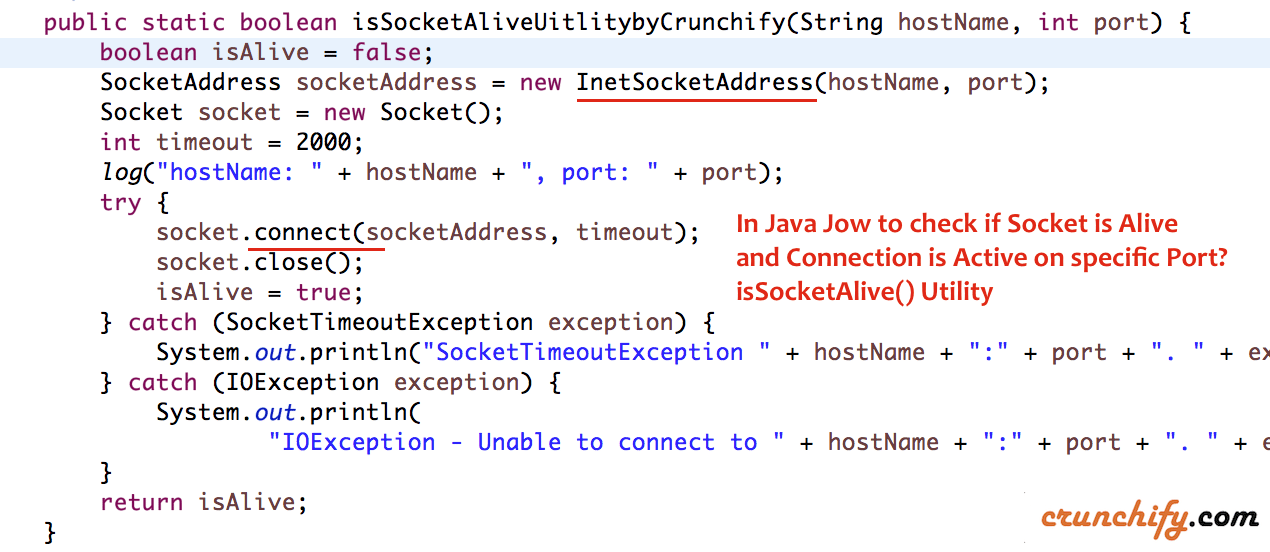
This is a very simple free Java Utility which tells you if you could establish a socket connection to different hosts on specific ports. Feel free to use it in production environment.
In Java InetSocketAddress
creates a socket address from a hostname
and a port number
. We call it as server socket in Java. Socket library with Non blocking IO
and Simple IO
is a very big topic.
In this tutorial we will go over simple utility which we are calling isSocketAlive
(String hostname, int port) which returns boolean
result – true/false.
Let’s get started:
Step-1
Create class CrunchifyIsSocketAliveUtility
.java
Step-2
Create utility isSocketAliveUitlitybyCrunchify
(hostname, port) which returns true/false
Step-3
In main method, we will do 5 tests
- Start Apache Tomcat Server in Eclipse IDE and try to connect to
localhost
on port8080
- Install Apache Tomcat in Eclipse Tutorial link
- Now connect to port
8081
which will be a failure scenario - We will also connect to site
crunchify.com
on port80
. As Crunchify.com runs on Apache Server by default port is 80. - We will connect to site pro.
crunchify.com
on port80
–> Success - Connect to site pro.
crunchify.com and 81
–> It will be failure
Provide default timeout to 2 seconds.
socket.connect
(socketAddress, timeout) connects socket to the server with a specified timeout value.
A timeout of zero
is interpreted as an infinite timeout. The connection will then block until established or an error occurs.
We are catching SocketTimeException
and IOException
in case of error.
This Java Program will help you in case you have below questions:
- Java socket programming tutorial
- Writing the Server Side of a Socket
- Sockets programming in Java
- java socket connection example
- Java client/server application with sockets
- java serversocket example
- java.net.socket example
- listen socket connection java example
Complete Java Program:
package crunchify.com.tutorials; import java.io.IOException; import java.net.InetSocketAddress; import java.net.Socket; import java.net.SocketAddress; import java.net.SocketTimeoutException; /** * @author Crunchify.com * */ public class CrunchifyIsSocketAliveUtility { public static void main(String[] args) { // Run Apache Tomcat server on Port 8080 in Eclipse to see success // result log(isSocketAliveUitlitybyCrunchify("localhost", 8080)); // Now change port to 8081 and you should see failure log(isSocketAliveUitlitybyCrunchify("localhost", 8081)); // Connect to Crunchify.com on port 80 log(isSocketAliveUitlitybyCrunchify("crunchify.com", 80)); // Connection to pro.crunchify.com on port 81 log(isSocketAliveUitlitybyCrunchify("pro.crunchify.com", 81)); // Connection to pro.crunchify.com on port 80 log(isSocketAliveUitlitybyCrunchify("pro.crunchify.com", 80)); } /** * Crunchify's isAlive Utility * * @param hostName * @param port * @return boolean - true/false */ public static boolean isSocketAliveUitlitybyCrunchify(String hostName, int port) { boolean isAlive = false; // Creates a socket address from a hostname and a port number SocketAddress socketAddress = new InetSocketAddress(hostName, port); Socket socket = new Socket(); // Timeout required - it's in milliseconds int timeout = 2000; log("hostName: " + hostName + ", port: " + port); try { socket.connect(socketAddress, timeout); socket.close(); isAlive = true; } catch (SocketTimeoutException exception) { System.out.println("SocketTimeoutException " + hostName + ":" + port + ". " + exception.getMessage()); } catch (IOException exception) { System.out.println( "IOException - Unable to connect to " + hostName + ":" + port + ". " + exception.getMessage()); } return isAlive; } // Simple log utility private static void log(String string) { System.out.println(string); } // Simple log utility returns boolean result private static void log(boolean isAlive) { System.out.println("isAlive result: " + isAlive + "\n"); } }
Output:
hostName: localhost, port: 8080 isAlive result: true hostName: localhost, port: 8081 IOException - Unable to connect to localhost:8081. Connection refused isAlive result: false hostName: crunchify.com, port: 80 isAlive result: true hostName: pro.crunchify.com, port: 81 SocketTimeoutException pro.crunchify.com:81. connect timed out isAlive result: false hostName: pro.crunchify.com, port: 80 isAlive result: true
The post In Java how to check if Socket is Alive, Connection is Active on specific Port? isSocketAlive() Utility appeared first on Crunchify.
0 Commentaires