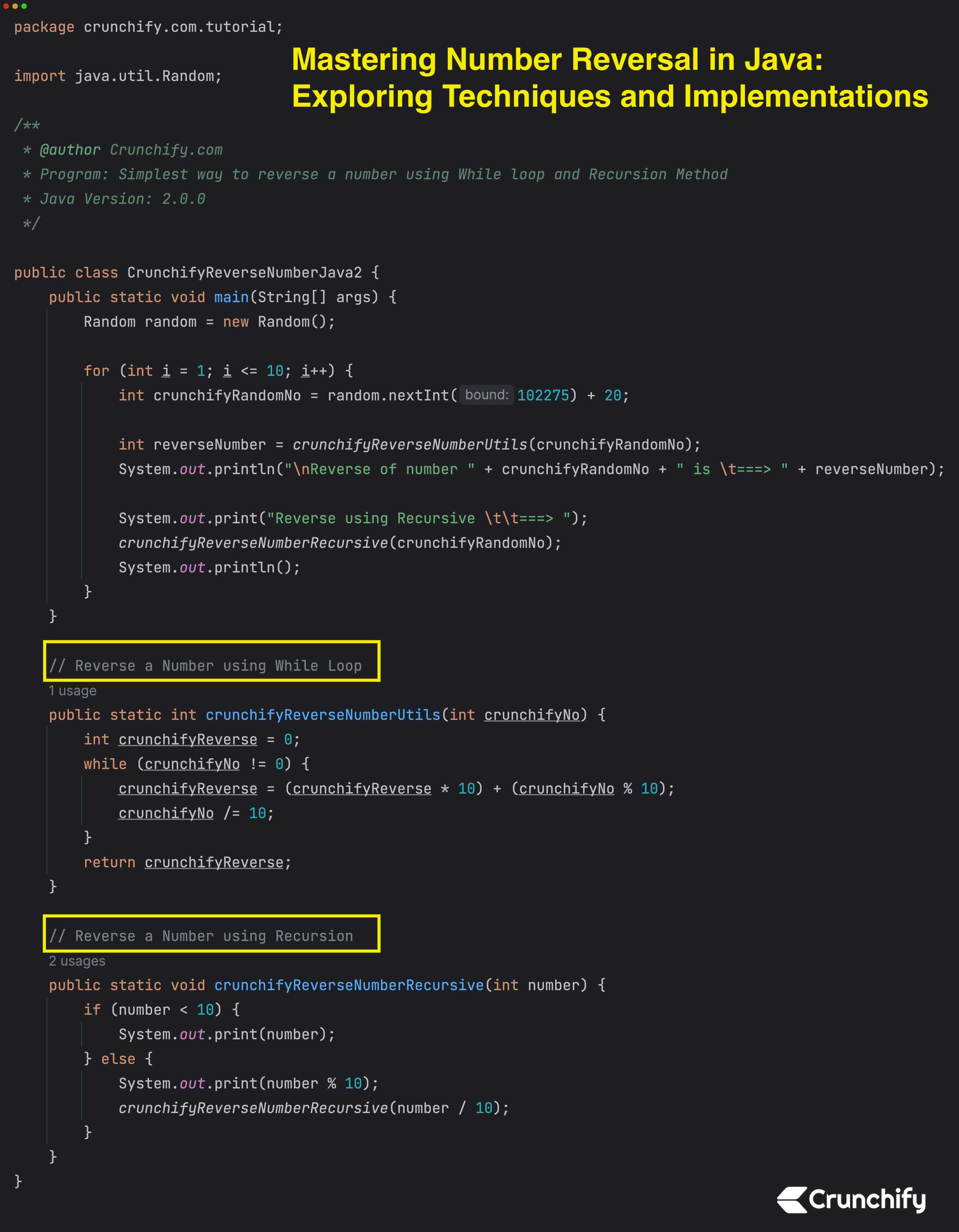
Explore a Java program showcasing two methods for reversing numbers: using a while loop and recursion. Learn how each technique works and see examples of reversing random numbers. A practical demonstration of fundamental programming concepts.
How to reverse a number in Java? How to reverse a number in Java using Recursion method? In this tutorial we will go over all details on how to reverse a number
using While Loop and Recursion Method.
Logic is very simple: In each iteration of while loop
, the remainder when crunchifyNo
is divided by 10 is calculated and the value of crunchifyNo is reduced by times. It is designed to reverse numbers using two different methods: a while loop and recursion. It generates random numbers between 4 and 102294, reverses each of them using both methods, and displays the results.
There is another tutorial on site about How to reserve a String in Java, if you are interested.
