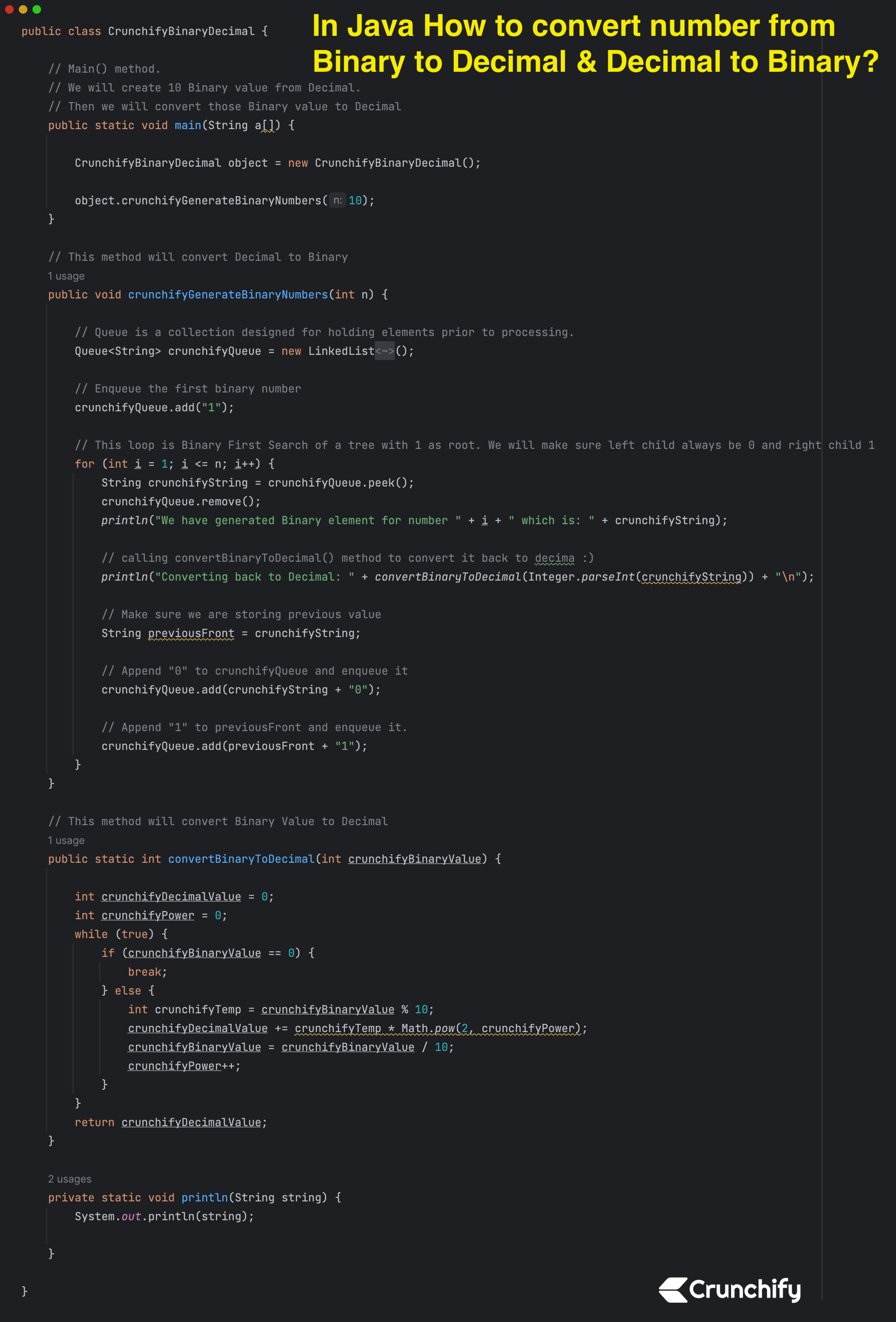
In Java How to convert from Binary to Decimal? At the same time, How to convert from Decimal to Binary? Well, this is another most common interview questions you may hear during interview process.
