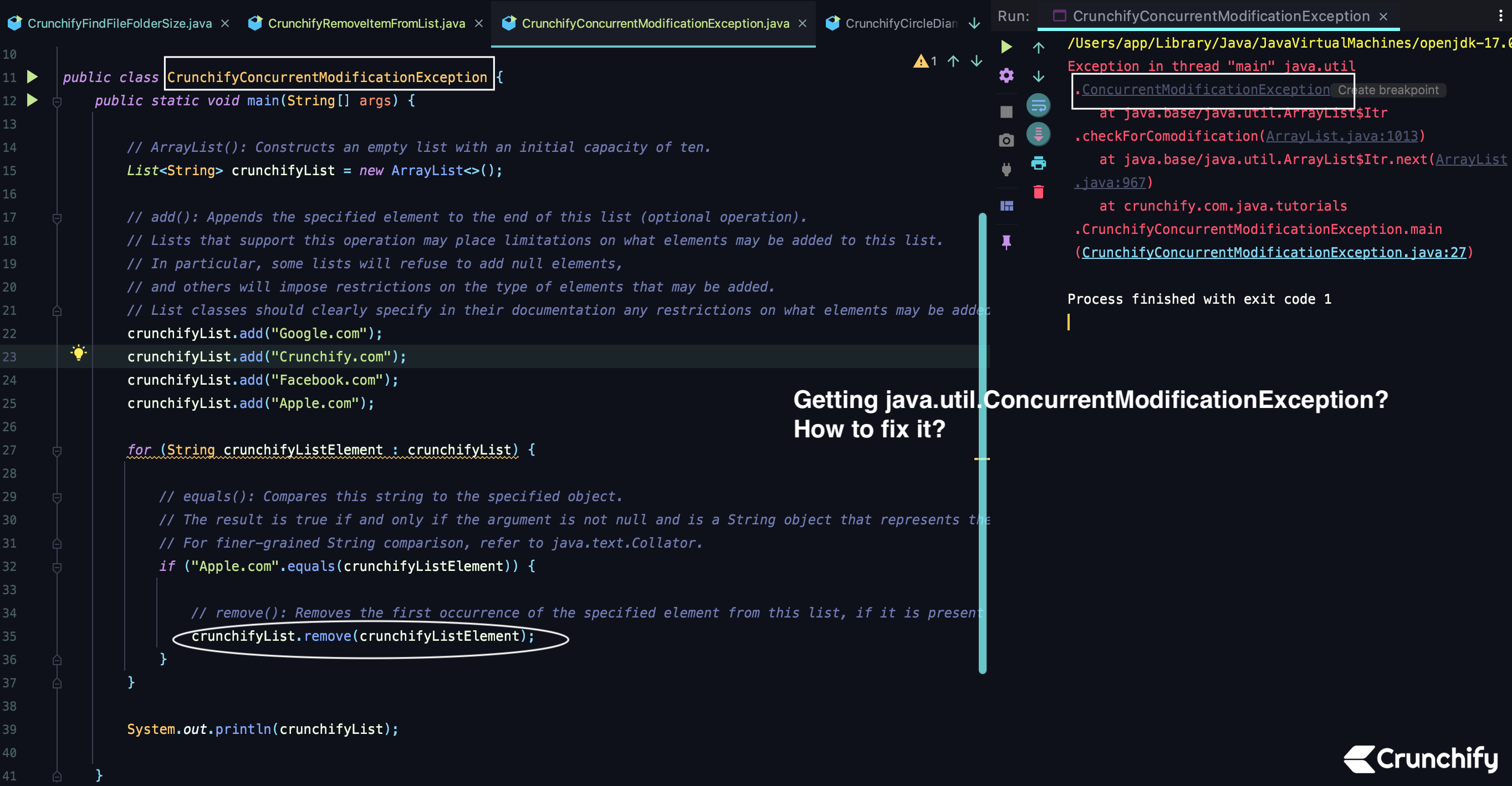
Are you getting ConcurrentModification Exception in Java? How to fix it? Mainly this happens when you try to remove element from ArrayList while iterating through it.
We usually get this exception in multithreading environment in production.
Concurrent Modification Exception: Understanding and Avoiding in a Multi-Threaded Environment
In a multi-threaded environment, where multiple threads are executing simultaneously, the ability to modify shared data structures is crucial. However, if the shared data structures are modified while they are being used by another thread, the result can be unpredictable behavior and the occurrence of an exception. One such exception is the Concurrent Modification Exception. In this post, we’ll discuss what the Concurrent Modification Exception is, why it occurs, and how to avoid it.
