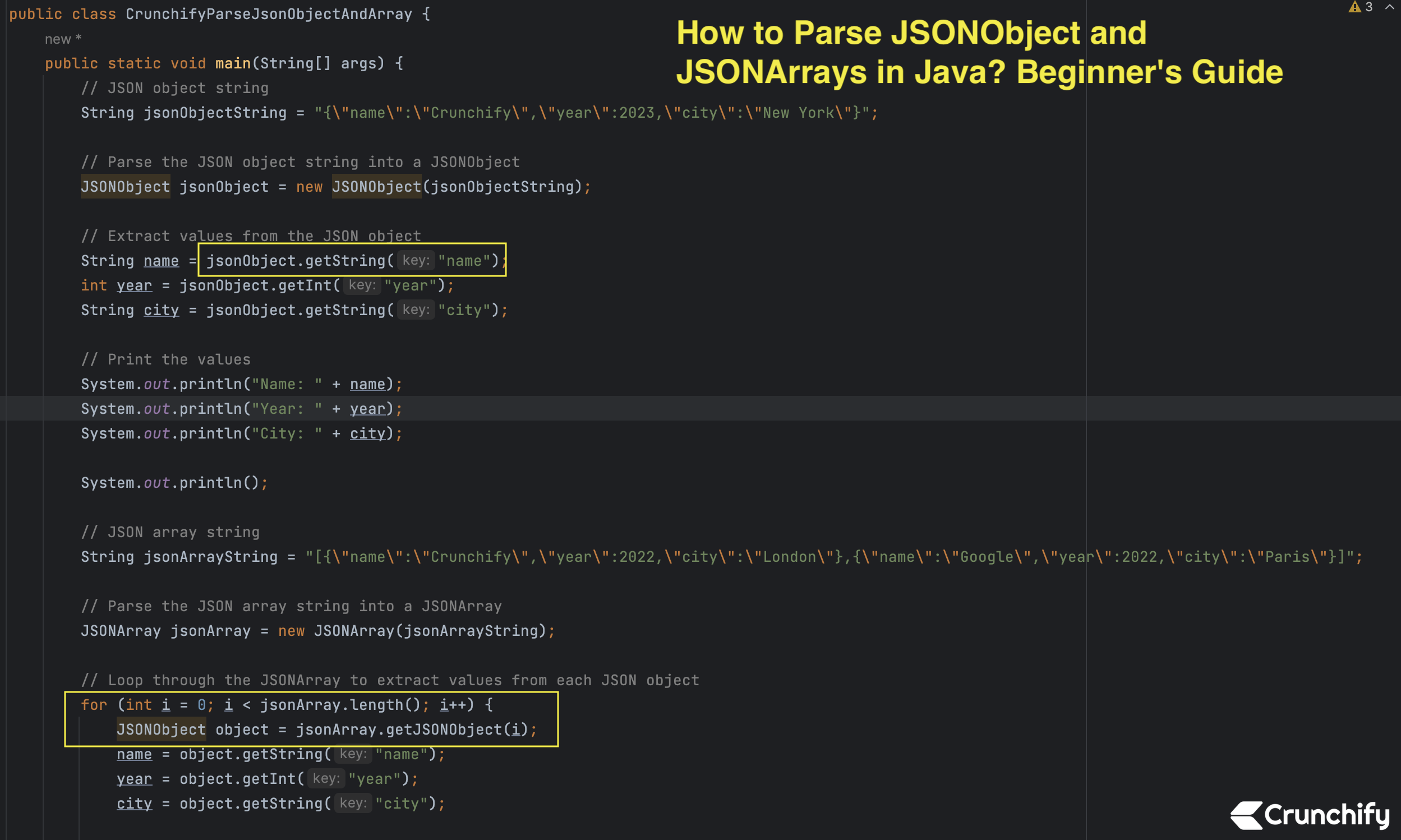
Here is a simple Java tutorial which demonstrate how to parse JSONObject and JSONArrays in Java.
JSON syntax is a subset of the JavaScript object notation syntax:
- Data is in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
Just incase if you want to take a look at simple JSON tutorial which I’ve written sometime back. In this example we will read JSON File Crunchify_JSON.txt
from file system and then we will iterate through it.
In order to run below Java project, please add below Maven Dependency to your project in pom.xml file.
