What is Java Collections Framework? Benefits of Collections Framework?
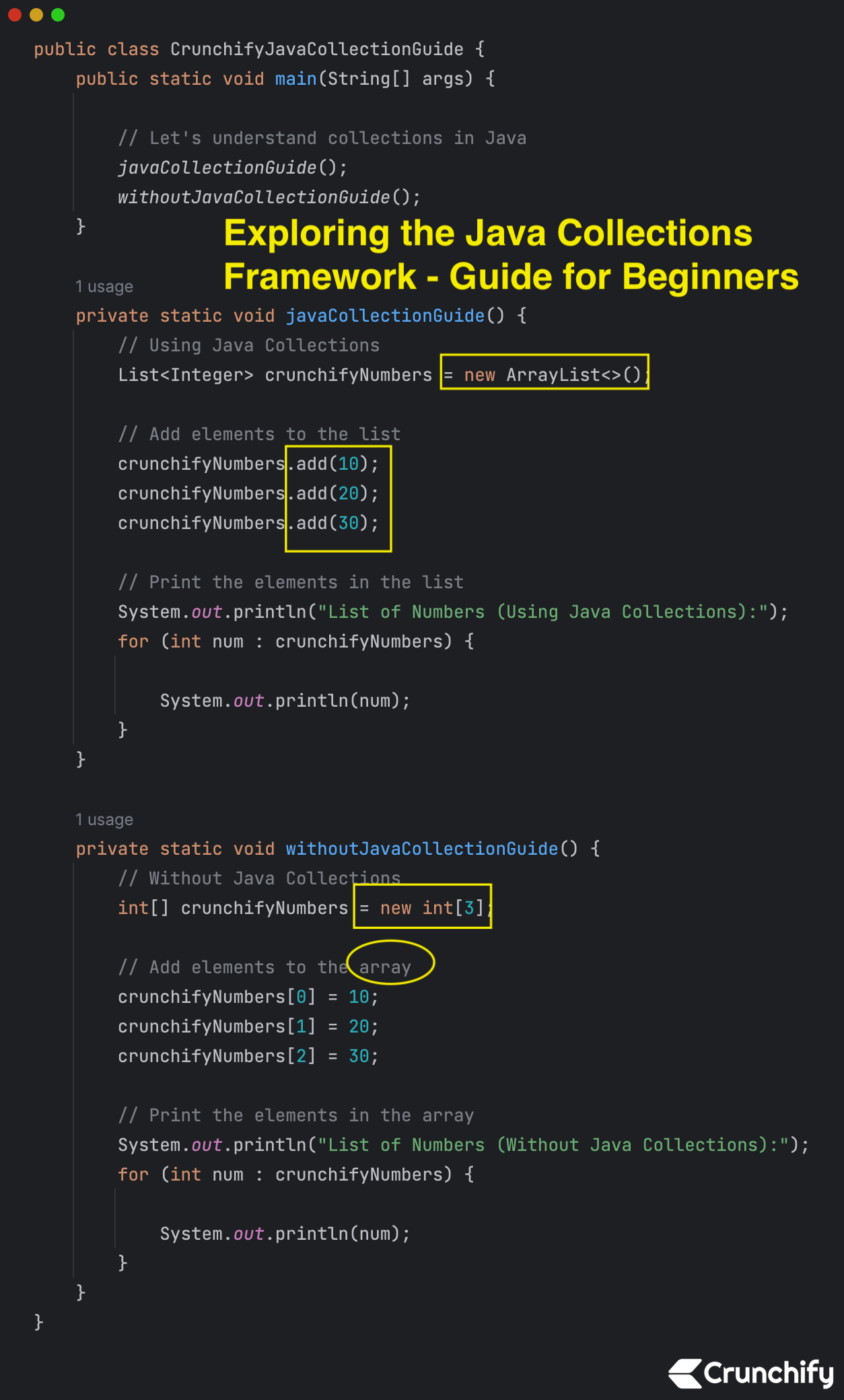
Java Collections are used in every programming language and initial java release contained few classes for collections: Vector, Stack, Hashtable, Array. But looking at the larger scope and usage, Java 1.2 came up with Collections Framework that group all the collections interfaces, implementations and algorithms.