What is Concurrent Modification Exception?
Concurrent Modification Exception is a runtime exception that is thrown when a collection (such as a list, set, or map) is modified while it is being iterated over by another thread. The result of this exception can be unexpected behavior and even crashes. This exception occurs because the collection’s underlying data structure is being altered while it is being accessed.
Why does Concurrent Modification Exception occur?
In a multi-threaded environment, it is possible for multiple threads to access and modify a shared collection concurrently. This can lead to race conditions where two or more threads attempt to modify the collection at the same time. If one thread modifies the collection while another thread is iterating over it, the result is the Concurrent Modification Exception.
How to avoid Concurrent Modification Exception
There are several ways to avoid the Concurrent Modification Exception in a multi-threaded environment:
- Synchronization: The easiest way to avoid the Concurrent Modification Exception is to use synchronization mechanisms such as locks or synchronized collections. This ensures that only one thread at a time can access the collection, avoiding race conditions and the exception.
- Iterators with fail-fast behavior: Java provides several Iterators, such as Iterator and ListIterator, that have a fail-fast behavior. This means that they will throw the Concurrent Modification Exception if they detect that the collection has been modified while they are being used. By using these iterators, you can detect and avoid the exception.
- Copying the collection: Another way to avoid the Concurrent Modification Exception is to make a copy of the collection before iterating over it. This ensures that the original collection cannot be modified while it is being iterated over, avoiding the exception.
The Concurrent Modification Exception is a common issue in a multi-threaded environment and can be avoided by using proper synchronization mechanisms, using iterators with fail-fast behavior, or by copying the collection before iterating over it. Understanding and avoiding the Concurrent Modification Exception is crucial for developing stable and reliable multi-threaded applications.
Here is a complete Java Exception Stack trace.
/Users/app/Library/Java/JavaVirtualMachines/openjdk-17.0.1/Contents/Home/bin/java --enable-preview -javaagent:/Applications/IntelliJ IDEA.app crunchify.com.java.tutorials.CrunchifyConcurrentModificationException Exception in thread "main" java.util.ConcurrentModificationException at java.base/java.util.ArrayList$Itr.checkForComodification(ArrayList.java:1013) at java.base/java.util.ArrayList$Itr.next(ArrayList.java:967) at crunchify.com.java.tutorials.CrunchifyConcurrentModificationException.main(CrunchifyConcurrentModificationException.java:27) Process finished with exit code 1
Let’s first reproduce Concurrent Modification Exception.
CrunchifyConcurrentModificationException.java
package crunchify.com.java.tutorials; import java.util.ArrayList; import java.util.List; /** * @author Crunchify.com * What is ConcurrentModification Exception in Java? How to fix it? */ public class CrunchifyConcurrentModificationException { public static void main(String[] args) { // ArrayList(): Constructs an empty list with an initial capacity of ten. List<String> crunchifyList = new ArrayList<>(); // add(): Appends the specified element to the end of this list (optional operation). // Lists that support this operation may place limitations on what elements may be added to this list. // In particular, some lists will refuse to add null elements, // and others will impose restrictions on the type of elements that may be added. // List classes should clearly specify in their documentation any restrictions on what elements may be added. crunchifyList.add("Google.com"); crunchifyList.add("Crunchify.com"); crunchifyList.add("Facebook.com"); crunchifyList.add("Apple.com"); for (String crunchifyListElement : crunchifyList) { // equals(): Compares this string to the specified object. // The result is true if and only if the argument is not null and is a String object that represents the same sequence of characters as this object. // For finer-grained String comparison, refer to java.text.Collator. if ("Apple.com".equals(crunchifyListElement)) { // remove(): Removes the first occurrence of the specified element from this list, if it is present (optional operation). crunchifyList.remove(crunchifyListElement); } } System.out.println(crunchifyList); } }
Just run above Java Program as Java application and you will be able to generate error.
How to fix Concurrent Modification Exception?
Here is a complete example and 5 different ways to avoid
ConcurrentModification Exception.Let me know if you face any issue running above program.
The post Concurrent Modification Exception: Understanding and Avoiding in a Multi-Threaded Environment appeared first on Crunchify.
0 Commentaires