In Java how to check if a file is hidden? Explore a Java program that checks whether a file is hidden or not, using logging to track its status. Learn how to set up logging configurations, handle file existence, and interpret log messages for effective debugging and monitoring.
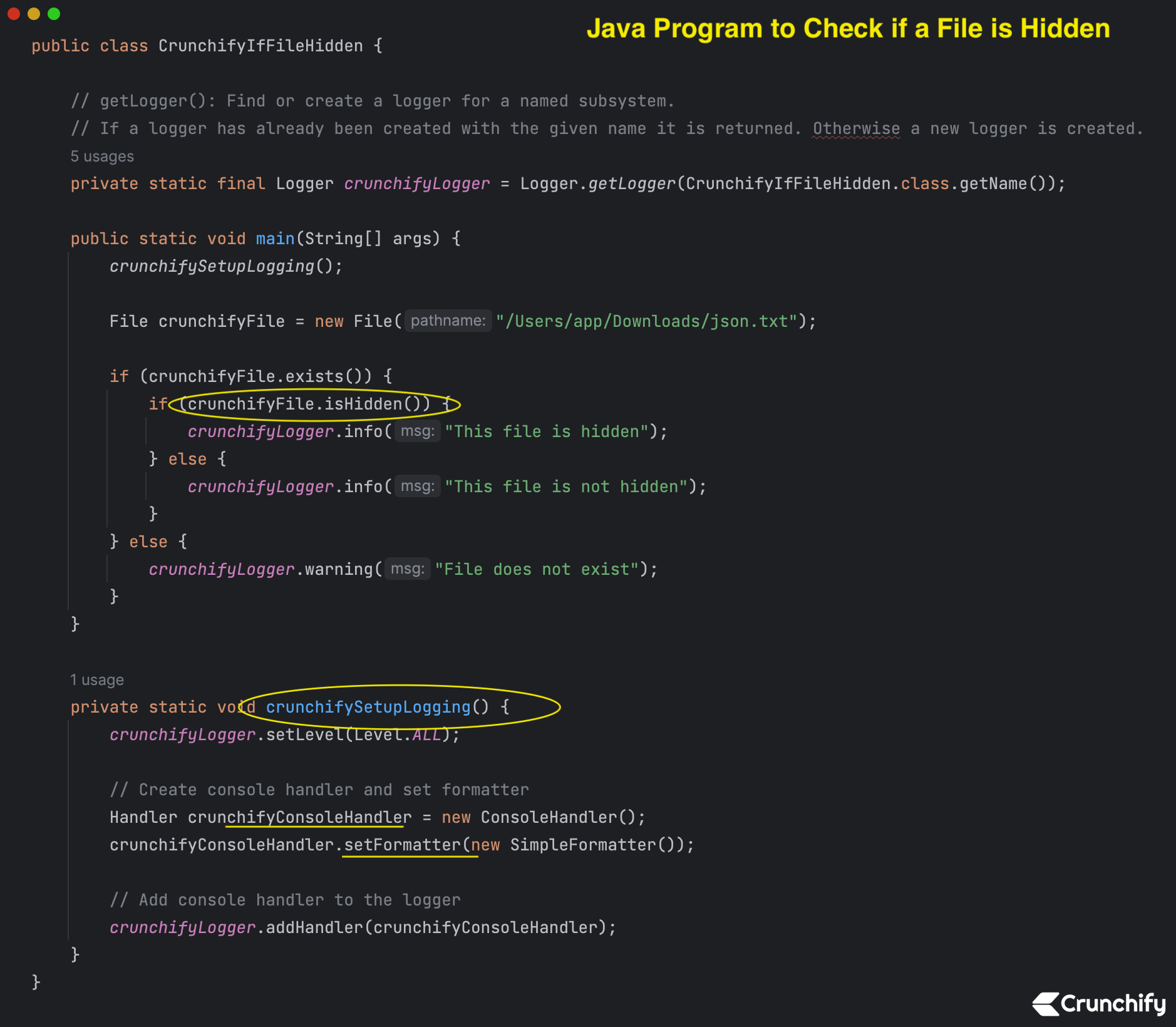
How to Check If A File is Hidden in Java?