In this tutorial we will go over steps on how to convert number from Binary to Decimal and vice versa.
What is Binary number?
Have you seen movie Matrix? Well, you must have remembered a screen with all numbers filled with 0 and 1
. Well, Binary number is a representation of decimal number in the form of 0 and 1.
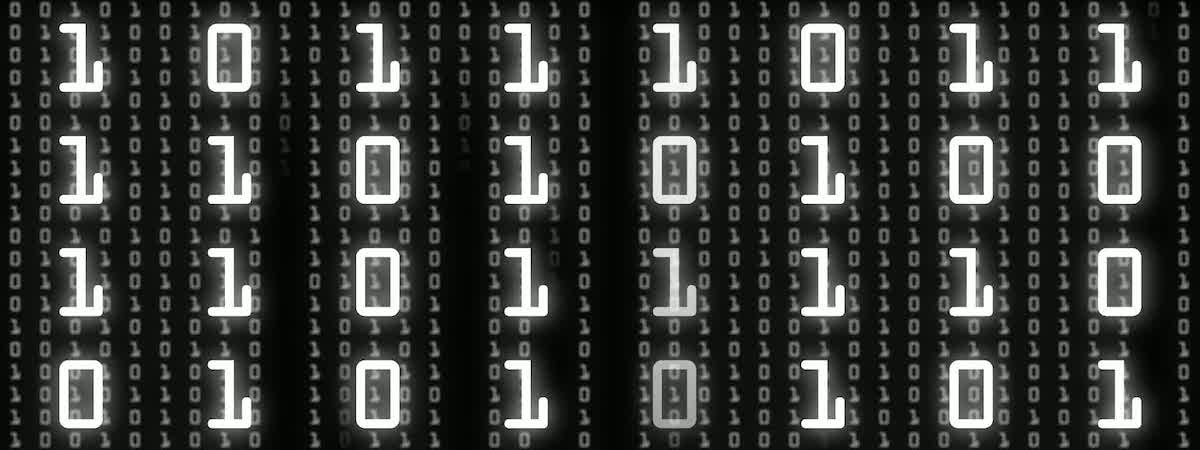
What is Decimal number?
Decimal number is also called number in base 10
. Also, we used it in our everyday life. 0,1,2,3,4,5,6,7,8,9 are decimal number
and all other numbers are based on these 10 numbers.
Let’s write Java program to convert number from
- Binary to Decimal
- Decimal to Binary
Detailed logic:
This Java code is a program that demonstrates how to convert numbers between binary and decimal representations using queues. Let’s break down the code step by step:
- The code is organized into a Java package named
crunchify.com.tutorial
. - It imports the necessary classes from the
java.util
package:LinkedList
andQueue
. - The main class is named
CrunchifyBinaryDecimal
. - Inside the
CrunchifyBinaryDecimal
class, there is amain
method which serves as the entry point of the program. It creates an instance of theCrunchifyBinaryDecimal
class and calls thecrunchifyGenerateBinaryNumbers
method to generate binary numbers and convert them back to decimal. - The
crunchifyGenerateBinaryNumbers
method takes an integern
as a parameter and generates binary numbers from 1 ton
. - The method uses a queue (
crunchifyQueue
) to generate binary numbers iteratively. The queue starts with the binary number “1”. - The loop iterates from 1 to
n
, generating binary numbers and converting them back to decimal. For each iteration:- The front element of the queue is removed and processed.
- The method
convertBinaryToDecimal
is called to convert the binary number to its decimal equivalent. - The previous front value is stored.
- “0” is appended to the current binary string and enqueued.
- “1” is appended to the previous front value and enqueued.