Here is a detailed explanation:
The key components of the provided Java program:
- Package and Class Declaration: The package statement (
package crunchify.com.tutorial;
) groups related classes together, aiding in organization. The classCrunchifyReverseNumberJava
serves as the main class for this program. - Main Method (
main
): Themain
method is where the program execution starts. In this program, it does the following:- Initializes variables to store the randomly generated number (
crunchifyRandomNo
) and its reversed form (reverseNumber
). - Enters a loop that generates random numbers and processes them.
- For each iteration of the loop (10 iterations in this case):
- Generates a random number within the range 4 to 102294 and assigns it to
crunchifyRandomNo
. - Calls the utility method
crunchifyReverseNumberUtils
to reversecrunchifyRandomNo
using a while loop, storing the result inreverseNumber
. - Prints the original and reversed numbers.
- Calls the recursive method
crunchifyReverseNumberRecursive
to reversecrunchifyRandomNo
using recursion.
- Generates a random number within the range 4 to 102294 and assigns it to
- Initializes variables to store the randomly generated number (
- Random Number Generation Loop: The loop generates random numbers for experimentation. The
Math.random()
function returns a random decimal number between 0 (inclusive) and 1 (exclusive). By scaling and shifting this value, the program generates random numbers in the desired range. println
andprint
Methods: These methods simplify the printing of text to the console.println
adds a newline character after printing, moving the cursor to the next line.print
outputs text without moving to the next line.crunchifyReverseNumberUtils
Method: This utility method reverses a number using a while loop. The process involves repeatedly extracting the last digit of the number (using the modulo%
operation) and constructing the reversed number by adding these digits to the right.crunchifyReverseNumberRecursive
Method: This method reverses a number using recursion. It employs the following steps:- Checks if the given number is less than 10. If true, it prints the number directly.
- If the number is not less than 10:
- Prints the last digit of the number (using the remainder
%
operation). - Recursively calls itself with the remaining portion of the number (excluding the last digit), effectively reversing the order.
- Prints the last digit of the number (using the remainder
CrunchifyReverseNumberJava.java
package crunchify.com.tutorial; /** * @author Crunchify.com * Program: Simplest way to reverse a number using While loop and Recursion Method * Java Version: 1.0.0 * */ public class CrunchifyReverseNumberJava { public static void main(String args[]) { int crunchifyRandomNo; int reverseNumber; for (int i = 1; i <= 10; i++) { crunchifyRandomNo = (4 + (int) (Math.random() * ((102295 - 20)))); reverseNumber = crunchifyReverseNumberUtils(crunchifyRandomNo); println("\nReverse of number " + crunchifyRandomNo + " is \t===> " + reverseNumber); print("Reverse using Recursive \t\t===> "); crunchifyReverseNumberRecursive(crunchifyRandomNo); } } private static void print(String string) { System.out.print(string); } private static void println(String result) { System.out.println(result); } // Reverse a Number using While Loop public static int crunchifyReverseNumberUtils(int crunchifyNo) { int crunchifyReverse = 0; while (crunchifyNo != 0) { crunchifyReverse = (crunchifyReverse * 10) + (crunchifyNo % 10); crunchifyNo = crunchifyNo / 10; } return crunchifyReverse; } // Reverse a Number using Recursion public static void crunchifyReverseNumberRecursive(int number) { if (number < 10) { println("" + number); return; } else { print(""+number % 10); // Method is calling itself: recursion crunchifyReverseNumberRecursive(number / 10); } } }
Just run above program as java application and you will see below result. As you notice in program, we are generating Random integer every time so your result might be different every time.
Eclipse console Output:
Reverse of number 38648 is ===> 84683 Reverse using Recursive ===> 84683 Reverse of number 50188 is ===> 88105 Reverse using Recursive ===> 88105 Reverse of number 87231 is ===> 13278 Reverse using Recursive ===> 13278 Reverse of number 37175 is ===> 57173 Reverse using Recursive ===> 57173 Reverse of number 70257 is ===> 75207 Reverse using Recursive ===> 75207 Reverse of number 60763 is ===> 36706 Reverse using Recursive ===> 36706 Reverse of number 76373 is ===> 37367 Reverse using Recursive ===> 37367 Reverse of number 26783 is ===> 38762 Reverse using Recursive ===> 38762 Reverse of number 99342 is ===> 24399 Reverse using Recursive ===> 24399 Reverse of number 4925 is ===> 5294 Reverse using Recursive ===> 5294
Hope you get an idea about logic and you face any issue in Java program to reverse number.
The post Mastering Number Reversal in Java: Exploring Techniques and Implementations appeared first on Crunchify.
0 Commentaires