Java Collections have come through a long way with usage of Generics and Concurrent Collection classes for thread-safe operations. It also includes blocking interfaces and their implementations in java concurrent package.
Key Components of Java Collections Framework:
- Interfaces: The Collections Framework consists of several core interfaces, including:
- List: Ordered collection with duplicate elements allowed (e.g., ArrayList, LinkedList).
- Set: Unordered collection with no duplicate elements (e.g., HashSet, TreeSet).
- Map: Key-value pairs where keys are unique (e.g., HashMap, TreeMap).
- Queue: A collection designed for holding elements prior to processing (e.g., LinkedList, PriorityQueue).
- Classes: These are concrete implementations of the collection interfaces, providing various functionalities and optimizations.
Benefits of Java Collections Framework:
- Reusability and Consistency: The framework promotes code reusability by providing a common set of interfaces and methods. This consistency allows developers to easily switch between different implementations without changing the overall code structure.
- Performance: Java Collections Framework offers optimized data structures that are designed for specific use cases. For example, LinkedList is efficient for insertions and deletions, while ArrayList provides fast random access. This performance optimization can significantly enhance the efficiency of your code.
- Readability and Maintainability: Using the Collections Framework leads to cleaner and more organized code. The intuitive naming conventions and consistent methods make it easier for developers to understand and maintain the codebase.
- Type Safety: The framework employs generics, allowing you to specify the type of elements a collection can hold. This enforces type safety and reduces the likelihood of runtime errors.
- Concurrency Support: Java Collections Framework provides concurrent collections that enable safe manipulation of data in multithreaded environments. ConcurrentHashMap and ConcurrentLinkedQueue are examples of classes that support concurrent access.
Complete Java Example
- CrunchifyJavaCollectionGuide.java
package crunchify.com.tutorials; import java.util.ArrayList; import java.util.List; /** * @author Crunchify * Exploring the Java Collections Framework: A Comprehensive Guide for Beginners * */ public class CrunchifyJavaCollectionGuide { public static void main(String[] args) { // Let's understand collections in Java javaCollectionGuide(); withoutJavaCollectionGuide(); } private static void javaCollectionGuide() { // Using Java Collections List<Integer> crunchifyNumbers = new ArrayList<>(); // Add elements to the list crunchifyNumbers.add(10); crunchifyNumbers.add(20); crunchifyNumbers.add(30); // Print the elements in the list System.out.println("List of Numbers (Using Java Collections):"); for (int num : crunchifyNumbers) { System.out.println(num); } } private static void withoutJavaCollectionGuide() { // Without Java Collections int[] crunchifyNumbers = new int[3]; // Add elements to the array crunchifyNumbers[0] = 10; crunchifyNumbers[1] = 20; crunchifyNumbers[2] = 30; // Print the elements in the array System.out.println("List of Numbers (Without Java Collections):"); for (int num : crunchifyNumbers) { System.out.println(num); } } }
Both examples achieve the same result of storing and printing a list of numbers, but the first example uses the Java Collections Framework to manage the list with more flexibility and built-in features. The second example manually handles an array without the benefits provided by the Collections Framework, such as dynamic resizing and various collection operations.
Remember, using Java Collections often results in more efficient and maintainable code, especially for complex data structures and operations.