- The
convertBinaryToDecimal
method converts a binary value to its decimal equivalent using the process of successive division by 10 and binary-to-decimal conversion formula. - The
println
method is a private utility method used to print output to the console.
package crunchify.com.tutorial; import java.util.LinkedList; import java.util.Queue; /** * @author Crunchify.com * Program: Java program to convert number from Binary to Decimal and Decimal to Binary * Version: 1.0.0 */ public class CrunchifyBinaryDecimal { // Main() method. // We will create 10 Binary value from Decimal. // Then we will convert those Binary value to Decimal public static void main(String a[]) { CrunchifyBinaryDecimal object = new CrunchifyBinaryDecimal(); object.crunchifyGenerateBinaryNumbers(10); } // This method will convert Decimal to Binary public void crunchifyGenerateBinaryNumbers(int n) { // Queue is a collection designed for holding elements prior to processing. Queue<String> crunchifyQueue = new LinkedList<String>(); // Enqueue the first binary number crunchifyQueue.add("1"); // This loop is Binary First Search of a tree with 1 as root. We will make sure left child always be 0 and right child 1 for (int i = 1; i <= n; i++) { String crunchifyString = crunchifyQueue.peek(); crunchifyQueue.remove(); println("We have generated Binary element for number " + i + " which is: " + crunchifyString); // calling convertBinaryToDecimal() method to convert it back to decima :) println("Converting back to Decimal: " + convertBinaryToDecimal(Integer.parseInt(crunchifyString)) + "\n"); // Make sure we are storing previous value String previousFront = crunchifyString; // Append "0" to crunchifyQueue and enqueue it crunchifyQueue.add(crunchifyString + "0"); // Append "1" to previousFront and enqueue it. crunchifyQueue.add(previousFront + "1"); } } // This method will convert Binary Value to Decimal public static int convertBinaryToDecimal(int crunchifyBinaryValue) { int crunchifyDecimalValue = 0; int crunchifyPower = 0; while (true) { if (crunchifyBinaryValue == 0) { break; } else { int crunchifyTemp = crunchifyBinaryValue % 10; crunchifyDecimalValue += crunchifyTemp * Math.pow(2, crunchifyPower); crunchifyBinaryValue = crunchifyBinaryValue / 10; crunchifyPower++; } } return crunchifyDecimalValue; } private static void println(String string) { System.out.println(string); } }
Here is a Eclipse console result
As you see below in Eclipse console result, we have first converted Decimal to Binary and then Binary to Decimal.
We have generated Binary element for number 1 which is: 1 Converting back to Decimal: 1 We have generated Binary element for number 2 which is: 10 Converting back to Decimal: 2 We have generated Binary element for number 3 which is: 11 Converting back to Decimal: 3 We have generated Binary element for number 4 which is: 100 Converting back to Decimal: 4 We have generated Binary element for number 5 which is: 101 Converting back to Decimal: 5 We have generated Binary element for number 6 which is: 110 Converting back to Decimal: 6 We have generated Binary element for number 7 which is: 111 Converting back to Decimal: 7 We have generated Binary element for number 8 which is: 1000 Converting back to Decimal: 8 We have generated Binary element for number 9 which is: 1001 Converting back to Decimal: 9 We have generated Binary element for number 10 which is: 1010 Converting back to Decimal: 10
More details if required:
Here is what we did:
- Create java class CrunchifyBinaryDecimal.java.
- Create 3 different crunchifyGenerateBinaryNumbers() and convertBinaryToDecimal(). Name explains the purpose of both methods.
- From main() – we will call method
crunchifyGenerateBinaryNumbers
() to start process.- This method will start for loop with number 10
- We will convert first 10 decimal numbers to Binary
- Immediately after that program will call
convertBinaryToDecimal
() to convert number back from Binary to Decimal
- We will print result on Eclipse console during this process.
Hope you get complete idea on how to convert numbers from Binary to Decimal and Decimal to Binary. Let me know if you have any questions.
The post Binary to Decimal Conversion and Vice Versa: Exploring Number Representations with Java Code appeared first on Crunchify.
0 Commentaires