Learn how to build a RESTful Java client using Jersey, making HTTP requests, handling JSON and XML responses. Step-by-step tutorial.
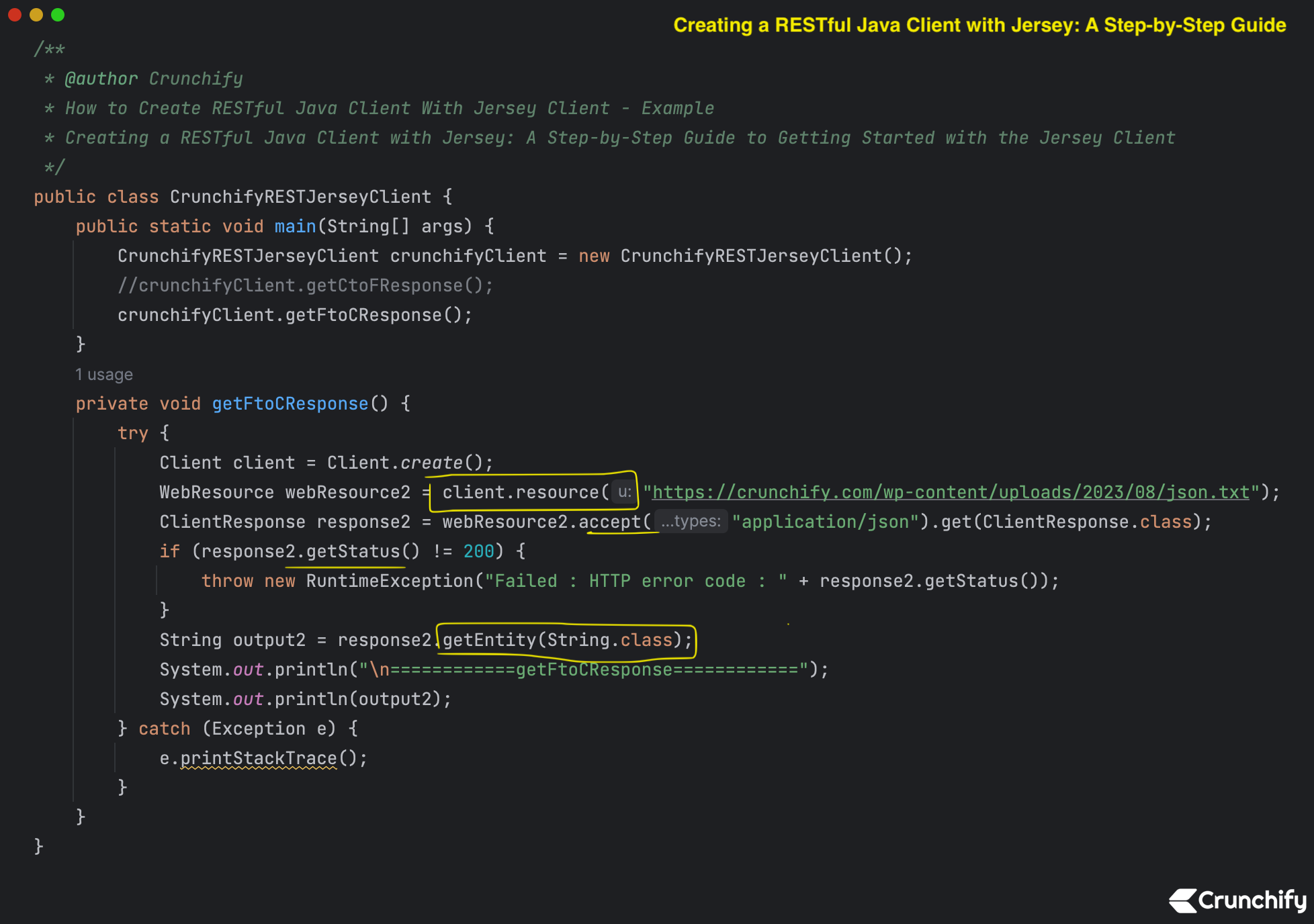
This tutorial show you how to use Jersey client APIs
to create a RESTful
Java client to perform “GET
” requests to REST service.