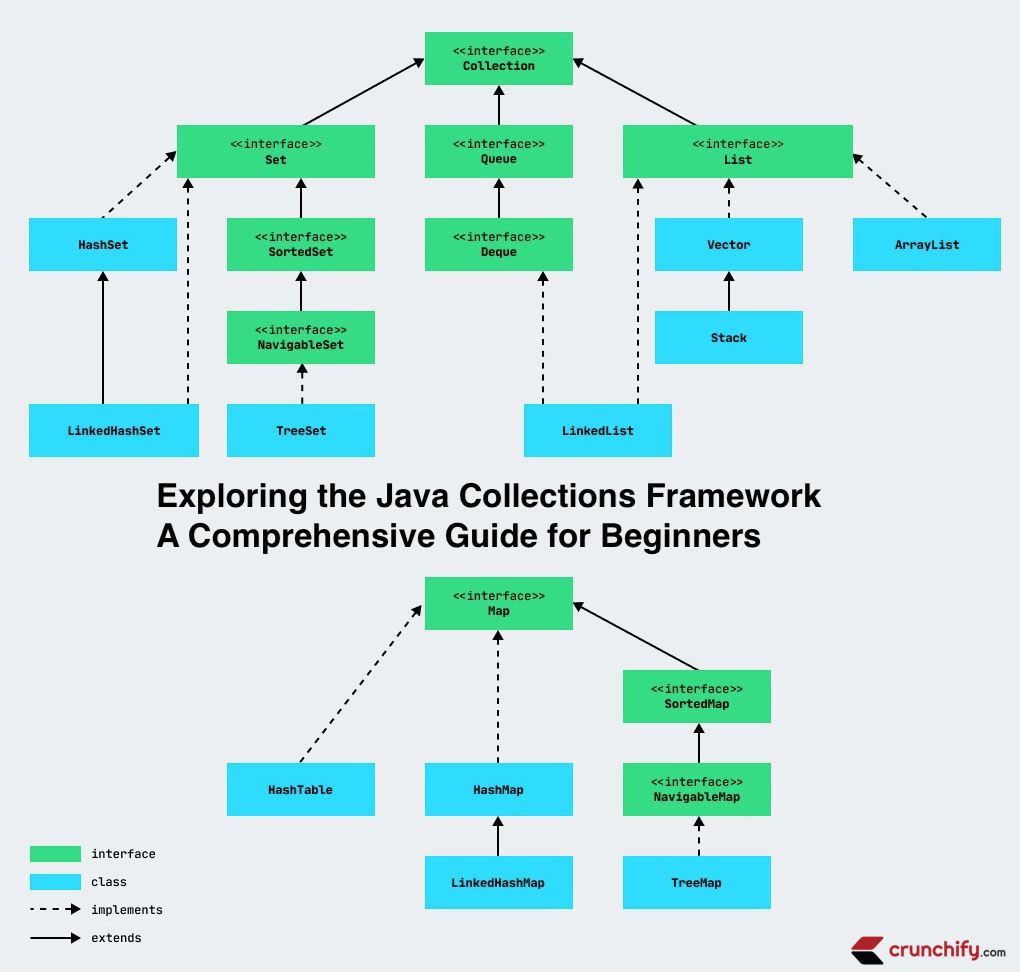
What is the benefit of Generics in Collections Framework?
Java 1.5 came with Generics and all collection interfaces and implementations use it heavily. Generics allow us to provide the type of Object that a collection can contain, so if you try to add any element of other type it throws compile time error.
This avoids ClassCastException at Runtime because you will get the error at compilation. Also Generics make code clean since we don’t need to use casting and instanceof operator. It also adds up to runtime benefit because the bytecode instructions that do type checking are not generated.
What are the basic interfaces of Java Collections Framework?
Collection is the root of the collection hierarchy. A collection represents a group of objects known as its elements. The Java platform doesn’t provide any direct implementations of this interface.
- Set is a collection that cannot contain duplicate elements. This interface models the mathematical set abstraction and is used to represent sets, such as the deck of cards.
- List is an ordered collection and can contain duplicate elements. You can access any element from it’s index. List is more like array with dynamic length.
- Map is an object that maps keys to values. A map cannot contain duplicate keys: Each key can map to at most one value.
Some other interfaces are Queue, Dequeue, Iterator, SortedSet, SortedMap and ListIterator.
The post Exploring the Java Collections Framework: A Comprehensive Guide for Beginners appeared first on Crunchify.
0 Commentaires