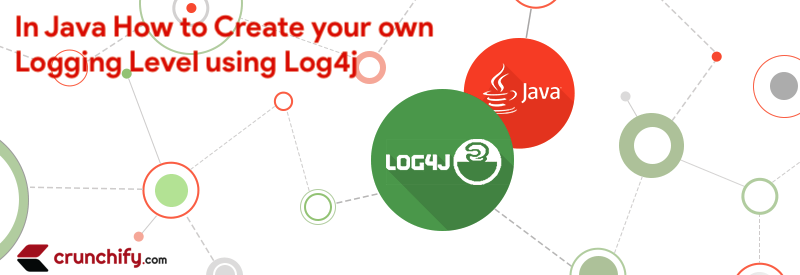
If you need to add your own logging level in Log4j, then you can do it as follows. You will have to create your own class which will extend from Level
, Custom Log Levels
with Apache Log4j 2.
Log4j
is a simple and flexible logging framework
. Logging equips the developer with detailed context for application failures. With log4j it is possible to enable logging at runtime without modifying the application binary.
The log4j package is designed so that these statements can remain in shipped code without incurring a heavy performance cost.
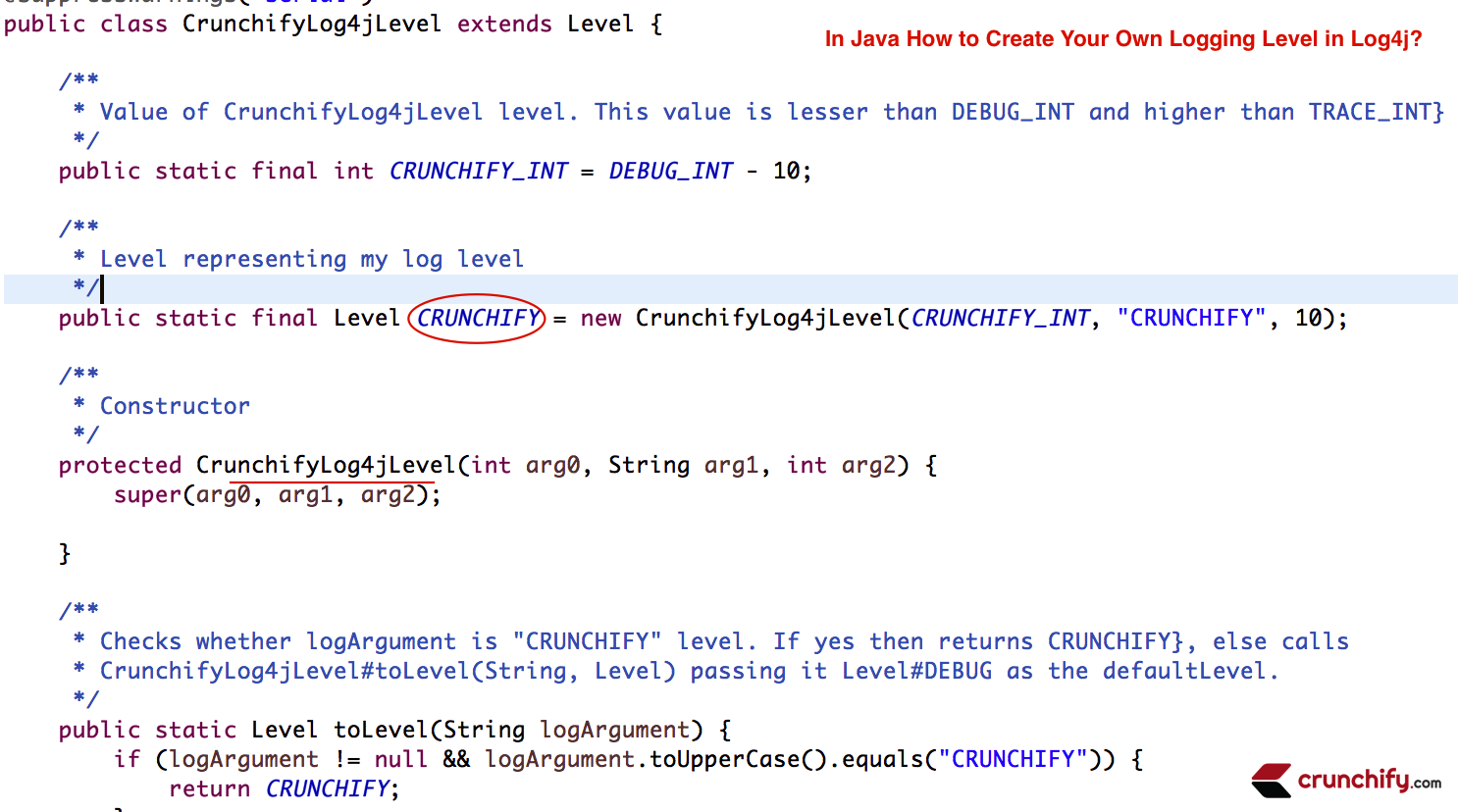
Log4j allows logging requests to print to multiple destinations. In log4j speak, an output destination is called an appender
. They vary from console, files, GUI components, remote socket servers to JMS.
Jar File you need.
Here is a Maven Dependency:
<dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>2.16.0</version> </dependency>
Update Log4j to latest version
CVE-2021-44228: Apache Log4j2 <=2.14.1 JNDI features used in configuration, log messages, and parameters do not protect against attacker controlled LDAP and other JNDI related endpoints.
From log4j 2.16.0, this behavior has been disabled by default.
You have to put log4j.xml
file under /resources
folder:
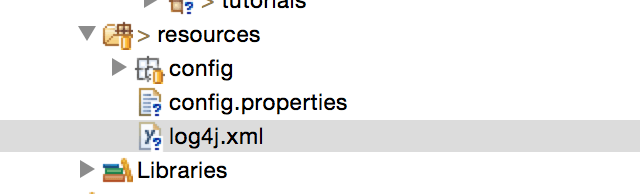
Here’s a sample Java Code for:
- Log4j Logging for custom Log levels in Java
- Creating your own logging level in log4j
- log4j custom logger example
CrunchifyLog4jLevel.java
package com.crunchify.tutorials; import org.apache.log4j.Level; /** * @author Crunchify.com * */ @SuppressWarnings("serial") public class CrunchifyLog4jLevel extends Level { /** * Value of CrunchifyLog4jLevel level. This value is lesser than DEBUG_INT and higher * than TRACE_INT} */ public static final int CRUNCHIFY_INT = DEBUG_INT - 10; /** * Level representing my log level */ public static final Level CRUNCHIFY = new CrunchifyLog4jLevel(CRUNCHIFY_INT, "CRUNCHIFY", 10); /** * Constructor */ protected CrunchifyLog4jLevel(int