Maven Dependency
<dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-client</artifactId> <version>1.19.4</version> </dependency>
Detailed Explanation
- Package Declaration: Specifies the package name where the
CrunchifyRESTJerseyClient
class resides. - Imports: Import statements to bring in necessary classes from the Jersey Client library for working with HTTP requests.
- Class Definition: Defines the main class named
CrunchifyRESTJerseyClient
. - Main Method: Entry point of the program. Creates an instance of
CrunchifyRESTJerseyClient
and calls its methods. - getCtoFResponse Method: This method demonstrates making a GET request to a specific URL that returns XML data.
- Inside the method:
- Creates a Jersey
Client
instance. - Prepares a
WebResource
object representing the URL. - Sends a GET request and prints the requested URL.
- Checks if the response status code is not 200 (OK), throwing an exception if not.
- Reads the response content and prints it to the console.
- Creates a Jersey
- getFtoCResponse Method: Similar to
getCtoFResponse
, but demonstrates making a GET request for JSON data. - Inside the method:
- Creates a new
WebResource
object with a different URL and media type (JSON). - Sends a GET request and prints the requested URL.
- Checks if the response status code is not 200 (OK), throwing an exception if not.
- Reads the response content and prints it to the console.
- Creates a new
Complete Program:
package crunchify.com.tutorial; import com.sun.jersey.api.client.Client; import com.sun.jersey.api.client.ClientResponse; import com.sun.jersey.api.client.WebResource; /** * @author Crunchify * How to Create RESTful Java Client With Jersey Client - Example * Creating a RESTful Java Client with Jersey: A Step-by-Step Guide to Getting Started with the Jersey Client */ public class CrunchifyRESTJerseyClient { public static void main(String[] args) { CrunchifyRESTJerseyClient crunchifyClient = new CrunchifyRESTJerseyClient(); crunchifyClient.getCtoFResponse(); crunchifyClient.getFtoCResponse(); } private void getFtoCResponse() { try { Client client = Client.create(); WebResource webResource2 = client.resource("https://crunchify.com/wp-content/uploads/2023/08/json.txt"); ClientResponse response2 = webResource2.accept("application/json").get(ClientResponse.class); if (response2.getStatus() != 200) { throw new RuntimeException("Failed : HTTP error code : " + response2.getStatus()); } String output2 = response2.getEntity(String.class); System.out.println("\n============getFtoCResponse============"); System.out.println(output2); } catch (Exception e) { e.printStackTrace(); } } private void getCtoFResponse() { try { Client client = Client.create(); WebResource webResource = client.resource("https://crunchify.com/wp-content/uploads/2023/08/json.txt"); ClientResponse response = webResource.accept("application/xml").get(ClientResponse.class); if (response.getStatus() != 200) { throw new RuntimeException("Failed : HTTP error code : " + response.getStatus()); } String output = response.getEntity(String.class); System.out.println("============getCtoFResponse============"); System.out.println(output); } catch (Exception e) { e.printStackTrace(); } } }
Output:
============getCtoFResponse============ { "Name": "crunchify.com", "Author": "App Shah", "Company List": [ "Compnay: eBay", "Compnay: Paypal", "Compnay: Google" ] } ============getFtoCResponse============ { "Name": "crunchify.com", "Author": "App Shah", "Company List": [ "Compnay: eBay", "Compnay: Paypal", "Compnay: Google" ] } Process finished with exit code 0
This tutorial has walked you through the process of creating a powerful RESTful Java client using Jersey. With detailed explanations and practical examples, you’ve gained insights into making HTTP requests, handling various response formats, and implementing inline logging for debugging.
Armed with this knowledge, you’re now equipped to confidently interact with RESTful APIs and incorporate client-side functionality into your Java applications. Take these skills and explore the world of seamless API integration and efficient communication.
The post Creating a RESTful Java Client with Jersey: A Step-by-Step Guide to Getting Started with the Jersey Client appeared first on Crunchify.
0 Commentaires