File.isHidden()
Tests whether the file named by this abstract pathname is a hidden file. The exact definition of hidden is system-dependent. On UNIX systems, a file is considered to be hidden if its name begins with a period character ('.')
. On Microsoft Windows systems, a file is considered to be hidden if it has been marked as such in the filesystem.
Returns:
true if and only if the file denoted by this abstract pathname is hidden according to the conventions of the underlying platform.
Throws:
SecurityException – If a security manager exists and its SecurityManager.checkRead
(java.lang.String) method denies read access to the file.
Code Explanation:
Our code represents a Java program that checks whether a specified file is hidden or not. Let’s go through each part of the code:
- public static void main(String[] args) { … }: This is the entry point of the Java program. The
main
method is where the program starts executing. crunchifySetupLogging();
: This line calls a method namedcrunchifySetupLogging()
. This method is responsible for setting up logging configurations, which is a good practice for tracking program behavior and debugging.File crunchifyFile = new File("/Users/app/Downloads/json.txt");
: Here, aFile
object namedcrunchifyFile
is created, representing the file located at the specified path/Users/app/Downloads/json.txt
. You would replace this path with the actual path to the file you want to check.if (crunchifyFile.exists()) { ... } else { ... }
: This conditional statement checks if thecrunchifyFile
exists. If it does, the code inside the first block will execute; otherwise, the code inside theelse
block will execute.- Inside the first block of the conditional statement (
if (crunchifyFile.exists()) { ... }
), another conditional statement is used:if (crunchifyFile.isHidden()) { ... } else { ... }
. This inner conditional checks whether the file is hidden. If it is hidden, the code inside the first block of this inner conditional will execute; otherwise, the code inside theelse
block will execute. - Within each block of the inner conditional, the appropriate log message is generated using the
crunchifyLogger
instance. If the file is hidden, an info-level log message is logged usingcrunchifyLogger.info("This file is hidden");
. If the file is not hidden, a similar log message indicating that the file is not hidden is logged. - If the file does not exist (handled in the outer conditional’s
else
block), a warning-level log message is generated usingcrunchifyLogger.warning("File does not exist");
.
Complete code with logger
package crunchify.com.tutorials; import java.io.File; import java.util.logging.Logger; import java.util.logging.Level; import java.util.logging.ConsoleHandler; import java.util.logging.Handler; import java.util.logging.SimpleFormatter; /** * @author Crunchify * Java Program to Check if a File is Hidden * */ public class CrunchifyIfFileHidden { // getLogger(): Find or create a logger for a named subsystem. // If a logger has already been created with the given name it is returned. Otherwise a new logger is created. private static final Logger crunchifyLogger = Logger.getLogger(CrunchifyIfFileHidden.class.getName()); public static void main(String[] args) { crunchifySetupLogging(); File crunchifyFile = new File("/Users/app/Downloads/json.txt"); if (crunchifyFile.exists()) { if (crunchifyFile.isHidden()) { crunchifyLogger.info("This file is hidden"); } else { crunchifyLogger.info("This file is not hidden"); } } else { crunchifyLogger.warning("File does not exist"); } } private static void crunchifySetupLogging() { crunchifyLogger.setLevel(Level.ALL); // Create console handler and set formatter Handler crunchifyConsoleHandler = new ConsoleHandler(); crunchifyConsoleHandler.setFormatter(new SimpleFormatter()); // Add console handler to the logger crunchifyLogger.addHandler(crunchifyConsoleHandler); } }
Console Result Output.
Just run above program in Eclipse IDE or IntelliJ IDEA and you will see result as below.
Aug 18, 2023 2:13:28 PM crunchify.com.tutorials.CrunchifyIfFileHidden main INFO: This file is not hidden Aug 18, 2023 2:13:28 PM crunchify.com.tutorials.CrunchifyIfFileHidden main INFO: This file is not hidden
Here we learned how to use logging to track whether a specified file is hidden or not. It first sets up logging configurations, then checks if the file exists, and finally checks whether the file is hidden.
The post Java Program to Check if a File is Hidden appeared first on Crunchify.
0 Commentaires