arg0, String arg1, int arg2) { super(arg0, arg1, arg2); } /** * Checks whether logArgument is "CRUNCHIFY" level. If yes then returns * CRUNCHIFY}, else calls CrunchifyLog4jLevel#toLevel(String, Level) passing * it Level#DEBUG as the defaultLevel. */ public static Level toLevel(String logArgument) { if (logArgument != null && logArgument.toUpperCase().equals("CRUNCHIFY")) { return CRUNCHIFY; } return (Level) toLevel(logArgument, Level.DEBUG); } /** * Checks whether val is CrunchifyLog4jLevel#CRUNCHIFY_INT. If yes then * returns CrunchifyLog4jLevel#CRUNCHIFY, else calls * CrunchifyLog4jLevel#toLevel(int, Level) passing it Level#DEBUG as the * defaultLevel * */ public static Level toLevel(int val) { if (val == CRUNCHIFY_INT) { return CRUNCHIFY; } return (Level) toLevel(val, Level.DEBUG); } /** * Checks whether val is CrunchifyLog4jLevel#CRUNCHIFY_INT. If yes * then returns CrunchifyLog4jLevel#CRUNCHIFY, else calls Level#toLevel(int, org.apache.log4j.Level) * */ public static Level toLevel(int val, Level defaultLevel) { if (val == CRUNCHIFY_INT) { return CRUNCHIFY; } return Level.toLevel(val, defaultLevel); } /** * Checks whether logArgument is "CRUNCHIFY" level. If yes then returns * CrunchifyLog4jLevel#CRUNCHIFY, else calls * Level#toLevel(java.lang.String, org.apache.log4j.Level) * */ public static Level toLevel(String logArgument, Level defaultLevel) { if (logArgument != null && logArgument.toUpperCase().equals("CRUNCHIFY")) { return CRUNCHIFY; } return Level.toLevel(logArgument, defaultLevel); } }
Another must read:
- How to Start Stop Apache Tomcat Server via Command Line? (Setup as Windows Service)
- Create and Deploy simple Web Service and Web Service Client in Eclipse
Here is a content of log4j.xml File