<dependency> <groupId>org.json</groupId> <artifactId>json</artifactId> <version>20151123</version> </dependency>
Example-1.
Create this .txt file and update path in Java project:
{ "blogURL": "https://crunchify.com", "twitter": "https://twitter.com/Crunchify", "social": { "facebook": "http://facebook.com/Crunchify", "pinterest": "https://www.pinterest.com/Crunchify/crunchify-articles", "rss": "https://crunchify.com/feed/" } }
Java Program:
package com.crunchify.tutorials; import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import org.json.JSONException; import org.json.JSONObject; /** * @author Crunchify.com * */ public class CrunchifyParseJSONObject { public static void main(String[] args) throws FileNotFoundException, JSONException { String jsonData = ""; BufferedReader br = null; try { String line; br = new BufferedReader(new FileReader("/Users/<username>/Documents/Crunchify_JSON.txt")); while ((line = br.readLine()) != null) { jsonData += line + "\n"; } } catch (IOException e) { e.printStackTrace(); } finally { try { if (br != null) br.close(); } catch (IOException ex) { ex.printStackTrace(); } } // System.out.println("File Content: \n" + jsonData); JSONObject obj = new JSONObject(jsonData); System.out.println("blogURL: " + obj.getString("blogURL")); System.out.println("twitter: " + obj.getString("twitter")); System.out.println("social: " + obj.getJSONObject("social")); } }
Result:
blogURL: https://crunchify.com twitter: https://twitter.com/Crunchify social: {"facebook":"http://facebook.com/Crunchify","rss":"https://crunchify.com/feed/","pinterest":"https://www.pinterest.com/Crunchify/crunchify-articles"}
Example-2.
Here is a complete Java program that demonstrates parsing both JSON objects and arrays.
CrunchifyParseJsonObjectAndArray.java
package crunchify.com.java.tutorials; import org.json.JSONArray; import org.json.JSONObject; /** * @author Crunchify.com * Program: How to Parse JSONObject and JSONArrays in Java? Beginner's Guide * */ public class CrunchifyParseJsonObjectAndArray { public static void main(String[] args) { // JSON object string String jsonObjectString = "{\"name\":\"Crunchify\",\"year\":2023,\"city\":\"New York\"}"; // Parse the JSON object string into a JSONObject JSONObject jsonObject = new JSONObject(jsonObjectString); // Extract values from the JSON object String name = jsonObject.getString("name"); int year = jsonObject.getInt("year"); String city = jsonObject.getString("city"); // Print the values System.out.println("Name: " + name); System.out.println("Year: " + year); System.out.println("City: " + city); System.out.println(); // JSON array string String jsonArrayString = "[{\"name\":\"Crunchify\",\"year\":2022,\"city\":\"London\"},{\"name\":\"Google\",\"year\":2022,\"city\":\"Paris\"}]"; // Parse the JSON array string into a JSONArray JSONArray jsonArray = new JSONArray(jsonArrayString); // Loop through the JSONArray to extract values from each JSON object for (int i = 0; i < jsonArray.length(); i++) { JSONObject object = jsonArray.getJSONObject(i); name = object.getString("name"); year = object.getInt("year"); city = object.getString("city"); System.out.println("Name: " + name); System.out.println("Year: " + year); System.out.println("City: " + city); } } }
IntelliJ IDEA Result
Just run above program as a Java Application and you should see result as below.
Name: Crunchify Year: 2023 City: New York Name: Crunchify Year: 2022 City: London Name: Google Year: 2022 City: Paris Process finished with exit code 0
Details:
- The first step is to import the
org.json.JSONObject
andorg.json.JSONArray
classes, which are part of the org.json library. - Then, we define a
jsonObjectString
variable, which holds a string representation of a JSON object. - Using the
JSONObject
class, we parse thejsonObjectString
into aJSONObject
using thenew JSONObject(jsonObjectString)
constructor. - Then, we use the
getString
,getInt
, andgetBoolean
methods to extract values from thejsonObject
. - After printing out the values, we do a similar process to parse a
jsonArrayString
into aJSONArray
and loop through each JSON object to extract and print values.
The post How to Parse JSONObject and JSONArrays in Java? Beginner’s Guide appeared first on Crunchify.
0 Commentaires