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE log4j:configuration SYSTEM "log4j.dtd"> <log4j:configuration xmlns:log4j="http://jakarta.apache.org/log4j/" debug="false"> <!-- FILE Appender --> <appender name="FILE" class="org.apache.log4j.FileAppender"> <param name="File" value="c:/crunchify.log" /> <param name="Append" value="false" /> <layout class="org.apache.log4j.PatternLayout"> <param name="ConversionPattern" value="%t %-5p %c - %m%n" /> </layout> </appender> <!-- CONSOLE Appender --> <appender name="CONSOLE" class="org.apache.log4j.ConsoleAppender"> <layout class="org.apache.log4j.PatternLayout"> <param name="ConversionPattern" value="%d{ISO8601} %-5p [%c{1}] %m%n" /> </layout> </appender> <!-- Limit Category and Specify Priority --> <category name="com.crunchify"> <priority value="CRUNCHIFY" class="com.crunchify.tutorials.CrunchifyLog4jLevel" /> <appender-ref ref="CONSOLE" /> </category> <!-- Setup the Root category --> <root> <appender-ref ref="CONSOLE" /> </root> </log4j:configuration>
CrunchifyLog4jLevelTest.java
package com.crunchify.tutorials; import org.apache.log4j.Level; import org.apache.log4j.Logger; import com.crunchify.tutorials.CrunchifyLog4jLevel; /** * Tests whether the new log level * com.crunchify.tutorials.CrunchifyLog4jLevel#CRUNCHIFY} is working * * @author Crunchify.com * */ public class CrunchifyLog4jLevelTest { public static void main(String[] args) { Logger logger = Logger.getLogger(CrunchifyLog4jLevelTest.class); logger.log(CrunchifyLog4jLevel.CRUNCHIFY, "I am CrunchifyLog4jLevelTest log"); logger.log(Level.DEBUG, "I am a DEBUG message"); } }
Run the test program and you should see below type of result in your Eclipse's Console
.
2013-08-01 15:22:36,758 CRUNCHIFY [CrunchifyLog4jLevelTest] I am CrunchifyLog4jLevelTest log 2013-08-01 15:22:36,758 DEBUG [CrunchifyLog4jLevelTest] I am a DEBUG message
The int value that you specify for your log level is important. Here I’ve defined “CRUNCHIFY
” log level is to be higher than the DEBUG
level but lower than the TRACE
level provided by log4j.
So whenever you have set a priority level to DEBUG on the category(in your log4j.xml
file), the CRUNCHIFY
level logs will NOT make it to the log file.
The post In Java How to Create your own Logging Level using Log4j (Configuring Log4j 2) appeared first on Crunchify.
0 Commentaires